mirror of
https://github.com/polybar/polybar.git
synced 2024-10-27 05:23:39 -04:00
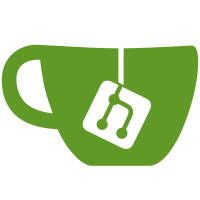
Since the forked processes are still our children, we need to wait on them, otherwise they become zombie processes. We now fork twice, let the first fork immediately return and wait on it. This reparents the second fork, which runs the actual code, to the init process which then collects it. Ref #770
32 lines
814 B
C++
32 lines
814 B
C++
#pragma once
|
|
|
|
#include <sys/types.h>
|
|
|
|
#include "common.hpp"
|
|
|
|
POLYBAR_NS
|
|
|
|
namespace process_util {
|
|
bool in_parent_process(pid_t pid);
|
|
bool in_forked_process(pid_t pid);
|
|
|
|
void redirect_stdio_to_dev_null();
|
|
|
|
pid_t spawn_async(std::function<void()> const& lambda);
|
|
void fork_detached(std::function<void()> const& lambda);
|
|
|
|
void exec(char* cmd, char** args);
|
|
void exec_sh(const char* cmd);
|
|
|
|
int wait(pid_t pid);
|
|
|
|
pid_t wait_for_completion(pid_t process_id, int* status_addr = nullptr, int waitflags = 0);
|
|
pid_t wait_for_completion(int* status_addr, int waitflags = 0);
|
|
pid_t wait_for_completion_nohang(pid_t process_id, int* status);
|
|
pid_t wait_for_completion_nohang(int* status);
|
|
pid_t wait_for_completion_nohang();
|
|
|
|
bool notify_childprocess();
|
|
} // namespace process_util
|
|
|
|
POLYBAR_NS_END
|