mirror of
https://github.com/jashkenas/coffeescript.git
synced 2022-11-09 12:23:24 -05:00
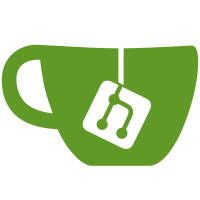
* Make `addLocationDataFn` more DRY * Style fixes * Provide access to full parser inside our custom function running in parser.js; rename the function to lay the groundwork for adding data aside from location data * Fix style. * Fix style. * Label test comments * Update grammar to remove comment tokens; update DSL to call new helper function that preserves comments through parsing * New implementation of compiling block comments: the lexer pulls them out of the token stream, attaching them as a property to a token; the rewriter moves the attachment around so it lives on a token that is destined to make it through to compilation (and in a good placement); and the nodes render the block comment. All tests but one pass (commented out). * If a comment follows a class declaration, move the comment inside the class body * Style * Improve indentation of multiline comments * Fix indentation for block comments, at least in the cases covered by the one failing test * Don’t reverse the order of unshifted comments * Simplify rewriter’s handling of comments, generalizing the special case * Expand the list of tokens we need to avoid for passing comments through the parser; get some literal tokens to have nodes created for them so that the comments pass through * Improve comments; fix multiline flag * Prepare HereComments for processing line comments * Line comments, first draft: the tests pass, but the line comments aren’t indented and sometimes trail previous lines when they shouldn’t; updated compiler output in following commit * Updated compiler, now with line comments * `process` doesn’t exist in the browser, so we should check for its existence first * Update parser output * Test that proves #4290 is fixed * Indent line comments, first pass * Compiled output with indented line comments * Comments that start a new line shouldn’t trail; don’t skip comments attached to generated tokens; stop looking for indentation once we hit a newline * Revised output * Cleanup * Split “multiline” line comment tokens, shifting them forward or back as appropriate * Fix comments in module specifiers * Abstract attaching comments to a node * Line comments in interpolated strings * Line comments can’t be multiline anymore * Improve handling of blank lines and indentation of following comments that start a new line (i.e. don’t trail) * Make comments compilation more object-oriented * Remove lots of dead code that we don’t need anymore because a comment is never a node, only a fragment * Improve eqJS helper * Fix #4290 definitively, with improved output for arrays with interspersed block comments * Add support for line comments output interspersed within arrays * Fix mistake, don’t lose the variable we’re working on * Remove redundant replacements * Check for indentation only from the start of the string * Indentations in generated JS are always multiples of two spaces (never tabs) so just look for 2+ spaces * Update package versions; run Babel twice, once for each preset, temporarily until a Babili bug is fixed that prevents it from running with the env preset * Don’t rely on `fragment.type`, which can break when the compiler is minified * Updated generated docs and browser compiler * Output block comments after function arguments * Comments appear above scope `var` declarations; better tracking of generated `JS` tokens created only to shepherd comments through to the output * Create new FuncGlyph node, to hold comments we want to output near the function parameters * Block comments between `)` and `->`/`=>` get output between `)` and `{`. * Fix indentation of comments that are the first line inside a bare mode block * Updated output * Full Flow example * Updated browser compiler * Abstract and organize comment fragment generation code; store more properties on the comment fragment objects; make `throw` behave like `return` * Abstract token insertion code * Add missing locationData to STRING_START token, giving it the locationData of the overall StringWithInterpolations token so that comments attached to STRING_START end up on the StringWithInterpolations node * Allow `SUPER` tokens to carry comments * Rescue comments from `Existence` nodes