mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
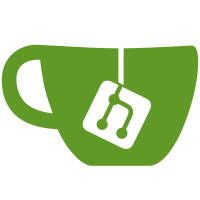
… to make sure it doesn't fail. It also introduce StartWithError, StopWithError and RestartWithError in case we care about the error (and want the error to happen). This removes the need to check for error and make the intent more clear : I want a deamon with busybox loaded on it — if an error occur it should fail the test, but it's not the test code that has the responsability to check that. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
52 lines
1.6 KiB
Go
52 lines
1.6 KiB
Go
package main
|
|
|
|
import (
|
|
"net"
|
|
"os/exec"
|
|
"strings"
|
|
|
|
"github.com/docker/docker/pkg/integration/checker"
|
|
"github.com/go-check/check"
|
|
)
|
|
|
|
func (s *DockerSuite) TestCLIProxyDisableProxyUnixSock(c *check.C) {
|
|
testRequires(c, DaemonIsLinux)
|
|
testRequires(c, SameHostDaemon) // test is valid when DOCKER_HOST=unix://..
|
|
|
|
cmd := exec.Command(dockerBinary, "info")
|
|
cmd.Env = appendBaseEnv(false, "HTTP_PROXY=http://127.0.0.1:9999")
|
|
|
|
out, _, err := runCommandWithOutput(cmd)
|
|
c.Assert(err, checker.IsNil, check.Commentf("%v", out))
|
|
|
|
}
|
|
|
|
// Can't use localhost here since go has a special case to not use proxy if connecting to localhost
|
|
// See https://golang.org/pkg/net/http/#ProxyFromEnvironment
|
|
func (s *DockerDaemonSuite) TestCLIProxyProxyTCPSock(c *check.C) {
|
|
testRequires(c, SameHostDaemon)
|
|
// get the IP to use to connect since we can't use localhost
|
|
addrs, err := net.InterfaceAddrs()
|
|
c.Assert(err, checker.IsNil)
|
|
var ip string
|
|
for _, addr := range addrs {
|
|
sAddr := addr.String()
|
|
if !strings.Contains(sAddr, "127.0.0.1") {
|
|
addrArr := strings.Split(sAddr, "/")
|
|
ip = addrArr[0]
|
|
break
|
|
}
|
|
}
|
|
|
|
c.Assert(ip, checker.Not(checker.Equals), "")
|
|
|
|
s.d.Start(c, "-H", "tcp://"+ip+":2375")
|
|
cmd := exec.Command(dockerBinary, "info")
|
|
cmd.Env = []string{"DOCKER_HOST=tcp://" + ip + ":2375", "HTTP_PROXY=127.0.0.1:9999"}
|
|
out, _, err := runCommandWithOutput(cmd)
|
|
c.Assert(err, checker.NotNil, check.Commentf("%v", out))
|
|
// Test with no_proxy
|
|
cmd.Env = append(cmd.Env, "NO_PROXY="+ip)
|
|
out, _, err = runCommandWithOutput(exec.Command(dockerBinary, "info"))
|
|
c.Assert(err, checker.IsNil, check.Commentf("%v", out))
|
|
}
|