mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
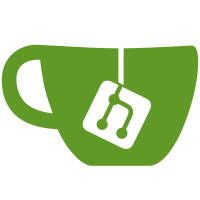
Currently, the behaviour for the version field in ServiceUpdate() is vague. Without an correct version number, users are unable to successfully run ServiceUpdate(), which is a pretty critical method for scaling services (for example). I've just added an extra sentence explaining what the version number is for, and where to find it. Signed-off-by: Harrison Turton <harrisonturton@gmail.com>
94 lines
2.8 KiB
Go
94 lines
2.8 KiB
Go
package client // import "github.com/docker/docker/client"
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
"net/url"
|
|
"strconv"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/swarm"
|
|
)
|
|
|
|
// ServiceUpdate updates a Service. The version number is required to avoid conflicting writes.
|
|
// It should be the value as set *before* the update. You can find this value in the Meta field
|
|
// of swarm.Service, which can be found using ServiceInspectWithRaw.
|
|
func (cli *Client) ServiceUpdate(ctx context.Context, serviceID string, version swarm.Version, service swarm.ServiceSpec, options types.ServiceUpdateOptions) (types.ServiceUpdateResponse, error) {
|
|
var (
|
|
query = url.Values{}
|
|
distErr error
|
|
)
|
|
|
|
headers := map[string][]string{
|
|
"version": {cli.version},
|
|
}
|
|
|
|
if options.EncodedRegistryAuth != "" {
|
|
headers["X-Registry-Auth"] = []string{options.EncodedRegistryAuth}
|
|
}
|
|
|
|
if options.RegistryAuthFrom != "" {
|
|
query.Set("registryAuthFrom", options.RegistryAuthFrom)
|
|
}
|
|
|
|
if options.Rollback != "" {
|
|
query.Set("rollback", options.Rollback)
|
|
}
|
|
|
|
query.Set("version", strconv.FormatUint(version.Index, 10))
|
|
|
|
if err := validateServiceSpec(service); err != nil {
|
|
return types.ServiceUpdateResponse{}, err
|
|
}
|
|
|
|
var imgPlatforms []swarm.Platform
|
|
// ensure that the image is tagged
|
|
if service.TaskTemplate.ContainerSpec != nil {
|
|
if taggedImg := imageWithTagString(service.TaskTemplate.ContainerSpec.Image); taggedImg != "" {
|
|
service.TaskTemplate.ContainerSpec.Image = taggedImg
|
|
}
|
|
if options.QueryRegistry {
|
|
var img string
|
|
img, imgPlatforms, distErr = imageDigestAndPlatforms(ctx, cli, service.TaskTemplate.ContainerSpec.Image, options.EncodedRegistryAuth)
|
|
if img != "" {
|
|
service.TaskTemplate.ContainerSpec.Image = img
|
|
}
|
|
}
|
|
}
|
|
|
|
// ensure that the image is tagged
|
|
if service.TaskTemplate.PluginSpec != nil {
|
|
if taggedImg := imageWithTagString(service.TaskTemplate.PluginSpec.Remote); taggedImg != "" {
|
|
service.TaskTemplate.PluginSpec.Remote = taggedImg
|
|
}
|
|
if options.QueryRegistry {
|
|
var img string
|
|
img, imgPlatforms, distErr = imageDigestAndPlatforms(ctx, cli, service.TaskTemplate.PluginSpec.Remote, options.EncodedRegistryAuth)
|
|
if img != "" {
|
|
service.TaskTemplate.PluginSpec.Remote = img
|
|
}
|
|
}
|
|
}
|
|
|
|
if service.TaskTemplate.Placement == nil && len(imgPlatforms) > 0 {
|
|
service.TaskTemplate.Placement = &swarm.Placement{}
|
|
}
|
|
if len(imgPlatforms) > 0 {
|
|
service.TaskTemplate.Placement.Platforms = imgPlatforms
|
|
}
|
|
|
|
var response types.ServiceUpdateResponse
|
|
resp, err := cli.post(ctx, "/services/"+serviceID+"/update", query, service, headers)
|
|
if err != nil {
|
|
return response, err
|
|
}
|
|
|
|
err = json.NewDecoder(resp.body).Decode(&response)
|
|
|
|
if distErr != nil {
|
|
response.Warnings = append(response.Warnings, digestWarning(service.TaskTemplate.ContainerSpec.Image))
|
|
}
|
|
|
|
ensureReaderClosed(resp)
|
|
return response, err
|
|
}
|