and `If` nodes’ conditions * Rescue comments after `\` line continuation tokens * Updated compiled output * Updated browser compiler * Output block comments in the same `compileFragments` method as line comments, except for inline block comments * Comments before splice * Updated browser compiler * Track compiledComments as a property of Base, to ensure that it’s not a global variable * Docs: split up the Usage section * Docs for type annotations via Flow; updated docs output * Update regular comments documentation * Updated browser compiler * Comments before soak * Comments before static methods, and probably before `@variable =` (this) assignments generally * Comments before ‘if exists?’, refactor comment before ‘if this.var’ to be more precise, improve helper methods * Comments before a method that contains ‘super()’ should output above the method property, not above the ‘super.method()’ call * Fix missing comments before `if not` (i.e. before a UNARY token) * Fix comments before ‘for’; add test for comment before assignment if (fixed in earlier commit) * Comments within heregexes * Updated browser compiler * Update description to reflect what’s now happening in compileCommentFragments * Preserve blank lines between line comments; output “whitespace-only” line comments as blank lines, rather than `//` following by whitespace * Better future-proof comments tests * Comments before object destructuring; abstract method for setting comments aside before compilation * Handle more cases of comments before or after `for` loop declaration lines * Fix indentation of comments preceding `for` loops * Fix comment before splat function parameter * Catch another RegexWithInterpolations comment edge case * Updated browser compiler * Change heregex example to one that’s more readable; update output * Remove a few last references to the defunct HERECOMMENT token * Abstract location hash creation into a function * Improved clarity per code review notes * Updated browser compiler
70 lines
2.3 KiB
CoffeeScript
70 lines
2.3 KiB
CoffeeScript
# JavaScript Literals
|
||
# -------------------
|
||
|
||
test "inline JavaScript is evaluated", ->
|
||
eq '\\`', `
|
||
// Inline JS
|
||
"\\\\\`"
|
||
`
|
||
|
||
test "escaped backticks are output correctly", ->
|
||
`var a = \`2 + 2 = ${4}\``
|
||
eq a, '2 + 2 = 4'
|
||
|
||
test "backslashes before a newline don’t break JavaScript blocks", ->
|
||
`var a = \`To be, or not\\
|
||
to be.\``
|
||
eq a, '''
|
||
To be, or not\\
|
||
to be.'''
|
||
|
||
test "block inline JavaScript is evaluated", ->
|
||
```
|
||
var a = 1;
|
||
var b = 2;
|
||
```
|
||
c = 3
|
||
```var d = 4;```
|
||
eq a + b + c + d, 10
|
||
|
||
test "block inline JavaScript containing backticks", ->
|
||
```
|
||
// This is a comment with `backticks`
|
||
var a = 42;
|
||
var b = `foo ${'bar'}`;
|
||
var c = 3;
|
||
var d = 'foo`bar`';
|
||
```
|
||
eq a + c, 45
|
||
eq b, 'foo bar'
|
||
eq d, 'foo`bar`'
|
||
|
||
test "block JavaScript can end with an escaped backtick character", ->
|
||
```var a = \`hello\````
|
||
```
|
||
var b = \`world${'!'}\````
|
||
eq a, 'hello'
|
||
eq b, 'world!'
|
||
|
||
test "JavaScript block only escapes backslashes followed by backticks", ->
|
||
eq `'\\\n'`, '\\\n'
|
||
|
||
test "escaped JavaScript blocks speed round", ->
|
||
# The following has escaped backslashes because they’re required in strings, but the intent is this:
|
||
# `hello` → hello;
|
||
# `\`hello\`` → `hello`;
|
||
# `\`Escaping backticks in JS: \\\`hello\\\`\`` → `Escaping backticks in JS: \`hello\``;
|
||
# `Single backslash: \ ` → Single backslash: \ ;
|
||
# `Double backslash: \\ ` → Double backslash: \\ ;
|
||
# `Single backslash at EOS: \\` → Single backslash at EOS: \;
|
||
# `Double backslash at EOS: \\\\` → Double backslash at EOS: \\;
|
||
for [input, output] in [
|
||
['`hello`', 'hello;']
|
||
['`\\`hello\\``', '`hello`;']
|
||
['`\\`Escaping backticks in JS: \\\\\\`hello\\\\\\`\\``', '`Escaping backticks in JS: \\`hello\\``;']
|
||
['`Single backslash: \\ `', 'Single backslash: \\ ;']
|
||
['`Double backslash: \\\\ `', 'Double backslash: \\\\ ;']
|
||
['`Single backslash at EOS: \\\\`', 'Single backslash at EOS: \\;']
|
||
['`Double backslash at EOS: \\\\\\\\`', 'Double backslash at EOS: \\\\;']
|
||
]
|
||
eqJS input, output
|