mirror of
https://github.com/awesome-print/awesome_print
synced 2023-03-27 23:22:34 -04:00
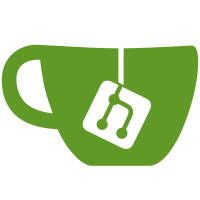
The previous stubbing of dotfiles was still allowing calls to fall through and populate the AwesomePrint.defaults hash which would then cause some tests to fail if run in a certain order. e.g. https://github.com/awesome-print/awesome_print/issues/265 One place in particular where this would happen is if you ran the action_view_spec before other specs as it did not stub the dotfile. This change makes dotfile stubbing more reliable, specific and ensures it is run for every spec. I've also removed all instances where it was being called manually, as this is now not needed due to it being automatic.
46 lines
1.4 KiB
Ruby
46 lines
1.4 KiB
Ruby
require 'spec_helper'
|
|
|
|
RSpec.describe "AwesomePrint/Nokogiri" do
|
|
it "should colorize tags" do
|
|
xml = Nokogiri::XML('<html><body><h1></h1></body></html>')
|
|
expect(xml.ai).to eq <<-EOS
|
|
<?xml version=\"1.0\"?>\e[1;32m
|
|
\e[0m<\e[1;36mhtml\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mbody\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mh1\e[0m/>\e[1;32m
|
|
\e[0m<\e[1;36m/body\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36m/html\e[0m>
|
|
EOS
|
|
end
|
|
|
|
it "should colorize contents" do
|
|
xml = Nokogiri::XML('<html><body><h1>Hello</h1></body></html>')
|
|
expect(xml.ai).to eq <<-EOS
|
|
<?xml version=\"1.0\"?>\e[1;32m
|
|
\e[0m<\e[1;36mhtml\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mbody\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mh1\e[0m>\e[1;32mHello\e[0m<\e[1;36m/h1\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36m/body\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36m/html\e[0m>
|
|
EOS
|
|
end
|
|
|
|
it "should colorize class and id" do
|
|
xml = Nokogiri::XML('<html><body><h1><span id="hello" class="world"></span></h1></body></html>')
|
|
expect(xml.ai).to eq <<-EOS
|
|
<?xml version=\"1.0\"?>\e[1;32m
|
|
\e[0m<\e[1;36mhtml\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mbody\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mh1\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36mspan\e[0m \e[1;33mid=\"hello\"\e[0m \e[1;33mclass=\"world\"\e[0m/>\e[1;32m
|
|
\e[0m<\e[1;36m/h1\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36m/body\e[0m>\e[1;32m
|
|
\e[0m<\e[1;36m/html\e[0m>
|
|
EOS
|
|
end
|
|
|
|
it "handle empty NodeSet" do
|
|
xml = Nokogiri::XML::NodeSet.new(Nokogiri::XML(''))
|
|
expect(xml.ai).to eq('[]')
|
|
end
|
|
end
|