mirror of
https://github.com/avelino/awesome-go.git
synced 2024-10-30 12:04:12 -04:00
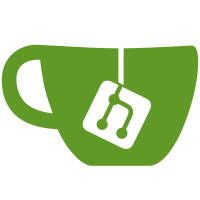
* fix typo in README.md fixes #3204 * #1446 implement test for stale repositories * fix #1446 * fixes #3211 added check if issue has not been previously opened * fixes #3211 add limit to number of issues created at a time * fixes #3211 reformat issue message * checks for dead links as well * fixes #3211 handle status code 302 and 301 * fixes #3211 handle status code 302 and 301 * fixes #3211 handle status code 302 and 301 * fixes #3211 test workflow * fixes #3211 test workflow * fixes #3211 test workflow again * fixes #3211 test workflow again * remove workflows and start over * re add workflow * apply review suggestions * add environment variable. modify workflow to run once a week * add check for archived repositories and reformat * reformat code to improve readability * reformat to improve readability * cause continue and not break if href not found * satisfy code climate requirements
29 lines
529 B
Go
29 lines
529 B
Go
package main
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
"io/ioutil"
|
|
|
|
"github.com/PuerkitoBio/goquery"
|
|
"github.com/russross/blackfriday"
|
|
)
|
|
|
|
func readme() []byte {
|
|
input, err := ioutil.ReadFile("./README.md")
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
html := fmt.Sprintf("<body>%s</body>", blackfriday.MarkdownCommon(input))
|
|
htmlByteArray := []byte(html)
|
|
return htmlByteArray
|
|
}
|
|
|
|
func startQuery() *goquery.Document {
|
|
buf := bytes.NewBuffer(readme())
|
|
query, err := goquery.NewDocumentFromReader(buf)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return query
|
|
}
|