mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
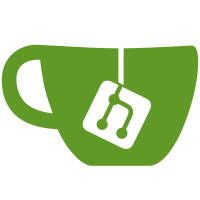
Signed-off-by: Evan Hazlett <ejhazlett@gmail.com> wip: use tmpfs for swarm secrets Signed-off-by: Evan Hazlett <ejhazlett@gmail.com> wip: inject secrets from swarm secret store Signed-off-by: Evan Hazlett <ejhazlett@gmail.com> secrets: use secret names in cli for service create Signed-off-by: Evan Hazlett <ejhazlett@gmail.com> switch to use mounts instead of volumes Signed-off-by: Evan Hazlett <ejhazlett@gmail.com> vendor: use ehazlett swarmkit Signed-off-by: Evan Hazlett <ejhazlett@gmail.com> secrets: finish secret update Signed-off-by: Evan Hazlett <ejhazlett@gmail.com>
34 lines
793 B
Go
34 lines
793 B
Go
package client
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/json"
|
|
"io/ioutil"
|
|
"net/http"
|
|
|
|
"github.com/docker/docker/api/types/swarm"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
// SecretInspectWithRaw returns the secret information with raw data
|
|
func (cli *Client) SecretInspectWithRaw(ctx context.Context, id string) (swarm.Secret, []byte, error) {
|
|
resp, err := cli.get(ctx, "/secrets/"+id, nil, nil)
|
|
if err != nil {
|
|
if resp.statusCode == http.StatusNotFound {
|
|
return swarm.Secret{}, nil, secretNotFoundError{id}
|
|
}
|
|
return swarm.Secret{}, nil, err
|
|
}
|
|
defer ensureReaderClosed(resp)
|
|
|
|
body, err := ioutil.ReadAll(resp.body)
|
|
if err != nil {
|
|
return swarm.Secret{}, nil, err
|
|
}
|
|
|
|
var secret swarm.Secret
|
|
rdr := bytes.NewReader(body)
|
|
err = json.NewDecoder(rdr).Decode(&secret)
|
|
|
|
return secret, body, err
|
|
}
|