mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
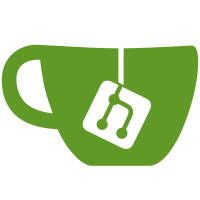
Use strongly typed errors to set HTTP status codes. Error interfaces are defined in the api/errors package and errors returned from controllers are checked against these interfaces. Errors can be wraeped in a pkg/errors.Causer, as long as somewhere in the line of causes one of the interfaces is implemented. The special error interfaces take precedence over Causer, meaning if both Causer and one of the new error interfaces are implemented, the Causer is not traversed. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
78 lines
2.1 KiB
Go
78 lines
2.1 KiB
Go
package httputils
|
|
|
|
import (
|
|
"net/http"
|
|
"path/filepath"
|
|
"strconv"
|
|
"strings"
|
|
)
|
|
|
|
// BoolValue transforms a form value in different formats into a boolean type.
|
|
func BoolValue(r *http.Request, k string) bool {
|
|
s := strings.ToLower(strings.TrimSpace(r.FormValue(k)))
|
|
return !(s == "" || s == "0" || s == "no" || s == "false" || s == "none")
|
|
}
|
|
|
|
// BoolValueOrDefault returns the default bool passed if the query param is
|
|
// missing, otherwise it's just a proxy to boolValue above.
|
|
func BoolValueOrDefault(r *http.Request, k string, d bool) bool {
|
|
if _, ok := r.Form[k]; !ok {
|
|
return d
|
|
}
|
|
return BoolValue(r, k)
|
|
}
|
|
|
|
// Int64ValueOrZero parses a form value into an int64 type.
|
|
// It returns 0 if the parsing fails.
|
|
func Int64ValueOrZero(r *http.Request, k string) int64 {
|
|
val, err := Int64ValueOrDefault(r, k, 0)
|
|
if err != nil {
|
|
return 0
|
|
}
|
|
return val
|
|
}
|
|
|
|
// Int64ValueOrDefault parses a form value into an int64 type. If there is an
|
|
// error, returns the error. If there is no value returns the default value.
|
|
func Int64ValueOrDefault(r *http.Request, field string, def int64) (int64, error) {
|
|
if r.Form.Get(field) != "" {
|
|
value, err := strconv.ParseInt(r.Form.Get(field), 10, 64)
|
|
return value, err
|
|
}
|
|
return def, nil
|
|
}
|
|
|
|
// ArchiveOptions stores archive information for different operations.
|
|
type ArchiveOptions struct {
|
|
Name string
|
|
Path string
|
|
}
|
|
|
|
type badParameterError struct {
|
|
param string
|
|
}
|
|
|
|
func (e badParameterError) Error() string {
|
|
return "bad parameter: " + e.param + "cannot be empty"
|
|
}
|
|
|
|
func (e badParameterError) InvalidParameter() {}
|
|
|
|
// ArchiveFormValues parses form values and turns them into ArchiveOptions.
|
|
// It fails if the archive name and path are not in the request.
|
|
func ArchiveFormValues(r *http.Request, vars map[string]string) (ArchiveOptions, error) {
|
|
if err := ParseForm(r); err != nil {
|
|
return ArchiveOptions{}, err
|
|
}
|
|
|
|
name := vars["name"]
|
|
if name == "" {
|
|
return ArchiveOptions{}, badParameterError{"name"}
|
|
}
|
|
|
|
path := filepath.FromSlash(r.Form.Get("path"))
|
|
if path == "" {
|
|
return ArchiveOptions{}, badParameterError{"path"}
|
|
}
|
|
return ArchiveOptions{name, path}, nil
|
|
}
|