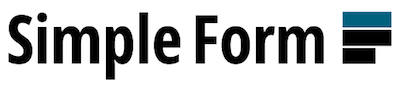
Rails forms made easy.
**Simple Form** aims to be as flexible as possible while helping you with powerful components to create
your forms. The basic goal of **Simple Form** is to not touch your way of defining the layout, letting
you find the better design for your eyes. Most of the DSL was inherited from Formtastic,
which we are thankful for and should make you feel right at home.
INFO: This README refers to **Simple Form** 5.0. For older releases, check the related branch for your version.
## Table of Contents
- [Installation](#installation)
- [Bootstrap](#bootstrap-5)
- [Zurb Foundation 5](#zurb-foundation-5)
- [Country Select](#country-select)
- [Usage](#usage)
- [Stripping away all wrapper divs](#stripping-away-all-wrapper-divs)
- [Collections](#collections)
- [Priority](#priority)
- [Associations](#associations)
- [Buttons](#buttons)
- [Wrapping Rails Form Helpers](#wrapping-rails-form-helpers)
- [Extra helpers](#extra-helpers)
- [Simple Fields For](#simple-fields-for)
- [Collection Radio Buttons](#collection-radio-buttons)
- [Collection Check Boxes](#collection-check-boxes)
- [Available input types and defaults for each column type](#available-input-types-and-defaults-for-each-column-type)
- [Custom inputs](#custom-inputs)
- [Custom form builder](#custom-form-builder)
- [I18n](#i18n)
- [Configuration](#configuration)
- [The wrappers API](#the-wrappers-api)
- [Custom Components](#custom-components)
- [HTML 5 Notice](#html-5-notice)
- [Using non Active Record objects](#using-non-active-record-objects)
- [Information](#information)
- [RDocs](#rdocs)
- [Supported Ruby / Rails versions](#supported-ruby--rails-versions)
- [Bug reports](#bug-reports)
- [Maintainers](#maintainers)
- [License](#license)
## Installation
Add it to your Gemfile:
```ruby
gem 'simple_form'
```
Run the following command to install it:
```console
bundle install
```
Run the generator:
```console
rails generate simple_form:install
```
### Bootstrap 5
**Simple Form** can be easily integrated with [Bootstrap 5](http://getbootstrap.com/).
Use the `bootstrap` option in the install generator, like this:
```console
rails generate simple_form:install --bootstrap
```
This will add an initializer that configures **Simple Form** wrappers for
Bootstrap 5's [form controls](https://getbootstrap.com/docs/5.0/forms/overview/).
You have to be sure that you added a copy of the [Bootstrap](http://getbootstrap.com/)
assets on your application.
For more information see the generator output, our
[example application code](https://github.com/heartcombo/simple_form-bootstrap) and
[the live example app](https://simple-form-bootstrap.herokuapp.com/).
### Zurb Foundation 5
To generate wrappers that are compatible with [Zurb Foundation 5](http://foundation.zurb.com/), pass
the `foundation` option to the generator, like this:
```console
rails generate simple_form:install --foundation
```
Please note that the Foundation wrapper does not support the `:hint` option by default. In order to
enable hints, please uncomment the appropriate line in `config/initializers/simple_form_foundation.rb`.
You will need to provide your own CSS styles for hints.
Please see the [instructions on how to install Foundation in a Rails app](http://foundation.zurb.com/docs/applications.html).
### Country Select
If you want to use the country select, you will need the
[country_select gem](https://rubygems.org/gems/country_select), add it to your Gemfile:
```ruby
gem 'country_select'
```
If you don't want to use the gem you can easily override this behaviour by mapping the
country inputs to something else, with a line like this in your `simple_form.rb` initializer:
```ruby
config.input_mappings = { /country/ => :string }
```
## Usage
**Simple Form** was designed to be customized as you need to. Basically it's a stack of components that
are invoked to create a complete html input for you, which by default contains label, hints, errors
and the input itself. It does not aim to create a lot of different logic from the default Rails
form helpers, as they do a great job by themselves. Instead, **Simple Form** acts as a DSL and just
maps your input type (retrieved from the column definition in the database) to a specific helper method.
To start using **Simple Form** you just have to use the helper it provides:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username %>
<%= f.input :password %>
<%= f.button :submit %>
<% end %>
```
This will generate an entire form with labels for user name and password as well, and render errors
by default when you render the form with invalid data (after submitting for example).
