mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
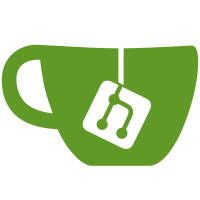
`Mounts` allows users to specify in a much safer way the volumes they want to use in the container. This replaces `Binds` and `Volumes`, which both still exist, but `Mounts` and `Binds`/`Volumes` are exclussive. The CLI will continue to use `Binds` and `Volumes` due to concerns with parsing the volume specs on the client side and cross-platform support (for now). The new API follows exactly the services mount API. Example usage of `Mounts`: ``` $ curl -XPOST localhost:2375/containers/create -d '{ "Image": "alpine:latest", "HostConfig": { "Mounts": [{ "Type": "Volume", "Target": "/foo" },{ "Type": "bind", "Source": "/var/run/docker.sock", "Target": "/var/run/docker.sock", },{ "Type": "volume", "Name": "important_data", "Target": "/var/data", "ReadOnly": true, "VolumeOptions": { "DriverConfig": { Name: "awesomeStorage", Options: {"size": "10m"}, Labels: {"some":"label"} } }] } }' ``` There are currently 2 types of mounts: - **bind**: Paths on the host that get mounted into the container. Paths must exist prior to creating the container. - **volume**: Volumes that persist after the container is removed. Not all fields are available in each type, and validation is done to ensure these fields aren't mixed up between types. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
41 lines
1.2 KiB
Go
41 lines
1.2 KiB
Go
package daemon
|
|
|
|
import (
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/backend"
|
|
"github.com/docker/docker/container"
|
|
"github.com/docker/docker/daemon/exec"
|
|
)
|
|
|
|
// This sets platform-specific fields
|
|
func setPlatformSpecificContainerFields(container *container.Container, contJSONBase *types.ContainerJSONBase) *types.ContainerJSONBase {
|
|
return contJSONBase
|
|
}
|
|
|
|
func addMountPoints(container *container.Container) []types.MountPoint {
|
|
mountPoints := make([]types.MountPoint, 0, len(container.MountPoints))
|
|
for _, m := range container.MountPoints {
|
|
mountPoints = append(mountPoints, types.MountPoint{
|
|
Type: m.Type,
|
|
Name: m.Name,
|
|
Source: m.Path(),
|
|
Destination: m.Destination,
|
|
Driver: m.Driver,
|
|
RW: m.RW,
|
|
})
|
|
}
|
|
return mountPoints
|
|
}
|
|
|
|
// containerInspectPre120 get containers for pre 1.20 APIs.
|
|
func (daemon *Daemon) containerInspectPre120(name string) (*types.ContainerJSON, error) {
|
|
return daemon.ContainerInspectCurrent(name, false)
|
|
}
|
|
|
|
func inspectExecProcessConfig(e *exec.Config) *backend.ExecProcessConfig {
|
|
return &backend.ExecProcessConfig{
|
|
Tty: e.Tty,
|
|
Entrypoint: e.Entrypoint,
|
|
Arguments: e.Args,
|
|
}
|
|
}
|