mirror of
https://github.com/capistrano/capistrano
synced 2023-03-27 23:21:18 -04:00
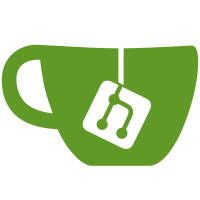
Cucumber has deprecated `puts` in favor of `log` for logging messages to the current cucumber formatter. In our case we actually want it both ways: we want to log messages using the cucumber formatter when cucumber is running, but use `Kernel#puts` otherwise. So, use `respond_to?` to see if cucumber's `log` is available, and if not, fall back to `puts`.
41 lines
1.1 KiB
Ruby
41 lines
1.1 KiB
Ruby
require "open3"
|
|
|
|
module VagrantHelpers
|
|
extend self
|
|
|
|
class VagrantSSHCommandError < RuntimeError; end
|
|
|
|
at_exit do
|
|
if ENV["KEEP_RUNNING"]
|
|
puts "Vagrant vm will be left up because KEEP_RUNNING is set."
|
|
puts "Rerun without KEEP_RUNNING set to cleanup the vm."
|
|
else
|
|
vagrant_cli_command("destroy -f")
|
|
end
|
|
end
|
|
|
|
def vagrant_cli_command(command)
|
|
puts "[vagrant] #{command}"
|
|
stdout, stderr, status = Dir.chdir(VAGRANT_ROOT) do
|
|
Open3.capture3("#{VAGRANT_BIN} #{command}")
|
|
end
|
|
|
|
(stdout + stderr).each_line { |line| puts "[vagrant] #{line}" }
|
|
|
|
[stdout, stderr, status]
|
|
end
|
|
|
|
def run_vagrant_command(command)
|
|
stdout, stderr, status = vagrant_cli_command("ssh -c #{command.inspect}")
|
|
return [stdout, stderr] if status.success?
|
|
raise VagrantSSHCommandError, status
|
|
end
|
|
|
|
def puts(message)
|
|
# Attach log messages to the current cucumber feature (`log`),
|
|
# or simply puts to the console (`super`) if we are outside of cucumber.
|
|
respond_to?(:log) ? log(message) : super(message)
|
|
end
|
|
end
|
|
|
|
World(VagrantHelpers)
|