You can overwrite the default label by passing it to the input method. You can also add a hint,
an error, or even a placeholder. For boolean inputs, you can add an inline label as well:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username, label: 'Your username please', error: 'Username is mandatory, please specify one' %>
<%= f.input :password, hint: 'No special characters.' %>
<%= f.input :email, placeholder: 'user@domain.com' %>
<%= f.input :remember_me, inline_label: 'Yes, remember me' %>
<%= f.button :submit %>
<% end %>
```
In some cases you may want to disable labels, hints or errors. Or you may want to configure the html
of any of them:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username, label_html: { class: 'my_class' }, hint_html: { class: 'hint_class' } %>
<%= f.input :password, hint: false, error_html: { id: 'password_error' } %>
<%= f.input :password_confirmation, label: false %>
<%= f.button :submit %>
<% end %>
```
It is also possible to pass any html attribute straight to the input, by using the `:input_html`
option, for instance:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username, input_html: { class: 'special' } %>
<%= f.input :password, input_html: { maxlength: 20 } %>
<%= f.input :remember_me, input_html: { value: '1' } %>
<%= f.button :submit %>
<% end %>
```
If you want to pass the same options to all inputs in the form (for example, a default class),
you can use the `:defaults` option in `simple_form_for`. Specific options in `input` call will
overwrite the defaults:
```erb
<%= simple_form_for @user, defaults: { input_html: { class: 'default_class' } } do |f| %>
<%= f.input :username, input_html: { class: 'special' } %>
<%= f.input :password, input_html: { maxlength: 20 } %>
<%= f.input :remember_me, input_html: { value: '1' } %>
<%= f.button :submit %>
<% end %>
```
Since **Simple Form** generates a wrapper div around your label and input by default, you can pass
any html attribute to that wrapper as well using the `:wrapper_html` option, like so:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username, wrapper_html: { class: 'username' } %>
<%= f.input :password, wrapper_html: { id: 'password' } %>
<%= f.input :remember_me, wrapper_html: { class: 'options' } %>
<%= f.button :submit %>
<% end %>
```
Required fields are marked with an * prepended to their labels.
By default all inputs are required. When the form object includes `ActiveModel::Validations`
(which, for example, happens with Active Record models), fields are required only when there is `presence` validation.
Otherwise, **Simple Form** will mark fields as optional. For performance reasons, this
detection is skipped on validations that make use of conditional options, such as `:if` and `:unless`.
And of course, the `required` property of any input can be overwritten as needed:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :name, required: false %>
<%= f.input :username %>
<%= f.input :password %>
<%= f.button :submit %>
<% end %>
```
By default, **Simple Form** will look at the column type in the database and use an
appropriate input for the column. For example, a column created with type
`:text` in the database will use a `textarea` input by default. See the section
[Available input types and defaults for each column
type](https://github.com/heartcombo/simple_form#available-input-types-and-defaults-for-each-column-type)
for a complete list of defaults.
**Simple Form** also lets you overwrite the default input type it creates:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username %>
<%= f.input :password %>
<%= f.input :description, as: :text %>
<%= f.input :accepts, as: :radio_buttons %>
<%= f.button :submit %>
<% end %>
```
So instead of a checkbox for the *accepts* attribute, you'll have a pair of radio buttons with yes/no
labels and a textarea instead of a text field for the description. You can also render boolean
attributes using `as: :select` to show a dropdown.
It is also possible to give the `:disabled` option to **Simple Form**, and it'll automatically mark
the wrapper as disabled with a CSS class, so you can style labels, hints and other components inside
the wrapper as well:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :username, disabled: true, hint: 'You cannot change your username.' %>
<%= f.button :submit %>
<% end %>
```
**Simple Form** inputs accept the same options as their corresponding input type helper in Rails:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :date_of_birth, as: :date, start_year: Date.today.year - 90,
end_year: Date.today.year - 12, discard_day: true,
order: [:month, :year] %>
<%= f.input :accepts, as: :boolean, checked_value: 'positive', unchecked_value: 'negative' %>
<%= f.button :submit %>
<% end %>
```
By default, **Simple Form** generates a hidden field to handle the un-checked case for boolean fields.
Passing `unchecked_value: false` in the options for boolean fields will cause this hidden field to be omitted,
following the convention in Rails. You can also specify `include_hidden: false` to skip the hidden field:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :just_the_checked_case, as: :boolean, include_hidden: false %>
<%= f.button :submit %>
<% end %>
```
**Simple Form** also allows you to use label, hint, input_field, error and full_error helpers
(please take a look at the rdocs for each method for more info):
```erb
<%= simple_form_for @user do |f| %>
<%= f.label :username %>
<%= f.input_field :username %>
<%= f.hint 'No special characters, please!' %>
<%= f.error :username, id: 'user_name_error' %>
<%= f.full_error :token %>
<%= f.submit 'Save' %>
<% end %>
```
Any extra option passed to these methods will be rendered as html option.
### Stripping away all wrapper divs
**Simple Form** also allows you to strip away all the div wrappers around the `` field that is
generated with the usual `f.input`.
The easiest way to achieve this is to use `f.input_field`.
Example:
```ruby
simple_form_for @user do |f|
f.input_field :name
f.input_field :remember_me, as: :boolean
end
```
```html
```
For check boxes and radio buttons you can remove the label changing `boolean_style` from default value `:nested` to `:inline`.
Example:
```ruby
simple_form_for @user do |f|
f.input_field :name
f.input_field :remember_me, as: :boolean, boolean_style: :inline
end
```
```html
```
To view the actual RDocs for this, check them out here - http://rubydoc.info/github/heartcombo/simple_form/main/SimpleForm/FormBuilder:input_field
### Collections
And what if you want to create a select containing the age from 18 to 60 in your form? You can do it
overriding the `:collection` option:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :user %>
<%= f.input :age, collection: 18..60 %>
<%= f.button :submit %>
<% end %>
```
Collections can be arrays or ranges, and when a `:collection` is given the `:select` input will be
rendered by default, so we don't need to pass the `as: :select` option. Other types of collection
are `:radio_buttons` and `:check_boxes`. Those are added by **Simple Form** to Rails set of form
helpers (read Extra Helpers section below for more information).
