mirror of
https://github.com/polybar/polybar.git
synced 2024-10-27 05:23:39 -04:00
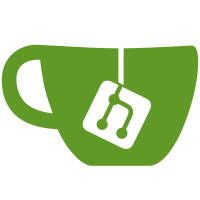
This adds the 'send' action to the ipc module that can be used to send arbitrary text to the module: polybar-msg action "#ipc.send.%{F#4444ff}hello%{F-}" * feat(ipc): allow receiving arbitrary text on IPC socket Instead of just allowing hook numbers to be executed, the user can send arbitrary text and the IPC module will put it in the bar. The IPC payload format is extended to accept an arbitrary string if the first character after the module name is ':'. polybar-msg hook test :'%{F#4444ff}hello%{F-}' Fix #2455 * Use actions for sending data to ipc module * ipc: Don't use exceptions when no hooks are defined * Update src/modules/ipc.cpp Co-authored-by: patrick96 <p.ziegler96@gmail.com>
51 lines
1.2 KiB
C++
51 lines
1.2 KiB
C++
#pragma once
|
|
|
|
#include "modules/meta/static_module.hpp"
|
|
#include "utils/command.hpp"
|
|
|
|
POLYBAR_NS
|
|
|
|
namespace modules {
|
|
/**
|
|
* Module that allow users to configure hooks on
|
|
* received ipc messages. The hook will execute the defined
|
|
* shell script and the resulting output will be used
|
|
* as the module content.
|
|
*/
|
|
class ipc_module : public static_module<ipc_module> {
|
|
public:
|
|
/**
|
|
* Hook structure that will be fired
|
|
* when receiving a message with specified id
|
|
*/
|
|
struct hook {
|
|
string payload;
|
|
string command;
|
|
};
|
|
|
|
public:
|
|
explicit ipc_module(const bar_settings&, string);
|
|
|
|
void start() override;
|
|
void update() {}
|
|
string get_output();
|
|
bool build(builder* builder, const string& tag) const;
|
|
void on_message(const string& message);
|
|
|
|
static constexpr auto TYPE = "custom/ipc";
|
|
|
|
static constexpr auto EVENT_SEND = "send";
|
|
|
|
protected:
|
|
void action_send(const string& data);
|
|
|
|
private:
|
|
static constexpr const char* TAG_OUTPUT{"<output>"};
|
|
vector<unique_ptr<hook>> m_hooks;
|
|
map<mousebtn, string> m_actions;
|
|
string m_output;
|
|
size_t m_initial;
|
|
};
|
|
} // namespace modules
|
|
|
|
POLYBAR_NS_END
|