mirror of
https://github.com/mperham/connection_pool
synced 2023-03-27 23:22:21 -04:00
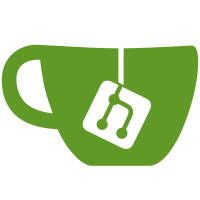
Now TimedStack only creates connections when the internal queue is empty and the number of connections is less than the maximum set. By default no connections are created when the pool is created. This required some changes to the TimedStack tests because I wrote poor tests the first time around. The new tests better exercise the intended behavior of TimedStack. This required some changes to the ConnectionPool tests to use up connections because now an unused pool has no connections.
123 lines
1.9 KiB
Ruby
123 lines
1.9 KiB
Ruby
Thread.abort_on_exception = true
|
|
require 'helper'
|
|
|
|
class TestConnectionPoolTimedStack < Minitest::Test
|
|
|
|
def setup
|
|
@stack = ConnectionPool::TimedStack.new { Object.new }
|
|
end
|
|
|
|
def test_empty_eh
|
|
stack = ConnectionPool::TimedStack.new(1) { Object.new }
|
|
|
|
refute_empty stack
|
|
|
|
popped = stack.pop
|
|
|
|
assert_empty stack
|
|
|
|
stack.push popped
|
|
|
|
refute_empty stack
|
|
end
|
|
|
|
def test_length
|
|
stack = ConnectionPool::TimedStack.new(1) { Object.new }
|
|
|
|
assert_equal 1, stack.length
|
|
|
|
popped = stack.pop
|
|
|
|
assert_equal 0, stack.length
|
|
|
|
stack.push popped
|
|
|
|
assert_equal 1, stack.length
|
|
end
|
|
|
|
def test_pop
|
|
object = Object.new
|
|
@stack.push object
|
|
|
|
popped = @stack.pop
|
|
|
|
assert_same object, popped
|
|
end
|
|
|
|
def test_pop_empty
|
|
e = assert_raises Timeout::Error do
|
|
@stack.pop 0
|
|
end
|
|
|
|
assert_equal 'Waited 0 sec', e.message
|
|
end
|
|
|
|
def test_pop_full
|
|
stack = ConnectionPool::TimedStack.new(1) { Object.new }
|
|
|
|
popped = stack.pop
|
|
|
|
refute_nil popped
|
|
assert_empty stack
|
|
end
|
|
|
|
def test_pop_wait
|
|
thread = Thread.start do
|
|
@stack.pop
|
|
end
|
|
|
|
Thread.pass while thread.status == 'run'
|
|
|
|
object = Object.new
|
|
|
|
@stack.push object
|
|
|
|
assert_same object, thread.value
|
|
end
|
|
|
|
def test_pop_shutdown
|
|
@stack.shutdown { }
|
|
|
|
assert_raises ConnectionPool::PoolShuttingDownError do
|
|
@stack.pop
|
|
end
|
|
end
|
|
|
|
def test_push
|
|
stack = ConnectionPool::TimedStack.new(1) { Object.new }
|
|
|
|
conn = stack.pop
|
|
|
|
stack.push conn
|
|
|
|
refute_empty stack
|
|
end
|
|
|
|
def test_push_shutdown
|
|
called = []
|
|
|
|
@stack.shutdown do |object|
|
|
called << object
|
|
end
|
|
|
|
@stack.push Object.new
|
|
|
|
refute_empty called
|
|
assert_empty @stack
|
|
end
|
|
|
|
def test_shutdown
|
|
@stack.push Object.new
|
|
|
|
called = []
|
|
|
|
@stack.shutdown do |object|
|
|
called << object
|
|
end
|
|
|
|
refute_empty called
|
|
assert_empty @stack
|
|
end
|
|
|
|
end
|
|
|