mirror of
https://github.com/awesome-print/awesome_print
synced 2023-03-27 23:22:34 -04:00
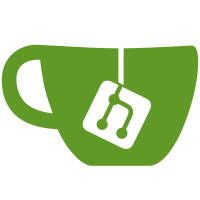
Use the ruby 1.9 hash syntax everywhere appropriate. This is to fix style inconsistencies in the code base. This is also so that Hound can be used without it bugging people every time they touch an older piece of code but forget to update the syntax. If git-blame brought you here you may want to read this, the problem is with git-blame, not this change. Try running these two lines just once: ``` git config --global alias.praise 'log -p -M --follow --stat --' git config --global alias.praise-line 'log -p -M --pretty=format:"%h (%an %ai)" -L' ``` Now in future you can use `git praise <path/to/your/file>` or if you want to see the evolution of a specific line or range of lines `git praise-line <start-line>:<end-line>:<path/to/your/file>` Some examples you should try: ``` git praise lib/awesome_print/version.rb git praise-line 8:8:lib/awesome_print/version.rb ``` Inspiration for these aliases: http://blog.andrewray.me/a-better-git-blame/
102 lines
2.6 KiB
Ruby
102 lines
2.6 KiB
Ruby
require 'spec_helper'
|
|
|
|
RSpec.describe 'AwesomePrint' do
|
|
def stub_tty!(output = true, stream = STDOUT)
|
|
if output
|
|
stream.instance_eval { def tty?; true; end }
|
|
else
|
|
stream.instance_eval { def tty?; false; end }
|
|
end
|
|
end
|
|
|
|
describe 'colorization' do
|
|
PLAIN = '[ 1, :two, "three", [ nil, [ true, false ] ] ]'
|
|
COLORIZED = "[ \e[1;34m1\e[0m, \e[0;36m:two\e[0m, \e[0;33m\"three\"\e[0m, [ \e[1;31mnil\e[0m, [ \e[1;32mtrue\e[0m, \e[1;31mfalse\e[0m ] ] ]"
|
|
|
|
before do
|
|
ENV['TERM'] = 'xterm-colors'
|
|
ENV.delete('ANSICON')
|
|
@arr = [ 1, :two, 'three', [ nil, [ true, false] ] ]
|
|
end
|
|
|
|
describe 'default settings (no forced colors)' do
|
|
before do
|
|
AwesomePrint.force_colors! false
|
|
end
|
|
|
|
it 'colorizes tty processes by default' do
|
|
stub_tty!
|
|
expect(@arr.ai(multiline: false)).to eq(COLORIZED)
|
|
end
|
|
|
|
it "colorizes processes with ENV['ANSICON'] by default" do
|
|
begin
|
|
stub_tty!
|
|
term, ENV['ANSICON'] = ENV['ANSICON'], '1'
|
|
expect(@arr.ai(multiline: false)).to eq(COLORIZED)
|
|
ensure
|
|
ENV['ANSICON'] = term
|
|
end
|
|
end
|
|
|
|
it 'does not colorize tty processes running in dumb terminals by default' do
|
|
begin
|
|
stub_tty!
|
|
term, ENV['TERM'] = ENV['TERM'], 'dumb'
|
|
expect(@arr.ai(multiline: false)).to eq(PLAIN)
|
|
ensure
|
|
ENV['TERM'] = term
|
|
end
|
|
end
|
|
|
|
it 'does not colorize subprocesses by default' do
|
|
begin
|
|
stub_tty! false
|
|
expect(@arr.ai(multiline: false)).to eq(PLAIN)
|
|
ensure
|
|
stub_tty!
|
|
end
|
|
end
|
|
end
|
|
|
|
describe 'forced colors override' do
|
|
before do
|
|
AwesomePrint.force_colors!
|
|
end
|
|
|
|
it 'still colorizes tty processes' do
|
|
stub_tty!
|
|
expect(@arr.ai(multiline: false)).to eq(COLORIZED)
|
|
end
|
|
|
|
it "colorizes processes with ENV['ANSICON'] set to 0" do
|
|
begin
|
|
stub_tty!
|
|
term, ENV['ANSICON'] = ENV['ANSICON'], '1'
|
|
expect(@arr.ai(multiline: false)).to eq(COLORIZED)
|
|
ensure
|
|
ENV['ANSICON'] = term
|
|
end
|
|
end
|
|
|
|
it 'colorizes dumb terminals' do
|
|
begin
|
|
stub_tty!
|
|
term, ENV['TERM'] = ENV['TERM'], 'dumb'
|
|
expect(@arr.ai(multiline: false)).to eq(COLORIZED)
|
|
ensure
|
|
ENV['TERM'] = term
|
|
end
|
|
end
|
|
|
|
it 'colorizes subprocess' do
|
|
begin
|
|
stub_tty! false
|
|
expect(@arr.ai(multiline: false)).to eq(COLORIZED)
|
|
ensure
|
|
stub_tty!
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|