mirror of
https://github.com/fog/fog.git
synced 2022-11-09 13:51:43 -05:00
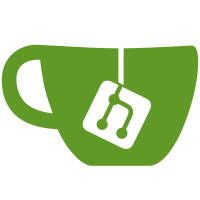
Refactor ninefold to provide generic Atmos support. Add an additional argument, when compared to ninefold, which is the endpoint. The endpoint should be a full URL, e.g. https://storage.provider.com:1337/atmos. The API path and port are optional. If the port is not specified, it is inferred from the protocol.
107 lines
3.1 KiB
Ruby
107 lines
3.1 KiB
Ruby
require 'fog/core/model'
|
|
|
|
module Fog
|
|
module Storage
|
|
class Atmos
|
|
|
|
class File < Fog::Model
|
|
|
|
identity :key, :aliases => :Filename
|
|
|
|
attribute :content_length, :aliases => ['bytes', 'Content-Length'], :type => :integer
|
|
attribute :content_type, :aliases => ['content_type', 'Content-Type']
|
|
attribute :objectid, :aliases => :ObjectID
|
|
|
|
def body
|
|
attributes[:body] ||= if objectid
|
|
collection.get(identity).body
|
|
else
|
|
''
|
|
end
|
|
end
|
|
|
|
def body=(new_body)
|
|
attributes[:body] = new_body
|
|
end
|
|
|
|
def directory
|
|
@directory
|
|
end
|
|
|
|
def copy(target_directory_key, target_file_key, options={})
|
|
target_directory = connection.directories.new(:key => target_directory_key)
|
|
target_directory.files.create(
|
|
:key => target_file_key,
|
|
:body => body
|
|
)
|
|
end
|
|
|
|
def destroy
|
|
requires :directory, :key
|
|
connection.delete_namespace([directory.key, key].join('/'))
|
|
true
|
|
end
|
|
|
|
# def owner=(new_owner)
|
|
# if new_owner
|
|
# attributes[:owner] = {
|
|
# :display_name => new_owner['DisplayName'],
|
|
# :id => new_owner['ID']
|
|
# }
|
|
# end
|
|
# end
|
|
|
|
def public=(new_public)
|
|
# NOOP - we don't need to flag files as public, getting the public URL for a file handles it.
|
|
end
|
|
|
|
# By default, expire in 5 years
|
|
def public_url(expires = (Time.now + 5 * 365 * 24 * 60 * 60))
|
|
requires :objectid
|
|
# TODO - more efficient method to get this?
|
|
storage = Fog::Storage.new(:provider => 'Atmos')
|
|
uri = URI::HTTP.build(:scheme => @prefix, :host => @storage_host, :port => @storage_port.to_i, :path => "/rest/objects/#{objectid}" )
|
|
Fog::Storage.new(:provider => 'Atmos').uid
|
|
|
|
sb = "GET\n"
|
|
sb += uri.path.downcase + "\n"
|
|
sb += storage.uid + "\n"
|
|
sb += String(expires.to_i())
|
|
|
|
signature = storage.sign( sb )
|
|
uri.query = "uid=#{CGI::escape(storage.uid)}&expires=#{expires.to_i()}&signature=#{CGI::escape(signature)}"
|
|
uri.to_s
|
|
end
|
|
|
|
def save(options = {})
|
|
requires :body, :directory, :key
|
|
directory.kind_of?(Directory) ? ns = directory.key : ns = directory
|
|
ns += key
|
|
options[:headers] ||= {}
|
|
options[:headers]['Content-Type'] = content_type if content_type
|
|
options[:body] = body
|
|
begin
|
|
data = connection.post_namespace(ns, options)
|
|
self.objectid = data.headers['location'].split('/')[-1]
|
|
rescue => error
|
|
if error.message =~ /The resource you are trying to create already exists./
|
|
data = connection.put_namespace(ns, options)
|
|
else
|
|
raise error
|
|
end
|
|
end
|
|
# merge_attributes(data.headers)
|
|
true
|
|
end
|
|
|
|
private
|
|
|
|
def directory=(new_directory)
|
|
@directory = new_directory
|
|
end
|
|
|
|
end
|
|
|
|
end
|
|
end
|
|
end
|