mirror of
https://github.com/jashkenas/coffeescript.git
synced 2022-11-09 12:23:24 -05:00
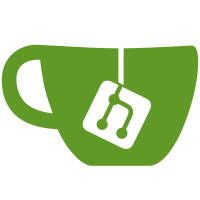
* Don’t confuse the syntax highlighter * Comment Assign::compilePatternMatch a bit * Assignment expressions in conditionals are a bad practice * Rename `wrapInBraces` to `wrapInParentheses`, to set the stage for future `wrapInBraces` that uses `{` and `wrapInBrackets` that uses `[` * Correct comment * object destructuring * Allow custom position of the rest element. * Output simple array destructuring assignments to ES2015 * Output simple object destructured assignments to ES2015 * Compile shorthand object properties to ES2015 shorthand properties This dramatically improves the appearance of destructured imports. * Don’t confuse the syntax highlighter * Comment Assign::compilePatternMatch a bit * Assignment expressions in conditionals are a bad practice * Rename `wrapInBraces` to `wrapInParentheses`, to set the stage for future `wrapInBraces` that uses `{` and `wrapInBrackets` that uses `[` * object destructuring * Allow custom position of the rest element. * rest element in object destructuring * rest element in object destructuring * fix string interpolation * merging * fixing splats in object literal * Rest element in parameter destructuring * merging with CS2 * merged with CS2 * Add support for the object spread initializer. https://github.com/tc39/proposal-object-rest-spread/blob/master/Spread.md * Fix misspellings, trailing whitespace, other minor details * merging with beta2 * refactor object spread properties * small fix * - Fixed object spread function parameters. - Clean up "Assign" and moved all logic for object rest properties in single method (compileObjectDestruct). - Add helper function "objectWithoutKeys" to the "UTILITIES" for use with object rest properties, e.g. {a, b, r...} = obj => {a, b} = obj, r = objectWithoutKeys(...) - Clean up "Obj" and moved all logic for object spread properties in single method (compileSpread). - Clean up "Code". - Add method "hasSplat" to "Obj" and "Value" for checking if Obj contains the splat. - Enable placing spread syntax triple dots on either right or left, per #85 (https://github.com/coffeescript6/discuss/issues/85) * Fixed typos * Remove unused code * Removed dots (e.g. splat) on the left side from the grammar * Initial release for deep spread properties, e.g. obj2 = {obj.b..., a: 1} or {obj[b][c]..., d: 7} Tests need to be prepared! * 1. Object literal spread properties Object literals: - obj = { {b:{c:{d:1}}}..., a:1 } Parenthetical: - obj = { ( body ), a:1 } - obj = { ( body )..., a:1 } Invocation: - obj = { ( (args) -> ... )(params), a:1 } - obj = { ( (args) -> ... )(params)..., a:1 } - obj = { foo(), a:1 } - obj = { foo()..., a:1 } 2. Refactor, cleanup & other optimizations. * Merged with 2.0 * Cleanup * Some more cleanup. * Fixed error with freeVariable and object destructuring. * Fixed errors with object spread properties. * Improvements, fixed errors. * Minor improvement. * Minor improvements. * Typo. * Remove unnecessary whitespace. * Remove unnecessary whitespace. * Changed few "assertErrorFormat" tests since parentheses are now allowed in the Obj. * Whitespace cleanup * Comments cleanup * fix destructured obj param declarations * refine fix; add test * Refactor function args ({a, b...