mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
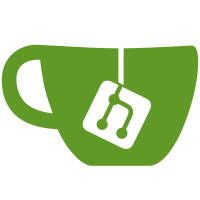
This remove a dependency on `go-check` (and more) when using `pkg/idtools`. `pkg/integration` should never be called from any other package then `integration`. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
33 lines
901 B
Go
33 lines
901 B
Go
package system
|
|
|
|
import (
|
|
"fmt"
|
|
"os/exec"
|
|
"syscall"
|
|
)
|
|
|
|
// GetExitCode returns the ExitStatus of the specified error if its type is
|
|
// exec.ExitError, returns 0 and an error otherwise.
|
|
func GetExitCode(err error) (int, error) {
|
|
exitCode := 0
|
|
if exiterr, ok := err.(*exec.ExitError); ok {
|
|
if procExit, ok := exiterr.Sys().(syscall.WaitStatus); ok {
|
|
return procExit.ExitStatus(), nil
|
|
}
|
|
}
|
|
return exitCode, fmt.Errorf("failed to get exit code")
|
|
}
|
|
|
|
// ProcessExitCode process the specified error and returns the exit status code
|
|
// if the error was of type exec.ExitError, returns nothing otherwise.
|
|
func ProcessExitCode(err error) (exitCode int) {
|
|
if err != nil {
|
|
var exiterr error
|
|
if exitCode, exiterr = GetExitCode(err); exiterr != nil {
|
|
// TODO: Fix this so we check the error's text.
|
|
// we've failed to retrieve exit code, so we set it to 127
|
|
exitCode = 127
|
|
}
|
|
}
|
|
return
|
|
}
|