mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
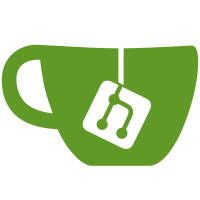
For now docker stats will sum the rxbytes, txbytes, etc. of all the interfaces. It is OK for the output of CLI `docker stats` but not good for the API response, especially when the container is in sereval subnets. It's better to leave these origianl data to user. Signed-off-by: Hu Keping <hukeping@huawei.com>
113 lines
4 KiB
Go
113 lines
4 KiB
Go
// Package types is used for API stability in the types and response to the
|
|
// consumers of the API stats endpoint.
|
|
package types
|
|
|
|
import "time"
|
|
|
|
// ThrottlingData stores CPU throttling stats of one running container
|
|
type ThrottlingData struct {
|
|
// Number of periods with throttling active
|
|
Periods uint64 `json:"periods"`
|
|
// Number of periods when the container hit its throttling limit.
|
|
ThrottledPeriods uint64 `json:"throttled_periods"`
|
|
// Aggregate time the container was throttled for in nanoseconds.
|
|
ThrottledTime uint64 `json:"throttled_time"`
|
|
}
|
|
|
|
// CPUUsage stores All CPU stats aggregated since container inception.
|
|
type CPUUsage struct {
|
|
// Total CPU time consumed.
|
|
// Units: nanoseconds.
|
|
TotalUsage uint64 `json:"total_usage"`
|
|
// Total CPU time consumed per core.
|
|
// Units: nanoseconds.
|
|
PercpuUsage []uint64 `json:"percpu_usage"`
|
|
// Time spent by tasks of the cgroup in kernel mode.
|
|
// Units: nanoseconds.
|
|
UsageInKernelmode uint64 `json:"usage_in_kernelmode"`
|
|
// Time spent by tasks of the cgroup in user mode.
|
|
// Units: nanoseconds.
|
|
UsageInUsermode uint64 `json:"usage_in_usermode"`
|
|
}
|
|
|
|
// CPUStats aggregates and wraps all CPU related info of container
|
|
type CPUStats struct {
|
|
CPUUsage CPUUsage `json:"cpu_usage"`
|
|
SystemUsage uint64 `json:"system_cpu_usage"`
|
|
ThrottlingData ThrottlingData `json:"throttling_data,omitempty"`
|
|
}
|
|
|
|
// MemoryStats aggregates All memory stats since container inception
|
|
type MemoryStats struct {
|
|
// current res_counter usage for memory
|
|
Usage uint64 `json:"usage"`
|
|
// maximum usage ever recorded.
|
|
MaxUsage uint64 `json:"max_usage"`
|
|
// TODO(vishh): Export these as stronger types.
|
|
// all the stats exported via memory.stat.
|
|
Stats map[string]uint64 `json:"stats"`
|
|
// number of times memory usage hits limits.
|
|
Failcnt uint64 `json:"failcnt"`
|
|
Limit uint64 `json:"limit"`
|
|
}
|
|
|
|
// BlkioStatEntry is one small entity to store a piece of Blkio stats
|
|
// TODO Windows: This can be factored out
|
|
type BlkioStatEntry struct {
|
|
Major uint64 `json:"major"`
|
|
Minor uint64 `json:"minor"`
|
|
Op string `json:"op"`
|
|
Value uint64 `json:"value"`
|
|
}
|
|
|
|
// BlkioStats stores All IO service stats for data read and write
|
|
// TODO Windows: This can be factored out
|
|
type BlkioStats struct {
|
|
// number of bytes tranferred to and from the block device
|
|
IoServiceBytesRecursive []BlkioStatEntry `json:"io_service_bytes_recursive"`
|
|
IoServicedRecursive []BlkioStatEntry `json:"io_serviced_recursive"`
|
|
IoQueuedRecursive []BlkioStatEntry `json:"io_queue_recursive"`
|
|
IoServiceTimeRecursive []BlkioStatEntry `json:"io_service_time_recursive"`
|
|
IoWaitTimeRecursive []BlkioStatEntry `json:"io_wait_time_recursive"`
|
|
IoMergedRecursive []BlkioStatEntry `json:"io_merged_recursive"`
|
|
IoTimeRecursive []BlkioStatEntry `json:"io_time_recursive"`
|
|
SectorsRecursive []BlkioStatEntry `json:"sectors_recursive"`
|
|
}
|
|
|
|
// NetworkStats aggregates All network stats of one container
|
|
// TODO Windows: This will require refactoring
|
|
type NetworkStats struct {
|
|
RxBytes uint64 `json:"rx_bytes"`
|
|
RxPackets uint64 `json:"rx_packets"`
|
|
RxErrors uint64 `json:"rx_errors"`
|
|
RxDropped uint64 `json:"rx_dropped"`
|
|
TxBytes uint64 `json:"tx_bytes"`
|
|
TxPackets uint64 `json:"tx_packets"`
|
|
TxErrors uint64 `json:"tx_errors"`
|
|
TxDropped uint64 `json:"tx_dropped"`
|
|
}
|
|
|
|
// Stats is Ultimate struct aggregating all types of stats of one container
|
|
type Stats struct {
|
|
Read time.Time `json:"read"`
|
|
PreCPUStats CPUStats `json:"precpu_stats,omitempty"`
|
|
CPUStats CPUStats `json:"cpu_stats,omitempty"`
|
|
MemoryStats MemoryStats `json:"memory_stats,omitempty"`
|
|
BlkioStats BlkioStats `json:"blkio_stats,omitempty"`
|
|
}
|
|
|
|
// StatsJSONPre121 is a backcompatibility struct along with ContainerConfig
|
|
type StatsJSONPre121 struct {
|
|
Stats
|
|
|
|
// Network is for fallback stats where API Version < 1.21
|
|
Network NetworkStats `json:"network,omitempty"`
|
|
}
|
|
|
|
// StatsJSON is newly used Networks
|
|
type StatsJSON struct {
|
|
Stats
|
|
|
|
// Networks request version >=1.21
|
|
Networks map[string]NetworkStats `json:"networks,omitempty"`
|
|
}
|