}) * Additional tests for object destructuring in function argument. * Minor improvement for object destructuring variable declaration. * refactor function args ({a, b...}) and ({a, b...} = {}); Obj And Param cleanup * fix comment * Fix object destructuring variable declaration. * more tests with default values * fix typo * Fixed default values in object destructuring. * small fix * Babel’s tests for object rest spread * Style: spaces after colons in object declarations * Cleanup comments * Simplify Babel tests * Fix comments * Fix destructuring with splats in multiple objects * Add test for default values in detsructuring assignment with splats * Handle default values when assigning to object splats * Rewrite traverseRest to fix handling of dynamic keys * Fix double parens around destructuring with splats * Update compileObjectDestruct comments * Improve formatting of top-level destructures with splats and tidy parens * Added a bigger destructuring-with-defaults test and fixed a bug * Refactor destructuring grammar to allow additional forms * Add a missing case to ObjSpreadExpr * These tests shouldn’t run in the browser * Fix test.html * Fix docs scroll position getting screwed up by CodeMirror initialization * Breaking change documentation about => (fixes #4593) * Spread/rest syntax documentation * Documentation about bound class methods * 2.0.0-beta3 changelog * Add note about ‘lib’ * Fix accidentally converting this to tabs * Bump version to 2.0.0-beta3 * Update annotated source and test.html
601 lines
18 KiB
JavaScript
601 lines
18 KiB
JavaScript
// Generated by CoffeeScript 2.0.0-beta3
|
|
(function() {
|
|
var BANNER, CoffeeScript, EventEmitter, SWITCHES, buildCSOptionParser, compileJoin, compileOptions, compilePath, compileScript, compileStdio, exec, findDirectoryIndex, forkNode, fs, helpers, hidden, joinTimeout, makePrelude, mkdirp, notSources, optionParser, optparse, opts, outputPath, parseOptions, path, printLine, printTokens, printWarn, removeSource, removeSourceDir, silentUnlink, sourceCode, sources, spawn, timeLog, usage, useWinPathSep, version, wait, watch, watchDir, watchedDirs, writeJs,
|
|
indexOf = [].indexOf;
|
|
|
|
fs = require('fs');
|
|
|
|
path = require('path');
|
|
|
|
helpers = require('./helpers');
|
|
|
|
optparse = require('./optparse');
|
|
|
|
CoffeeScript = require('./');
|
|
|
|
({spawn, exec} = require('child_process'));
|
|
|
|
({EventEmitter} = require('events'));
|
|
|
|
useWinPathSep = path.sep === '\\';
|
|
|
|
helpers.extend(CoffeeScript, new EventEmitter);
|
|
|
|
printLine = function(line) {
|
|
return process.stdout.write(line + '\n');
|
|
};
|
|
|
|
printWarn = function(line) {
|
|
return process.stderr.write(line + '\n');
|
|
};
|
|
|
|
hidden = function(file) {
|
|
return /^\.|~$/.test(file);
|
|
};
|
|
|
|
BANNER = 'Usage: coffee [options] path/to/script.coffee -- [args]\n\nIf called without options, `coffee` will run your script.';
|
|
|
|
