mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
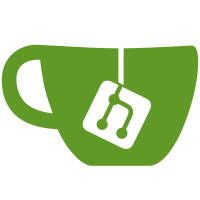
[pkg/archive] Update archive/copy path handling - Remove unused TarOptions.Name field. - Add new TarOptions.RebaseNames field. - Update some of the logic around path dir/base splitting. - Update some of the logic behind archive entry name rebasing. [api/types] Add LinkTarget field to PathStat [daemon] Fix stat, archive, extract of symlinks These operations *should* resolve symlinks that are in the path but if the resource itself is a symlink then it *should not* be resolved. This patch puts this logic into a common function `resolvePath` which resolves symlinks of the path's dir in scope of the container rootfs but does not resolve the final element of the path. Now archive, extract, and stat operations will return symlinks if the path is indeed a symlink. [api/client] Update cp path hanling [docs/reference/api] Update description of stat Add the linkTarget field to the header of the archive endpoint. Remove path field. [integration-cli] Fix/Add cp symlink test cases Copying a symlink should do just that: copy the symlink NOT copy the target of the symlink. Also, the resulting file from the copy should have the name of the symlink NOT the name of the target file. Copying to a symlink should copy to the symlink target and not modify the symlink itself. Docker-DCO-1.1-Signed-off-by: Josh Hawn <josh.hawn@docker.com> (github: jlhawn)
279 lines
6.2 KiB
Go
279 lines
6.2 KiB
Go
package types
|
|
|
|
import (
|
|
"os"
|
|
"time"
|
|
|
|
"github.com/docker/docker/daemon/network"
|
|
"github.com/docker/docker/pkg/version"
|
|
"github.com/docker/docker/runconfig"
|
|
)
|
|
|
|
// ContainerCreateResponse contains the information returned to a client on the
|
|
// creation of a new container.
|
|
type ContainerCreateResponse struct {
|
|
// ID is the ID of the created container.
|
|
ID string `json:"Id"`
|
|
|
|
// Warnings are any warnings encountered during the creation of the container.
|
|
Warnings []string `json:"Warnings"`
|
|
}
|
|
|
|
// POST /containers/{name:.*}/exec
|
|
type ContainerExecCreateResponse struct {
|
|
// ID is the exec ID.
|
|
ID string `json:"Id"`
|
|
}
|
|
|
|
// POST /auth
|
|
type AuthResponse struct {
|
|
// Status is the authentication status
|
|
Status string `json:"Status"`
|
|
}
|
|
|
|
// POST "/containers/"+containerID+"/wait"
|
|
type ContainerWaitResponse struct {
|
|
// StatusCode is the status code of the wait job
|
|
StatusCode int `json:"StatusCode"`
|
|
}
|
|
|
|
// POST "/commit?container="+containerID
|
|
type ContainerCommitResponse struct {
|
|
ID string `json:"Id"`
|
|
}
|
|
|
|
// GET "/containers/{name:.*}/changes"
|
|
type ContainerChange struct {
|
|
Kind int
|
|
Path string
|
|
}
|
|
|
|
// GET "/images/{name:.*}/history"
|
|
type ImageHistory struct {
|
|
ID string `json:"Id"`
|
|
Created int64
|
|
CreatedBy string
|
|
Tags []string
|
|
Size int64
|
|
Comment string
|
|
}
|
|
|
|
// DELETE "/images/{name:.*}"
|
|
type ImageDelete struct {
|
|
Untagged string `json:",omitempty"`
|
|
Deleted string `json:",omitempty"`
|
|
}
|
|
|
|
// GET "/images/json"
|
|
type Image struct {
|
|
ID string `json:"Id"`
|
|
ParentId string
|
|
RepoTags []string
|
|
RepoDigests []string
|
|
Created int
|
|
Size int
|
|
VirtualSize int
|
|
Labels map[string]string
|
|
}
|
|
|
|
type GraphDriverData struct {
|
|
Name string
|
|
Data map[string]string
|
|
}
|
|
|
|
// GET "/images/{name:.*}/json"
|
|
type ImageInspect struct {
|
|
Id string
|
|
Parent string
|
|
Comment string
|
|
Created string
|
|
Container string
|
|
ContainerConfig *runconfig.Config
|
|
DockerVersion string
|
|
Author string
|
|
Config *runconfig.Config
|
|
Architecture string
|
|
Os string
|
|
Size int64
|
|
VirtualSize int64
|
|
GraphDriver GraphDriverData
|
|
}
|
|
|
|
// GET "/containers/json"
|
|
type Port struct {
|
|
IP string `json:",omitempty"`
|
|
PrivatePort int
|
|
PublicPort int `json:",omitempty"`
|
|
Type string
|
|
}
|
|
|
|
type Container struct {
|
|
ID string `json:"Id"`
|
|
Names []string
|
|
Image string
|
|
Command string
|
|
Created int
|
|
Ports []Port
|
|
SizeRw int `json:",omitempty"`
|
|
SizeRootFs int `json:",omitempty"`
|
|
Labels map[string]string
|
|
Status string
|
|
HostConfig struct {
|
|
NetworkMode string `json:",omitempty"`
|
|
}
|
|
}
|
|
|
|
// POST "/containers/"+containerID+"/copy"
|
|
type CopyConfig struct {
|
|
Resource string
|
|
}
|
|
|
|
// ContainerPathStat is used to encode the header from
|
|
// GET /containers/{name:.*}/archive
|
|
// "name" is basename of the resource.
