mirror of
https://github.com/alacritty/alacritty.git
synced 2024-11-11 13:51:01 -05:00
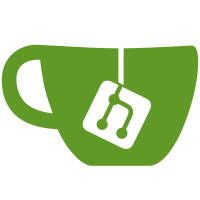
This commit adds support for a visual bell. Although the Handler in src/ansi.rs warns "Hopefully this is never implemented", I wanted to give it a try. A new config option is added, `visual_bell`, which sets the `duration` and `animation` function of the visual bell. The default `duration` is 150 ms, and the default `animation` is `EaseOutExpo`. To disable the visual bell, set its duration to 0. The visual bell is modeled by VisualBell in src/term/mod.rs. It has a method to ring the bell, `ring`, and another method, `intensity`. Both return the "intensity" of the bell, which ramps down from 1.0 to 0.0 at a rate set by `duration` and `animation`. Whether or not the Processor waits for events is now configurable in order to allow for smooth drawing of the visual bell.
35 lines
1.1 KiB
GLSL
35 lines
1.1 KiB
GLSL
// Copyright 2016 Joe Wilm, The Alacritty Project Contributors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
#version 330 core
|
|
in vec2 TexCoords;
|
|
in vec3 fg;
|
|
in vec3 bg;
|
|
flat in float vb;
|
|
flat in int background;
|
|
|
|
layout(location = 0, index = 0) out vec4 color;
|
|
layout(location = 0, index = 1) out vec4 alphaMask;
|
|
|
|
uniform sampler2D mask;
|
|
|
|
void main()
|
|
{
|
|
if (background != 0) {
|
|
alphaMask = vec4(1.0, 1.0, 1.0, 1.0);
|
|
color = vec4(bg + vb, 1.0);
|
|
} else {
|
|
alphaMask = vec4(texture(mask, TexCoords).rgb, 1.0);
|
|
color = vec4(fg, 1.0);
|
|
}
|
|
}
|