mirror of
https://github.com/fog/fog.git
synced 2022-11-09 13:51:43 -05:00
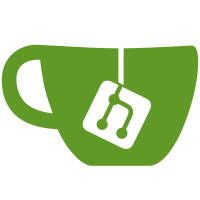
Using a reference to the server object in the interfaces model leads to a circular dependency when calling .inspect() on the server. Specifically, every server object has a reference to an interfaces collection. Each instance of the collection has a reference to the server object. When attempting to print out the object (in CLI or by calling .inspect()), this results in a loop: the server object's attributes are printed, which calls .inspect() on the interfaces field, which in turn calls .inspect() on the server field, and so on. The patch makes a functional change to address this. The interfaces class now expects a server_id (which can be retrieved with server.id). The id is used internally when performing any lookups for that VM. The patch also adds server() and server=() helpers, such that callers that rely on this functionality will not break. Refs: #2938
67 lines
1.7 KiB
Ruby
67 lines
1.7 KiB
Ruby
require 'fog/core/collection'
|
|
require 'fog/vsphere/models/compute/interface'
|
|
|
|
module Fog
|
|
module Compute
|
|
class Vsphere
|
|
class Interfaces < Fog::Collection
|
|
model Fog::Compute::Vsphere::Interface
|
|
|
|
attribute :server_id
|
|
|
|
def all(filters = {})
|
|
requires :server_id
|
|
|
|
case server
|
|
when Fog::Compute::Vsphere::Server
|
|
load service.list_vm_interfaces(server.id)
|
|
when Fog::Compute::Vsphere::Template
|
|
load service.list_template_interfaces(server.id)
|
|
else
|
|
raise 'interfaces should have vm or template'
|
|
end
|
|
|
|
self.each { |interface| interface.server_id = server.id }
|
|
self
|
|
end
|
|
|
|
def get(id)
|
|
requires :server_id
|
|
|
|
case server
|
|
when Fog::Compute::Vsphere::Server
|
|
interface = service.get_vm_interface(server.id, :key => id, :mac=> id, :name => id)
|
|
when Fog::Compute::Vsphere::Template
|
|
interface = service.get_template_interfaces(server.id, :key => id, :mac=> id, :name => id)
|
|
else
|
|
|
|
raise 'interfaces should have vm or template'
|
|
end
|
|
|
|
if interface
|
|
Fog::Compute::Vsphere::Interface.new(interface.merge(:server_id => server.id, :service => service))
|
|
else
|
|
nil
|
|
end
|
|
end
|
|
|
|
def new(attributes = {})
|
|
if server_id
|
|
super({ :server_id => server_id }.merge(attributes))
|
|
else
|
|
super
|
|
end
|
|
end
|
|
|
|
def server
|
|
return nil if server_id.nil?
|
|
service.servers.get(server_id)
|
|
end
|
|
|
|
def server=(new_server)
|
|
server_id = new_server.id
|
|
end
|
|
end
|
|
end
|
|
end
|
|
end
|