mirror of
https://github.com/fog/fog.git
synced 2022-11-09 13:51:43 -05:00
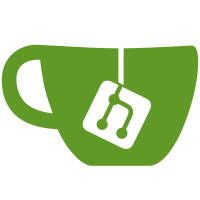
Check that server and disk destroy operation starts successfully Implement get and delete requests Handle 204 responses without body Return excon response with same status as the api returned Delete delete_operation api method that doesn't exist Add mocks for operations and use them in disk and server
55 lines
1.8 KiB
Ruby
55 lines
1.8 KiB
Ruby
module Fog
|
|
module Compute
|
|
class Google
|
|
|
|
class Mock
|
|
|
|
def delete_disk(disk_name, zone_name)
|
|
get_disk(disk_name, zone_name)
|
|
|
|
operation = self.random_operation
|
|
self.data[:operations][operation] = {
|
|
"kind" => "compute#operation",
|
|
"id" => Fog::Mock.random_numbers(19).to_s,
|
|
"name" => operation,
|
|
"zone" => "https://www.googleapis.com/compute/#{api_version}/projects/#{@project}/zones/#{zone_name}",
|
|
"operationType" => "delete",
|
|
"targetLink" => "https://www.googleapis.com/compute/#{api_version}/projects/#{@project}/zones/#{zone_name}/disks/#{disk_name}",
|
|
"targetId" => self.data[:disks][disk_name]["id"],
|
|
"status" => Fog::Compute::Google::Operation::PENDING_STATE,
|
|
"user" => "123456789012-qwertyuiopasdfghjkl1234567890qwe@developer.gserviceaccount.com",
|
|
"progress" => 0,
|
|
"insertTime" => Time.now.iso8601,
|
|
"startTime" => Time.now.iso8601,
|
|
"selfLink" => "https://www.googleapis.com/compute/#{api_version}/projects/#{@project}/zones/#{zone_name}/operations/#{operation}"
|
|
}
|
|
self.data[:disks].delete disk_name
|
|
|
|
build_response(:body => self.data[:operations][operation])
|
|
end
|
|
|
|
end
|
|
|
|
class Real
|
|
|
|
def delete_disk(disk_name, zone_name)
|
|
if zone_name.start_with? 'http'
|
|
zone_name = zone_name.split('/')[-1]
|
|
end
|
|
|
|
api_method = @compute.disks.delete
|
|
parameters = {
|
|
'project' => @project,
|
|
'disk' => disk_name,
|
|
'zone' => zone_name
|
|
}
|
|
|
|
result = self.build_result(api_method, parameters)
|
|
response = self.build_response(result)
|
|
end
|
|
|
|
end
|
|
|
|
end
|
|
end
|
|
end
|