mirror of
https://github.com/fog/fog-aws.git
synced 2022-11-09 13:50:52 -05:00
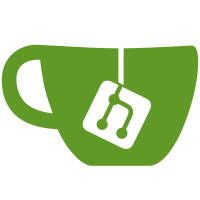
Also fix up various things that rubocop called out, though not everything. Mostly whitespace fixes, changing double-quotes to single if double wasn't required, changing to use ruby 2.x hash syntax where possible, etc. While tests don't run, they are no more broken than before (at least, as far as I can tell).
90 lines
2.3 KiB
Ruby
90 lines
2.3 KiB
Ruby
def collection_tests(collection, params = {}, mocks_implemented = true)
|
|
tests('success') do
|
|
tests("#new(#{params.inspect})").succeeds do
|
|
pending if Fog.mocking? && !mocks_implemented
|
|
collection.new(params)
|
|
end
|
|
|
|
tests("#create(#{params.inspect})").succeeds do
|
|
pending if Fog.mocking? && !mocks_implemented
|
|
@instance = collection.create(params)
|
|
end
|
|
# FIXME: work around for timing issue on AWS describe_instances mocks
|
|
|
|
if Fog.mocking? && @instance.respond_to?(:ready?)
|
|
@instance.wait_for { ready? }
|
|
end
|
|
|
|
tests("#all").succeeds do
|
|
pending if Fog.mocking? && !mocks_implemented
|
|
collection.all
|
|
end
|
|
|
|
if !Fog.mocking? || mocks_implemented
|
|
@identity = @instance.identity
|
|
end
|
|
|
|
tests("#get(#{@identity})").succeeds do
|
|
pending if Fog.mocking? && !mocks_implemented
|
|
collection.get(@identity)
|
|
end
|
|
|
|
tests('Enumerable') do
|
|
pending if Fog.mocking? && !mocks_implemented
|
|
|
|
methods = [
|
|
'all?', 'any?', 'find', 'detect', 'collect', 'map',
|
|
'find_index', 'flat_map', 'collect_concat', 'group_by',
|
|
'none?', 'one?'
|
|
]
|
|
|
|
methods.each do |enum_method|
|
|
if collection.respond_to?(enum_method)
|
|
tests("##{enum_method}").succeeds do
|
|
block_called = false
|
|
collection.send(enum_method) { block_called = true }
|
|
block_called
|
|
end
|
|
end
|
|
end
|
|
|
|
[
|
|
'max_by','min_by'
|
|
].each do |enum_method|
|
|
if collection.respond_to?(enum_method)
|
|
tests("##{enum_method}").succeeds do
|
|
block_called = false
|
|
collection.send(enum_method) { block_called = true; 0 }
|
|
block_called
|
|
end
|
|
end
|
|
|
|
end
|
|
|
|
end
|
|
|
|
if block_given?
|
|
yield(@instance)
|
|
end
|
|
|
|
if !Fog.mocking? || mocks_implemented
|
|
@instance.destroy
|
|
end
|
|
end
|
|
|
|
tests('failure') do
|
|
|
|
if !Fog.mocking? || mocks_implemented
|
|
@identity = @identity.to_s
|
|
@identity = @identity.gsub(/[a-zA-Z]/) { Fog::Mock.random_letters(1) }
|
|
@identity = @identity.gsub(/\d/) { Fog::Mock.random_numbers(1) }
|
|
@identity
|
|
end
|
|
|
|
tests("#get('#{@identity}')").returns(nil) do
|
|
pending if Fog.mocking? && !mocks_implemented
|
|
collection.get(@identity)
|
|
end
|
|
|
|
end
|
|
end
|