mirror of
https://github.com/polybar/polybar.git
synced 2024-10-27 05:23:39 -04:00
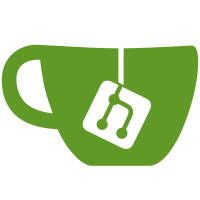
The intent is for every color to be stored in a rgba instance The rgba class now stores the color in a 32 bit integer to save space This also removes the unused class rgb and moves everything else into a cpp file. Many functions also had weird template parameters. For example alpha_channel<unsigned short int> would give a 2 byte number with the alpha channel byte in both bytes. color_util::hex would return a hex string with alpha channel if unsigned short int was given and without if unsigned char was given. Even more curiously those parameters were passed to *_channel and the result nevertheless truncated to 8bits.
53 lines
1 KiB
C++
53 lines
1 KiB
C++
#pragma once
|
|
|
|
#include <cstdlib>
|
|
|
|
#include "common.hpp"
|
|
#include "utils/cache.hpp"
|
|
|
|
POLYBAR_NS
|
|
|
|
static cache<string, uint32_t> g_cache_hex;
|
|
|
|
struct rgba {
|
|
/**
|
|
* Color value in the form ARGB or A000 depending on the type
|
|
*/
|
|
uint32_t m_value;
|
|
|
|
enum color_type { NONE, ARGB, ALPHA_ONLY } m_type{NONE};
|
|
|
|
explicit rgba();
|
|
explicit rgba(uint32_t value, color_type type = ARGB);
|
|
explicit rgba(string hex);
|
|
|
|
operator string() const;
|
|
operator uint32_t() const;
|
|
bool operator==(const rgba& other) const;
|
|
|
|
double a() const;
|
|
double r() const;
|
|
double g() const;
|
|
double b() const;
|
|
|
|
uint8_t a_int() const;
|
|
uint8_t r_int() const;
|
|
uint8_t g_int() const;
|
|
uint8_t b_int() const;
|
|
|
|
bool has_color() const;
|
|
};
|
|
|
|
namespace color_util {
|
|
|
|
uint8_t alpha_channel(const uint32_t value);
|
|
uint8_t red_channel(const uint32_t value);
|
|
uint8_t green_channel(const uint32_t value);
|
|
uint8_t blue_channel(const uint32_t value);
|
|
|
|
string hex(uint32_t color);
|
|
|
|
string simplify_hex(string hex);
|
|
} // namespace color_util
|
|
|
|
POLYBAR_NS_END
|