mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
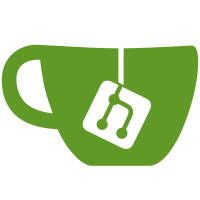
Generate a hash chain involving the image configuration, layer digests, and parent image hashes. Use the digests to compute IDs for each image in a manifest, instead of using the remotely specified IDs. To avoid breaking users' caches, check for images already in the graph under old IDs, and avoid repulling an image if the version on disk under the legacy ID ends up with the same digest that was computed from the manifest for that image. When a calculated ID already exists in the graph but can't be verified, continue trying SHA256(digest) until a suitable ID is found. "save" and "load" are not changed to use a similar scheme. "load" will preserve the IDs present in the tar file. Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com>
63 lines
3.2 KiB
Go
63 lines
3.2 KiB
Go
package runconfig
|
|
|
|
import (
|
|
"encoding/json"
|
|
"io"
|
|
|
|
"github.com/docker/docker/pkg/nat"
|
|
"github.com/docker/docker/pkg/stringutils"
|
|
)
|
|
|
|
// Config contains the configuration data about a container.
|
|
// It should hold only portable information about the container.
|
|
// Here, "portable" means "independent from the host we are running on".
|
|
// Non-portable information *should* appear in HostConfig.
|
|
// All fields added to this struct must be marked `omitempty` to keep getting
|
|
// predictable hashes from the old `v1Compatibility` configuration.
|
|
type Config struct {
|
|
Hostname string // Hostname
|
|
Domainname string // Domainname
|
|
User string // User that will run the command(s) inside the container
|
|
AttachStdin bool // Attach the standard input, makes possible user interaction
|
|
AttachStdout bool // Attach the standard output
|
|
AttachStderr bool // Attach the standard error
|
|
ExposedPorts map[nat.Port]struct{} `json:",omitempty"` // List of exposed ports
|
|
PublishService string `json:",omitempty"` // Name of the network service exposed by the container
|
|
Tty bool // Attach standard streams to a tty, including stdin if it is not closed.
|
|
OpenStdin bool // Open stdin
|
|
StdinOnce bool // If true, close stdin after the 1 attached client disconnects.
|
|
Env []string // List of environment variable to set in the container
|
|
Cmd *stringutils.StrSlice // Command to run when starting the container
|
|
Image string // Name of the image as it was passed by the operator (eg. could be symbolic)
|
|
Volumes map[string]struct{} // List of volumes (mounts) used for the container
|
|
WorkingDir string // Current directory (PWD) in the command will be launched
|
|
Entrypoint *stringutils.StrSlice // Entrypoint to run when starting the container
|
|
NetworkDisabled bool `json:",omitempty"` // Is network disabled
|
|
MacAddress string `json:",omitempty"` // Mac Address of the container
|
|
OnBuild []string // ONBUILD metadata that were defined on the image Dockerfile
|
|
Labels map[string]string // List of labels set to this container
|
|
StopSignal string `json:",omitempty"` // Signal to stop a container
|
|
}
|
|
|
|
// DecodeContainerConfig decodes a json encoded config into a ContainerConfigWrapper
|
|
// struct and returns both a Config and an HostConfig struct
|
|
// Be aware this function is not checking whether the resulted structs are nil,
|
|
// it's your business to do so
|
|
func DecodeContainerConfig(src io.Reader) (*Config, *HostConfig, error) {
|
|
decoder := json.NewDecoder(src)
|
|
|
|
var w ContainerConfigWrapper
|
|
if err := decoder.Decode(&w); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
hc := w.getHostConfig()
|
|
|
|
// Certain parameters need daemon-side validation that cannot be done
|
|
// on the client, as only the daemon knows what is valid for the platform.
|
|
if err := ValidateNetMode(w.Config, hc); err != nil {
|
|
return nil, nil, err
|
|
}
|
|
|
|
return w.Config, hc, nil
|
|
}
|