mirror of
https://github.com/fog/fog.git
synced 2022-11-09 13:51:43 -05:00
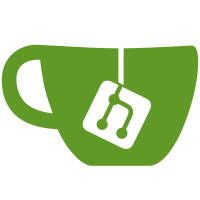
This moves tag_sets out of individual resources and up to a top-level item in each mock account's hash. Starting with images, this allows other mock accounts to create and use their own tags on images they have launchPermission on.
72 lines
2.3 KiB
Ruby
72 lines
2.3 KiB
Ruby
module Fog
|
|
module Compute
|
|
class AWS
|
|
class Real
|
|
|
|
require 'fog/aws/parsers/compute/create_snapshot'
|
|
|
|
# Create a snapshot of an EBS volume and store it in S3
|
|
#
|
|
# ==== Parameters
|
|
# * volume_id<~String> - Id of EBS volume to snapshot
|
|
#
|
|
# ==== Returns
|
|
# * response<~Excon::Response>:
|
|
# * body<~Hash>:
|
|
# * 'progress'<~String> - The percentage progress of the snapshot
|
|
# * 'requestId'<~String> - id of request
|
|
# * 'snapshotId'<~String> - id of snapshot
|
|
# * 'startTime'<~Time> - timestamp when snapshot was initiated
|
|
# * 'status'<~String> - state of snapshot
|
|
# * 'volumeId'<~String> - id of volume snapshot targets
|
|
#
|
|
# {Amazon API Reference}[http://docs.amazonwebservices.com/AWSEC2/latest/APIReference/ApiReference-query-CreateSnapshot.html]
|
|
def create_snapshot(volume_id, description = nil)
|
|
request(
|
|
'Action' => 'CreateSnapshot',
|
|
'Description' => description,
|
|
'VolumeId' => volume_id,
|
|
:parser => Fog::Parsers::Compute::AWS::CreateSnapshot.new
|
|
)
|
|
end
|
|
|
|
end
|
|
|
|
class Mock
|
|
|
|
#
|
|
# Usage
|
|
#
|
|
# AWS[:compute].create_snapshot("vol-f7c23423", "latest snapshot")
|
|
#
|
|
|
|
def create_snapshot(volume_id, description = nil)
|
|
response = Excon::Response.new
|
|
if volume = self.data[:volumes][volume_id]
|
|
response.status = 200
|
|
snapshot_id = Fog::AWS::Mock.snapshot_id
|
|
data = {
|
|
'description' => description,
|
|
'ownerId' => self.data[:owner_id],
|
|
'progress' => nil,
|
|
'snapshotId' => snapshot_id,
|
|
'startTime' => Time.now,
|
|
'status' => 'pending',
|
|
'volumeId' => volume_id,
|
|
'volumeSize' => volume['size']
|
|
}
|
|
self.data[:snapshots][snapshot_id] = data
|
|
response.body = {
|
|
'requestId' => Fog::AWS::Mock.request_id
|
|
}.merge!(data)
|
|
else
|
|
response.status = 400
|
|
raise(Excon::Errors.status_error({:expects => 200}, response))
|
|
end
|
|
response
|
|
end
|
|
|
|
end
|
|
end
|
|
end
|
|
end
|