SWITCHES = [['-b', '--bare', 'compile without a top-level function wrapper'], ['-c', '--compile', 'compile to JavaScript and save as .js files'], ['-e', '--eval', 'pass a string from the command line as input'], ['-h', '--help', 'display this help message'], ['-i', '--interactive', 'run an interactive CoffeeScript REPL'], ['-j', '--join [FILE]', 'concatenate the source CoffeeScript before compiling'], ['-m', '--map', 'generate source map and save as .js.map files'], ['-M', '--inline-map', 'generate source map and include it directly in output'], ['-n', '--nodes', 'print out the parse tree that the parser produces'], ['--nodejs [ARGS]', 'pass options directly to the "node" binary'], ['--no-header', 'suppress the "Generated by" header'], ['-o', '--output [DIR]', 'set the output directory for compiled JavaScript'], ['-p', '--print', 'print out the compiled JavaScript'], ['-r', '--require [MODULE*]', 'require the given module before eval or REPL'], ['-s', '--stdio', 'listen for and compile scripts over stdio'], ['-l', '--literate', 'treat stdio as literate style coffeescript'], ['-t', '--tokens', 'print out the tokens that the lexer/rewriter produce'], ['-v', '--version', 'display the version number'], ['-w', '--watch', 'watch scripts for changes and rerun commands']];
|
|
|
|
opts = {};
|
|
|
|
sources = [];
|
|
|
|
sourceCode = [];
|
|
|
|
notSources = {};
|
|
|
|
watchedDirs = {};
|
|
|
|
optionParser = null;
|
|
|
|
exports.buildCSOptionParser = buildCSOptionParser = function() {
|
|
return new optparse.OptionParser(SWITCHES, BANNER);
|
|
};
|
|
|
|
exports.run = function() {
|
|
var i, len, literals, ref, replCliOpts, results, source;
|
|
optionParser = buildCSOptionParser();
|
|
parseOptions();
|
|
replCliOpts = {
|
|
useGlobal: true
|
|
};
|
|
if (opts.require) {
|
|
opts.prelude = makePrelude(opts.require);
|
|
}
|
|
replCliOpts.prelude = opts.prelude;
|
|
if (opts.nodejs) {
|
|
return forkNode();
|
|
}
|
|
if (opts.help) {
|
|
return usage();
|
|
}
|
|
if (opts.version) {
|
|
return version();
|
|
}
|
|
if (opts.interactive) {
|
|
return require('./repl').start(replCliOpts);
|
|
}
|
|
if (opts.stdio) {
|
|
return compileStdio();
|
|
}
|
|
if (opts.eval) {
|
|
return compileScript(null, opts.arguments[0]);
|
|
}
|
|
if (!opts.arguments.length) {
|
|
return require('./repl').start(replCliOpts);
|
|
}
|
|
literals = opts.run ? opts.arguments.splice(1) : [];
|
|
process.argv = process.argv.slice(0, 2).concat(literals);
|
|
process.argv[0] = 'coffee';
|
|
if (opts.output) {
|
|
opts.output = path.resolve(opts.output);
|
|
}
|
|
if (opts.join) {
|
|
opts.join = path.resolve(opts.join);
|
|
console.error('\nThe --join option is deprecated and will be removed in a future version.\n\nIf for some reason it\'s necessary to share local variables between files,\nreplace...\n\n $ coffee --compile --join bundle.js -- a.coffee b.coffee c.coffee\n\nwith...\n\n $ cat a.coffee b.coffee c.coffee | coffee --compile --stdio > bundle.js\n');
|
|
}
|
|
ref = opts.arguments;
|
|
results = [];
|
|
for (i = 0, len = ref.length; i < len; i++) {
|
|
source = ref[i];
|
|
source = path.resolve(source);
|
|
results.push(compilePath(source, true, source));
|
|
}
|
|
return results;
|
|
};
|
|
|
|
makePrelude = function(requires) {
|
|
