mirror of
https://github.com/yshui/picom.git
synced 2024-10-27 05:24:17 -04:00
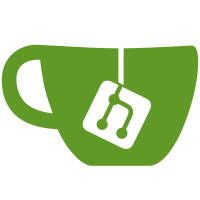
- Add D-Bus support. Currently 7 methods are available: "reset" (same as SIGUSR1), "list_win" (list the windows compton manages), "win_get" (get a property of the window), "win_set" (set a property of the window), "find_win" (find window based on client window / focus), "opts_get" (get the value of a compton option), and "opts_set" (set the value of a compton option), together with 4 signals: "win_added", "win_destroyed", "win_mapped", "win_unmapped". - D-Bus support depends on libdbus. - As there are many items and my time is tight, no much tests are done. Bugs to be expected. - Create a new header file `common.h` that contains shared content. - Fix some bugs in timeout handling. - Update file headers in all source files. - Re-enable --unredir-if-possible on multi-screen set-ups, as the user could turn if off manually anyway. - Check if the window is mapped in `repair_win()`. - Add ps->track_atom_lst and its handlers, to prepare for the new condition format. - Known issue 1: "win_get", "win_set", "opts_get", "opts_set" support a very limited number of targets only. New ones will be added gradually. - Known issue 2: Accidental drop of D-Bus connection is not handled. - Known issue 3: Introspection does not reveal all available methods, because some methods have unpredictable prototypes. Still hesitating about what to do... - Known issue 4: Error handling is not finished yet. Compton does not always reply with the correct error message (but it does print out the correct error message, usually).
45 lines
1.5 KiB
Bash
Executable file
45 lines
1.5 KiB
Bash
Executable file
#!/bin/sh
|
|
|
|
# === Get connection parameters ===
|
|
|
|
dpy=$(echo -n "$DISPLAY" | tr -c '[:alnum:]' _)
|
|
|
|
if [ -z "$dpy" ]; then
|
|
echo "Cannot find display."
|
|
exit 1
|
|
fi
|
|
|
|
service="com.github.chjj.compton.${dpy}"
|
|
interface='com.github.chjj.compton'
|
|
object='/com/github/chjj/compton'
|
|
type_win='uint32'
|
|
type_enum='uint16'
|
|
|
|
# === DBus methods ===
|
|
|
|
# List all window ID compton manages (except destroyed ones)
|
|
dbus-send --print-reply --dest="$service" "$object" "${interface}.list_win"
|
|
|
|
# Get window ID of currently focused window
|
|
focused=$(dbus-send --print-reply --dest="$service" "$object" "${interface}.find_win" string:focused | sed -n 's/^[[:space:]]*'${type_win}'\s*\([[:digit:]]*\).*/\1/p')
|
|
|
|
if [ -n "$focused" ]; then
|
|
# Get invert_color_force property of the window
|
|
dbus-send --print-reply --dest="$service" "$object" "${interface}.win_get" "${type_win}:${focused}" string:invert_color_force
|
|
|
|
# Set the window to have inverted color
|
|
dbus-send --print-reply --dest="$service" "$object" "${interface}.win_set" "${type_win}:${focused}" string:invert_color_force "${type_enum}:1"
|
|
else
|
|
echo "Cannot find focused window."
|
|
fi
|
|
|
|
# Set the clear_shadow setting to true
|
|
dbus-send --print-reply --dest="$service" "$object" "${interface}.opts_set" string:clear_shadow boolean:true
|
|
|
|
# Get the clear_shadow setting
|
|
dbus-send --print-reply --dest="$service" "$object" "${interface}.opts_get" string:clear_shadow
|
|
|
|
# Reset compton
|
|
sleep 3
|
|
dbus-send --print-reply --dest="$service" "$object" "${interface}.reset"
|
|
|