mirror of
https://github.com/polybar/polybar.git
synced 2024-11-11 13:50:56 -05:00
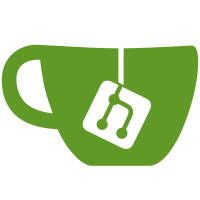
* Handle relative includes We change to the directory of the given config file before parsing. This allows us to handle relative includes. TODO: Maybe improve the name of the change_dir() function. * Fix unused result warning * Add `relative_to` parameter to expand() If the path is relative, we resolve it by prepending dirname(config) to the path. Add dirname() - Returns the parent directory of the file or an empty string. * Resolve relative paths Handle paths relative to the current file being parsed. * Remove unneeded change_dir() * Fix expand() `is_absolute` is calculated after we expand the path. `relative_to` must be a directory. Add test for expand() with relative paths * Recalculate `is_absolute` after expanding `path` * Add more file_util::expand tests * Add changelog Co-authored-by: patrick96 <p.ziegler96@gmail.com>
46 lines
1.5 KiB
C++
46 lines
1.5 KiB
C++
#include "utils/file.hpp"
|
|
|
|
#include <iomanip>
|
|
#include <iostream>
|
|
|
|
#include "common/test.hpp"
|
|
#include "utils/command.hpp"
|
|
#include "utils/env.hpp"
|
|
|
|
using namespace polybar;
|
|
|
|
using expand_test_t = pair<string, string>;
|
|
class ExpandTest : public testing::TestWithParam<expand_test_t> {};
|
|
|
|
vector<expand_test_t> expand_absolute_test_list = {
|
|
{"~/foo", env_util::get("HOME") + "/foo"},
|
|
{"$HOME/foo", env_util::get("HOME") + "/foo"},
|
|
{"/scratch/polybar", "/scratch/polybar"},
|
|
};
|
|
|
|
INSTANTIATE_TEST_SUITE_P(Inst, ExpandTest, ::testing::ValuesIn(expand_absolute_test_list));
|
|
|
|
TEST_P(ExpandTest, absolute) {
|
|
EXPECT_EQ(file_util::expand(GetParam().first), GetParam().second);
|
|
}
|
|
|
|
TEST_P(ExpandTest, relativeToAbsolute) {
|
|
EXPECT_EQ(file_util::expand(GetParam().first, "/scratch"), GetParam().second);
|
|
}
|
|
|
|
using expand_relative_test_t = std::tuple<string, string, string>;
|
|
class ExpandRelativeTest : public testing::TestWithParam<expand_relative_test_t> {};
|
|
|
|
vector<expand_relative_test_t> expand_relative_test_list = {
|
|
{"../test", "/scratch", "/scratch/../test"},
|
|
{"modules/battery", "/scratch/polybar", "/scratch/polybar/modules/battery"},
|
|
{"/tmp/foo", "/scratch", "/tmp/foo"},
|
|
};
|
|
|
|
INSTANTIATE_TEST_SUITE_P(Inst, ExpandRelativeTest, ::testing::ValuesIn(expand_relative_test_list));
|
|
|
|
TEST_P(ExpandRelativeTest, correctness) {
|
|
string path, relative_to, expected;
|
|
std::tie(path, relative_to, expected) = GetParam();
|
|
EXPECT_EQ(file_util::expand(path, relative_to), expected);
|
|
}
|