return requires.map(function(module) {
|
|
var _, match, name;
|
|
if (match = module.match(/^(.*)=(.*)$/)) {
|
|
[_, name, module] = match;
|
|
}
|
|
name || (name = helpers.baseFileName(module, true, useWinPathSep));
|
|
return `${name} = require('${module}')`;
|
|
}).join(';');
|
|
};
|
|
|
|
compilePath = function(source, topLevel, base) {
|
|
var code, err, file, files, i, len, results, stats;
|
|
if (indexOf.call(sources, source) >= 0 || watchedDirs[source] || !topLevel && (notSources[source] || hidden(source))) {
|
|
return;
|
|
}
|
|
try {
|
|
stats = fs.statSync(source);
|
|
} catch (error) {
|
|
err = error;
|
|
if (err.code === 'ENOENT') {
|
|
console.error(`File not found: ${source}`);
|
|
process.exit(1);
|
|
}
|
|
throw err;
|
|
}
|
|
if (stats.isDirectory()) {
|
|
if (path.basename(source) === 'node_modules') {
|
|
notSources[source] = true;
|
|
return;
|
|
}
|
|
if (opts.run) {
|
|
compilePath(findDirectoryIndex(source), topLevel, base);
|
|
return;
|
|
}
|
|
if (opts.watch) {
|
|
watchDir(source, base);
|
|
}
|
|
try {
|
|
files = fs.readdirSync(source);
|
|
} catch (error) {
|
|
err = error;
|
|
if (err.code === 'ENOENT') {
|
|
return;
|
|
} else {
|
|
throw err;
|
|
}
|
|
}
|
|
results = [];
|
|
for (i = 0, len = files.length; i < len; i++) {
|
|
file = files[i];
|
|
results.push(compilePath(path.join(source, file), false, base));
|
|
}
|
|
return results;
|
|
} else if (topLevel || helpers.isCoffee(source)) {
|
|
sources.push(source);
|
|
sourceCode.push(null);
|
|
delete notSources[source];
|
|
if (opts.watch) {
|
|
watch(source, base);
|
|
}
|
|
try {
|
|
code = fs.readFileSync(source);
|
|
} catch (error) {
|
|
err = error;
|
|
if (err.code === 'ENOENT') {
|
|
return;
|
|
} else {
|
|
throw err;
|
|
}
|
|
}
|
|
return compileScript(source, code.toString(), base);
|
|
} else {
|
|
return notSources[source] = true;
|
|
}
|
|
};
|
|
|
|
findDirectoryIndex = function(source) {
|
|
var err, ext, i, index, len, ref;
|
|
ref = CoffeeScript.FILE_EXTENSIONS;
|
|
for (i = 0, len = ref.length; i < len; i++) {
|
|
ext = ref[i];
|
|
index = path.join(source, `index${ext}`);
|
|
try {
|
|
if ((fs.statSync(index)).isFile()) {
|
|
return index;
|
|
}
|
|
} catch (error) {
|
|
err = error;
|
|
if (err.code !== 'ENOENT') {
|
|
throw err;
|
|
}
|
|
}
|
|
}
|
|
console.error(`Missing index.coffee or index.litcoffee in ${source}`);
|
|
return process.exit(1);
|
|
};
|
|
|
|
compileScript = function(file, input, base = null) {
|
|
var compiled, err, message, o, options, t, task;
|
|
o = opts;
|
|
options = compileOptions(file, base);
|
|
try {
|
|
t = task = {file, input, options};
|
|
CoffeeScript.emit('compile', task);
|
|
if (o.tokens) {
|
|
return printTokens(CoffeeScript.tokens(t.input, t.options));
|
|
} else if (o.nodes) {
|
|
return printLine(CoffeeScript.nodes(t.input, t.options).toString().trim());
|
|
} else if (o.run) {
|
|
CoffeeScript.register();
|
|
if (opts.prelude) {
|
|
CoffeeScript.eval(opts.prelude, t.options);
|
|
}
|
|
return CoffeeScript.run(t.input, t.options);
|
|
} else if (o.join && t.file !== o.join) {
|
|
if (helpers.isLiterate(file)) {
|
|
t.input = helpers.invertLiterate(t.input);
|
|
}
|
|
