mirror of
https://github.com/polybar/polybar.git
synced 2024-10-27 05:23:39 -04:00
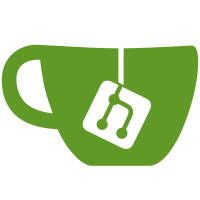
expand-right defaults to true to preserve the current functionality If set to false, the items in the menu will be added to the left of the toggle label (instead of the right side) Should resolve the issue discussed in #655
48 lines
1 KiB
C++
48 lines
1 KiB
C++
#pragma once
|
|
|
|
#include "modules/meta/input_handler.hpp"
|
|
#include "modules/meta/static_module.hpp"
|
|
|
|
POLYBAR_NS
|
|
|
|
namespace modules {
|
|
class menu_module : public static_module<menu_module>, public input_handler {
|
|
public:
|
|
struct menu_tree_item {
|
|
string exec;
|
|
label_t label;
|
|
};
|
|
|
|
struct menu_tree {
|
|
vector<unique_ptr<menu_tree_item>> items;
|
|
};
|
|
|
|
public:
|
|
explicit menu_module(const bar_settings&, string);
|
|
|
|
bool build(builder* builder, const string& tag) const;
|
|
void update() {}
|
|
|
|
protected:
|
|
bool input(string&& cmd);
|
|
|
|
private:
|
|
static constexpr auto TAG_LABEL_TOGGLE = "<label-toggle>";
|
|
static constexpr auto TAG_MENU = "<menu>";
|
|
|
|
static constexpr auto EVENT_MENU_OPEN = "menu-open-";
|
|
static constexpr auto EVENT_MENU_CLOSE = "menu-close";
|
|
|
|
bool m_expand_right{true};
|
|
|
|
label_t m_labelopen;
|
|
label_t m_labelclose;
|
|
label_t m_labelseparator;
|
|
|
|
vector<unique_ptr<menu_tree>> m_levels;
|
|
|
|
std::atomic<int> m_level{-1};
|
|
};
|
|
}
|
|
|
|
POLYBAR_NS_END
|