Collection inputs accept two other options beside collections:
* *label_method* => the label method to be applied to the collection to retrieve the label (use this
instead of the `text_method` option in `collection_select`)
* *value_method* => the value method to be applied to the collection to retrieve the value
Those methods are useful to manipulate the given collection. Both of these options also accept
lambda/procs in case you want to calculate the value or label in a special way eg. custom
translation. You can also define a `to_label` method on your model as **Simple Form** will search for
and use `:to_label` as a `:label_method` first if it is found.
By default, **Simple Form** will use the first item from an array as the label and the second one as the value.
If you want to change this behavior you must make it explicit, like this:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :gender, as: :radio_buttons, collection: [['0', 'female'], ['1', 'male']], label_method: :second, value_method: :first %>
<% end %>
```
All other options given are sent straight to the underlying Rails helper(s): [`collection_select`](http://api.rubyonrails.org/classes/ActionView/Helpers/FormOptionsHelper.html#method-i-collection_select), [`collection_check_boxes`](http://api.rubyonrails.org/classes/ActionView/Helpers/FormOptionsHelper.html#method-i-collection_check_boxes), [`collection_radio_buttons`](http://api.rubyonrails.org/classes/ActionView/Helpers/FormOptionsHelper.html#method-i-collection_radio_buttons). For example, you can pass `prompt` and `selected` as:
```ruby
f.input :age, collection: 18..60, prompt: "Select your age", selected: 21
```
It may also be useful to explicitly pass a value to the optional `:selected` like above, especially if passing a collection of nested objects.
It is also possible to create grouped collection selects, that will use the html *optgroup* tags, like this:
```ruby
f.input :country_id, collection: @continents, as: :grouped_select, group_method: :countries
```
Grouped collection inputs accept the same `:label_method` and `:value_method` options, which will be
used to retrieve label/value attributes for the `option` tags. Besides that, you can give:
* *group_method* => the method to be called on the given collection to generate the options for
each group (required)
* *group_label_method* => the label method to be applied on the given collection to retrieve the label
for the _optgroup_ (**Simple Form** will attempt to guess the best one the same way it does with
`:label_method`)
### Priority
**Simple Form** also supports `:time_zone` and `:country`. When using such helpers, you can give
`:priority` as an option to select which time zones and/or countries should be given higher priority:
```ruby
f.input :residence_country, priority: [ "Brazil" ]
f.input :time_zone, priority: /US/
```
Those values can also be configured with a default value to be used on the site through the
`SimpleForm.country_priority` and `SimpleForm.time_zone_priority` helpers.
Note: While using `country_select` if you want to restrict to only a subset of countries for a specific
drop down then you may use the `:collection` option:
```ruby
f.input :shipping_country, priority: [ "Brazil" ], collection: [ "Australia", "Brazil", "New Zealand"]
```
### Associations
To deal with associations, **Simple Form** can generate select inputs, a series of radios buttons or checkboxes.
Lets see how it works: imagine you have a user model that belongs to a company and `has_and_belongs_to_many`
roles. The structure would be something like:
```ruby
class User < ActiveRecord::Base
belongs_to :company
has_and_belongs_to_many :roles
end
class Company < ActiveRecord::Base
has_many :users
end
class Role < ActiveRecord::Base
has_and_belongs_to_many :users
end
```
Now we have the user form:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :name %>
<%= f.association :company %>
<%= f.association :roles %>
<%= f.button :submit %>
<% end %>
```
Simple enough, right? This is going to render a `:select` input for choosing the `:company`, and another
`:select` input with `:multiple` option for the `:roles`. You can, of course, change it to use radio
buttons and checkboxes as well:
```ruby
f.association :company, as: :radio_buttons
f.association :roles, as: :check_boxes
```
The association helper just invokes `input` under the hood, so all options available to `:select`,
`:radio_buttons` and `:check_boxes` are also available to association. Additionally, you can specify
the collection by hand, all together with the prompt:
```ruby
f.association :company, collection: Company.active.order(:name), prompt: "Choose a Company"
```
In case you want to declare different labels and values:
```ruby
f.association :company, label_method: :company_name, value_method: :id, include_blank: false
```
Please note that the association helper is currently only tested with Active Record. It currently
does not work well with Mongoid and depending on the ORM you're using your mileage may vary.
### Buttons
All web forms need buttons, right? **Simple Form** wraps them in the DSL, acting like a proxy:
```erb
<%= simple_form_for @user do |f| %>
<%= f.input :name %>
<%= f.button :submit %>
<% end %>
```
The above will simply call submit. You choose to use it or not, it's just a question of taste.
The button method also accepts optional parameters, that are delegated to the underlying submit call:
```erb
<%= f.button :submit, "Custom Button Text", class: "my-button" %>
```
To create a `