sourceCode[sources.indexOf(t.file)] = t.input;
|
|
return compileJoin();
|
|
} else {
|
|
compiled = CoffeeScript.compile(t.input, t.options);
|
|
t.output = compiled;
|
|
if (o.map) {
|
|
t.output = compiled.js;
|
|
t.sourceMap = compiled.v3SourceMap;
|
|
}
|
|
CoffeeScript.emit('success', task);
|
|
if (o.print) {
|
|
return printLine(t.output.trim());
|
|
} else if (o.compile || o.map) {
|
|
return writeJs(base, t.file, t.output, options.jsPath, t.sourceMap);
|
|
}
|
|
}
|
|
} catch (error) {
|
|
err = error;
|
|
CoffeeScript.emit('failure', err, task);
|
|
if (CoffeeScript.listeners('failure').length) {
|
|
return;
|
|
}
|
|
message = (err != null ? err.stack : void 0) || `${err}`;
|
|
if (o.watch) {
|
|
return printLine(message + '\x07');
|
|
} else {
|
|
printWarn(message);
|
|
return process.exit(1);
|
|
}
|
|
}
|
|
};
|
|
|
|
compileStdio = function() {
|
|
var buffers, stdin;
|
|
buffers = [];
|
|
stdin = process.openStdin();
|
|
stdin.on('data', function(buffer) {
|
|
if (buffer) {
|
|
return buffers.push(buffer);
|
|
}
|
|
});
|
|
return stdin.on('end', function() {
|
|
return compileScript(null, Buffer.concat(buffers).toString());
|
|
});
|
|
};
|
|
|
|
joinTimeout = null;
|
|
|
|
compileJoin = function() {
|
|
if (!opts.join) {
|
|
return;
|
|
}
|
|
if (!sourceCode.some(function(code) {
|
|
return code === null;
|
|
})) {
|
|
clearTimeout(joinTimeout);
|
|
return joinTimeout = wait(100, function() {
|
|
return compileScript(opts.join, sourceCode.join('\n'), opts.join);
|
|
});
|
|
}
|
|
};
|
|
|
|
watch = function(source, base) {
|
|
var compile, compileTimeout, err, prevStats, rewatch, startWatcher, watchErr, watcher;
|
|
watcher = null;
|
|
prevStats = null;
|
|
compileTimeout = null;
|
|
watchErr = function(err) {
|
|
if (err.code !== 'ENOENT') {
|
|
throw err;
|
|
}
|
|
if (indexOf.call(sources, source) < 0) {
|
|
return;
|
|
}
|
|
try {
|
|
rewatch();
|
|
return compile();
|
|
} catch (error) {
|
|
removeSource(source, base);
|
|
return compileJoin();
|
|
}
|
|
};
|
|
compile = function() {
|
|
clearTimeout(compileTimeout);
|
|
return compileTimeout = wait(25, function() {
|
|
return fs.stat(source, function(err, stats) {
|
|
if (err) {
|
|
return watchErr(err);
|
|
}
|
|
if (prevStats && stats.size === prevStats.size && stats.mtime.getTime() === prevStats.mtime.getTime()) {
|
|
return rewatch();
|
|
}
|
|
prevStats = stats;
|
|
return fs.readFile(source, function(err, code) {
|
|
if (err) {
|
|
return watchErr(err);
|
|
}
|
|
compileScript(source, code.toString(), base);
|
|
return rewatch();
|
|
});
|
|
});
|
|
});
|
|
};
|
|
startWatcher = function() {
|
|
return watcher = fs.watch(source).on('change', compile).on('error', function(err) {
|
|
if (err.code !== 'EPERM') {
|
|
throw err;
|
|
}
|
|
return removeSource(source, base);
|
|
});
|
|
};
|
|
rewatch = function() {
|
|
if (watcher != null) {
|
|
watcher.close();
|
|
}
|
|
return startWatcher();
|
|
};
|
|
try {
|
|
return startWatcher();
|
|
} catch (error) {
|
|
err = error;
|
|
return watchErr(err);
|
|
}
|
|
};
|
|
|
|
watchDir = function(source, base) {
|
|
var err, readdirTimeout, startWatcher, stopWatcher, watcher;
|
|
watcher = null;
|
|
readdirTimeout = null;
|
|