|
|
type ContainerPathStat struct {
|
|
Name string `json:"name"`
|
|
Size int64 `json:"size"`
|
|
Mode os.FileMode `json:"mode"`
|
|
Mtime time.Time `json:"mtime"`
|
|
LinkTarget string `json:"linkTarget"`
|
|
}
|
|
|
|
// GET "/containers/{name:.*}/top"
|
|
type ContainerProcessList struct {
|
|
Processes [][]string
|
|
Titles []string
|
|
}
|
|
|
|
type Version struct {
|
|
Version string
|
|
ApiVersion version.Version
|
|
GitCommit string
|
|
GoVersion string
|
|
Os string
|
|
Arch string
|
|
KernelVersion string `json:",omitempty"`
|
|
Experimental bool `json:",omitempty"`
|
|
BuildTime string `json:",omitempty"`
|
|
}
|
|
|
|
// GET "/info"
|
|
type Info struct {
|
|
ID string
|
|
Containers int
|
|
Images int
|
|
Driver string
|
|
DriverStatus [][2]string
|
|
MemoryLimit bool
|
|
SwapLimit bool
|
|
CpuCfsPeriod bool
|
|
CpuCfsQuota bool
|
|
IPv4Forwarding bool
|
|
BridgeNfIptables bool
|
|
BridgeNfIp6tables bool
|
|
Debug bool
|
|
NFd int
|
|
OomKillDisable bool
|
|
NGoroutines int
|
|
SystemTime string
|
|
ExecutionDriver string
|
|
LoggingDriver string
|
|
NEventsListener int
|
|
KernelVersion string
|
|
OperatingSystem string
|
|
IndexServerAddress string
|
|
RegistryConfig interface{}
|
|
InitSha1 string
|
|
InitPath string
|
|
NCPU int
|
|
MemTotal int64
|
|
DockerRootDir string
|
|
HttpProxy string
|
|
HttpsProxy string
|
|
NoProxy string
|
|
Name string
|
|
Labels []string
|
|
ExperimentalBuild bool
|
|
}
|
|
|
|
// This struct is a temp struct used by execStart
|
|
// Config fields is part of ExecConfig in runconfig package
|
|
type ExecStartCheck struct {
|
|
// ExecStart will first check if it's detached
|
|
Detach bool
|
|
// Check if there's a tty
|
|
Tty bool
|
|
}
|
|
|
|
type ContainerState struct {
|
|
Running bool
|
|
Paused bool
|
|
Restarting bool
|
|
OOMKilled bool
|
|
Dead bool
|
|
Pid int
|
|
ExitCode int
|
|
Error string
|
|
StartedAt string
|
|
FinishedAt string
|
|
}
|
|
|
|
// GET "/containers/{name:.*}/json"
|
|
type ContainerJSONBase struct {
|
|
Id string
|
|
Created string
|
|
Path string
|
|
Args []string
|
|
State *ContainerState
|
|
Image string
|
|
NetworkSettings *network.Settings
|
|
ResolvConfPath string
|
|
HostnamePath string
|
|
HostsPath string
|
|
LogPath string
|
|
Name string
|
|
RestartCount int
|
|
Driver string
|
|
ExecDriver string
|
|
MountLabel string
|
|
ProcessLabel string
|
|
AppArmorProfile string
|
|
ExecIDs []string
|
|
HostConfig *runconfig.HostConfig
|
|
GraphDriver GraphDriverData
|
|
}
|
|
|
|
type ContainerJSON struct {
|
|
*ContainerJSONBase
|
|
Mounts []MountPoint
|
|
Config *runconfig.Config
|
|
}
|
|
|
|
// backcompatibility struct along with ContainerConfig. Note this is not
|
|
// used by the Windows daemon.
|
|
type ContainerJSONPre120 struct {
|
|
*ContainerJSONBase
|
|
Volumes map[string]string
|
|
VolumesRW map[string]bool
|
|
Config *ContainerConfig
|
|
}
|
|
|
|
type ContainerConfig struct {
|
|
*runconfig.Config
|
|
|
|
// backward compatibility, they now live in HostConfig
|
|
Memory int64
|
|
MemorySwap int64
|
|
CpuShares int64
|
|
Cpuset string
|
|
}
|
|
|
|
// MountPoint represents a mount point configuration inside the container.
|
|
type MountPoint struct {
|
|
Name string `json:",omitempty"`
|
|
Source string
|
|
Destination string
|
|
Driver string `json:",omitempty"`
|
|
Mode string
|
|
RW bool
|
|
}
|