mirror of
https://github.com/yshui/picom.git
synced 2024-11-11 13:51:02 -05:00
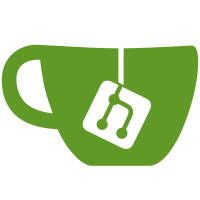
Previously we were using glibc's strtod function to parse floating point numbers. The problem is, strtod is locale dependent. Meaning 7,7 might be parsed as two numbers (7 and 7) in one locale, and parsed as one number (7 point 7) in another locale. This is undesirable. We need to set the locale to a value we know to make number parsing consistently. We could use setlocale(), but that is not thread-safe. We can also use uselocale(), which is thread-safe, but doesn't cover strtod (Yeah, some of the locale-aware functions only acknowledge the global locale, not the thread local one). So in frustration, I just wrote a simple floating point number parser myself. This parser obviously doesn't cover all cases strtod covers, but is good enough for our needs. Signed-off-by: Yuxuan Shui <yshuiv7@gmail.com>
57 lines
1.1 KiB
C
57 lines
1.1 KiB
C
// SPDX-License-Identifier: MPL-2.0
|
|
// Copyright (c) Yuxuan Shui <yshuiv7@gmail.com>
|
|
#pragma once
|
|
#include <ctype.h>
|
|
#include <stddef.h>
|
|
|
|
#define mstrncmp(s1, s2) strncmp((s1), (s2), strlen(s1))
|
|
|
|
char *mstrjoin(const char *src1, const char *src2);
|
|
char *
|
|
mstrjoin3(const char *src1, const char *src2, const char *src3);
|
|
void mstrextend(char **psrc1, const char *src2);
|
|
|
|
/// Parse a floating point number of form (+|-)?[0-9]*(\.[0-9]*)
|
|
double strtod_simple(const char *, const char **);
|
|
|
|
static inline int uitostr(unsigned int n, char *buf) {
|
|
int ret = 0;
|
|
unsigned int tmp = n;
|
|
while (tmp > 0) {
|
|
tmp /= 10;
|
|
ret++;
|
|
}
|
|
|
|
if (ret == 0)
|
|
ret = 1;
|
|
|
|
int pos = ret;
|
|
while (pos--) {
|
|
buf[pos] = n%10 + '0';
|
|
n /= 10;
|
|
}
|
|
return ret;
|
|
}
|
|
|
|
static inline const char *
|
|
skip_space_const(const char *src) {
|
|
if (!src)
|
|
return NULL;
|
|
while (*src && isspace(*src))
|
|
src++;
|
|
return src;
|
|
}
|
|
|
|
static inline char *
|
|
skip_space_mut(char *src) {
|
|
if (!src)
|
|
return NULL;
|
|
while (*src && isspace(*src))
|
|
src++;
|
|
return src;
|
|
}
|
|
|
|
#define skip_space(x) _Generic((x), \
|
|
char *: skip_space_mut, \
|
|
const char *: skip_space_const \
|
|
)(x)
|