startWatcher = function() {
|
|
return watcher = fs.watch(source).on('error', function(err) {
|
|
if (err.code !== 'EPERM') {
|
|
throw err;
|
|
}
|
|
return stopWatcher();
|
|
}).on('change', function() {
|
|
clearTimeout(readdirTimeout);
|
|
return readdirTimeout = wait(25, function() {
|
|
var err, file, files, i, len, results;
|
|
try {
|
|
files = fs.readdirSync(source);
|
|
} catch (error) {
|
|
err = error;
|
|
if (err.code !== 'ENOENT') {
|
|
throw err;
|
|
}
|
|
return stopWatcher();
|
|
}
|
|
results = [];
|
|
for (i = 0, len = files.length; i < len; i++) {
|
|
file = files[i];
|
|
results.push(compilePath(path.join(source, file), false, base));
|
|
}
|
|
return results;
|
|
});
|
|
});
|
|
};
|
|
stopWatcher = function() {
|
|
watcher.close();
|
|
return removeSourceDir(source, base);
|
|
};
|
|
watchedDirs[source] = true;
|
|
try {
|
|
return startWatcher();
|
|
} catch (error) {
|
|
err = error;
|
|
if (err.code !== 'ENOENT') {
|
|
throw err;
|
|
}
|
|
}
|
|
};
|
|
|
|
removeSourceDir = function(source, base) {
|
|
var file, i, len, sourcesChanged;
|
|
delete watchedDirs[source];
|
|
sourcesChanged = false;
|
|
for (i = 0, len = sources.length; i < len; i++) {
|
|
file = sources[i];
|
|
if (!(source === path.dirname(file))) {
|
|
continue;
|
|
}
|
|
removeSource(file, base);
|
|
sourcesChanged = true;
|
|
}
|
|
if (sourcesChanged) {
|
|
return compileJoin();
|
|
}
|
|
};
|
|
|
|
removeSource = function(source, base) {
|
|
var index;
|
|
index = sources.indexOf(source);
|
|
sources.splice(index, 1);
|
|
sourceCode.splice(index, 1);
|
|
if (!opts.join) {
|
|
silentUnlink(outputPath(source, base));
|
|
silentUnlink(outputPath(source, base, '.js.map'));
|
|
return timeLog(`removed ${source}`);
|
|
}
|
|
};
|
|
|
|
silentUnlink = function(path) {
|
|
var err, ref;
|
|
try {
|
|
return fs.unlinkSync(path);
|
|
} catch (error) {
|
|
err = error;
|
|
if ((ref = err.code) !== 'ENOENT' && ref !== 'EPERM') {
|
|
throw err;
|
|
}
|
|
}
|
|
};
|
|
|
|
outputPath = function(source, base, extension = ".js") {
|
|
var basename, dir, srcDir;
|
|
basename = helpers.baseFileName(source, true, useWinPathSep);
|
|
srcDir = path.dirname(source);
|
|
if (!opts.output) {
|
|
dir = srcDir;
|
|
} else if (source === base) {
|
|
dir = opts.output;
|
|
} else {
|
|
dir = path.join(opts.output, path.relative(base, srcDir));
|
|
}
|
|
return path.join(dir, basename + extension);
|
|
};
|
|
|
|
mkdirp = function(dir, fn) {
|
|
var mkdirs, mode;
|
|
mode = 0o777 & ~process.umask();
|
|
return (mkdirs = function(p, fn) {
|
|
return fs.exists(p, function(exists) {
|
|
if (exists) {
|
|
return fn();
|
|
} else {
|
|
return mkdirs(path.dirname(p), function() {
|
|
return fs.mkdir(p, mode, function(err) {
|
|
if (err) {
|
|
return fn(err);
|
|
}
|
|
return fn();
|
|
});
|
|
});
|
|
}
|
|
});
|
|
})(dir, fn);
|
|
};
|
|
|
|
writeJs = function(base, sourcePath, js, jsPath, generatedSourceMap = null) {
|
|
var compile, jsDir, sourceMapPath;
|
|
sourceMapPath = outputPath(sourcePath, base, ".js.map");
|
|
jsDir = path.dirname(jsPath);
|
|
compile = function() {
|
|
if (opts.compile) {
|
|
if (js.length <= 0) {
|
|
js = ' ';
|
|
}
|
|
if (generatedSourceMap) {
