mirror of
https://github.com/mperham/connection_pool
synced 2023-03-27 23:22:21 -04:00
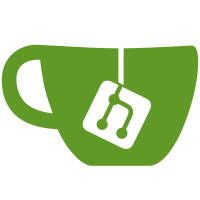
This means that, when not saturated, objects in the pool will be reused more often. This behavior allows you to create a pool of objects which in turn have some kind of time outs themselves. For instance, objects which keep an underlying TCP connection open until they're idle for some time. An example of this is `Net::HTTP::Persistent`. See also issue #20.
42 lines
747 B
Ruby
42 lines
747 B
Ruby
require 'thread'
|
|
require 'timeout'
|
|
|
|
class TimedQueue
|
|
def initialize(size = 0)
|
|
@que = Array.new(size) { yield }
|
|
@mutex = Mutex.new
|
|
@resource = ConditionVariable.new
|
|
end
|
|
|
|
def push(obj)
|
|
@mutex.synchronize do
|
|
@que.unshift obj
|
|
@resource.broadcast
|
|
end
|
|
end
|
|
alias_method :<<, :push
|
|
|
|
def timed_pop(timeout=0.5)
|
|
deadline = Time.now + timeout
|
|
@mutex.synchronize do
|
|
loop do
|
|
return @que.shift unless @que.empty?
|
|
to_wait = deadline - Time.now
|
|
raise Timeout::Error, "Waited #{timeout} sec" if to_wait <= 0
|
|
@resource.wait(@mutex, to_wait)
|
|
end
|
|
end
|
|
end
|
|
|
|
def empty?
|
|
@que.empty?
|
|
end
|
|
|
|
def clear
|
|
@que.clear
|
|
end
|
|
|
|
def length
|
|
@que.length
|
|
end
|
|
end
|