mirror of
https://github.com/fog/fog.git
synced 2022-11-09 13:51:43 -05:00
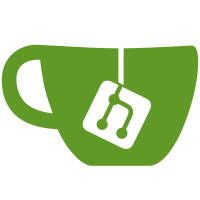
This function allows you to get the ip address of the current machine. I found this useful because I wanted to add my personal or production machine to a specific RDS security group. The call is quite simple and connects to amazon's checkip website. The class is threadsafe and I have included specs with it as well. I have also added the Fog::AWS::RDS::SecurityGroup#authorize_me function to make adding the current machine to a given RDS security group very easy.
38 lines
1 KiB
Ruby
38 lines
1 KiB
Ruby
require 'net/http'
|
|
require 'uri'
|
|
|
|
module Fog
|
|
class CurrentMachine
|
|
@@lock = Mutex.new
|
|
AMAZON_AWS_CHECK_IP = 'http://checkip.amazonaws.com'
|
|
|
|
def self.ip_address= ip_address
|
|
@@lock.synchronize do
|
|
@@ip_address = ip_address
|
|
end
|
|
end
|
|
|
|
# Get the ip address of the machine from which this command is run. It is
|
|
# recommended that you surround calls to this function with a timeout block
|
|
# to ensure optimum performance in the case where the amazonaws checkip
|
|
# service is unavailable.
|
|
#
|
|
# @example Get the current ip address
|
|
# begin
|
|
# Timeout::timeout(5) do
|
|
# puts "Your ip address is #{Fog::CurrentMachine.ip_address}"
|
|
# end
|
|
# rescue Timeout::Error
|
|
# puts "Service timeout"
|
|
# end
|
|
#
|
|
# @raise [Net::HTTPExceptions] if the net/http request fails.
|
|
def self.ip_address
|
|
@@lock.synchronize do
|
|
@@ip_address ||= Net::HTTP \
|
|
.get_response(URI.parse(AMAZON_AWS_CHECK_IP)) \
|
|
.body.chomp
|
|
end
|
|
end
|
|
end
|
|
end
|