|
|
js = `${js}\n//# sourceMappingURL=${helpers.baseFileName(sourceMapPath, false, useWinPathSep)}\n`;
|
|
}
|
|
fs.writeFile(jsPath, js, function(err) {
|
|
if (err) {
|
|
printLine(err.message);
|
|
return process.exit(1);
|
|
} else if (opts.compile && opts.watch) {
|
|
return timeLog(`compiled ${sourcePath}`);
|
|
}
|
|
});
|
|
}
|
|
if (generatedSourceMap) {
|
|
return fs.writeFile(sourceMapPath, generatedSourceMap, function(err) {
|
|
if (err) {
|
|
printLine(`Could not write source map: ${err.message}`);
|
|
return process.exit(1);
|
|
}
|
|
});
|
|
}
|
|
};
|
|
return fs.exists(jsDir, function(itExists) {
|
|
if (itExists) {
|
|
return compile();
|
|
} else {
|
|
return mkdirp(jsDir, compile);
|
|
}
|
|
});
|
|
};
|
|
|
|
wait = function(milliseconds, func) {
|
|
return setTimeout(func, milliseconds);
|
|
};
|
|
|
|
timeLog = function(message) {
|
|
return console.log(`${(new Date).toLocaleTimeString()} - ${message}`);
|
|
};
|
|
|
|
printTokens = function(tokens) {
|
|
var strings, tag, token, value;
|
|
strings = (function() {
|
|
var i, len, results;
|
|
results = [];
|
|
for (i = 0, len = tokens.length; i < len; i++) {
|
|
token = tokens[i];
|
|
tag = token[0];
|
|
value = token[1].toString().replace(/\n/, '\\n');
|
|
results.push(`[${tag} ${value}]`);
|
|
}
|
|
return results;
|
|
})();
|
|
return printLine(strings.join(' '));
|
|
};
|
|
|
|
parseOptions = function() {
|
|
var o;
|
|
o = opts = optionParser.parse(process.argv.slice(2));
|
|
o.compile || (o.compile = !!o.output);
|
|
o.run = !(o.compile || o.print || o.map);
|
|
return o.print = !!(o.print || (o.eval || o.stdio && o.compile));
|
|
};
|
|
|
|
compileOptions = function(filename, base) {
|
|
var answer, cwd, jsDir, jsPath;
|
|
answer = {
|
|
filename,
|
|
literate: opts.literate || helpers.isLiterate(filename),
|
|
bare: opts.bare,
|
|
header: opts.compile && !opts['no-header'],
|
|
sourceMap: opts.map,
|
|
inlineMap: opts['inline-map']
|
|
};
|
|
if (filename) {
|
|
if (base) {
|
|
cwd = process.cwd();
|
|
jsPath = outputPath(filename, base);
|
|
jsDir = path.dirname(jsPath);
|
|
answer = helpers.merge(answer, {
|
|
jsPath,
|
|
sourceRoot: path.relative(jsDir, cwd),
|
|
sourceFiles: [path.relative(cwd, filename)],
|
|
generatedFile: helpers.baseFileName(jsPath, false, useWinPathSep)
|
|
});
|
|
} else {
|
|
answer = helpers.merge(answer, {
|
|
sourceRoot: "",
|
|
sourceFiles: [helpers.baseFileName(filename, false, useWinPathSep)],
|
|
generatedFile: helpers.baseFileName(filename, true, useWinPathSep) + ".js"
|
|
});
|
|
}
|
|
}
|
|
return answer;
|
|
};
|
|
|
|
forkNode = function() {
|
|
var args, nodeArgs, p;
|
|
nodeArgs = opts.nodejs.split(/\s+/);
|
|
args = process.argv.slice(1);
|
|
args.splice(args.indexOf('--nodejs'), 2);
|
|
p = spawn(process.execPath, nodeArgs.concat(args), {
|
|
cwd: process.cwd(),
|
|
env: process.env,
|
|
stdio: [0, 1, 2]
|
|
});
|
|
return p.on('exit', function(code) {
|
|
return process.exit(code);
|
|
});
|
|
};
|
|
|
|
usage = function() {
|
|
return printLine(optionParser.help());
|
|
};
|
|
|
|
version = function() {
|
|
return printLine(`CoffeeScript version ${CoffeeScript.VERSION}`);
|
|
};
|
|
|
|
}).call(this);
|