mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
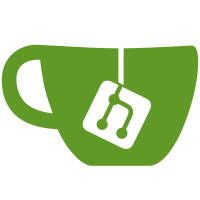
containers may specify these cgroup values at runtime. This will allow processes to change their priority to real-time within the container when CONFIG_RT_GROUP_SCHED is enabled in the kernel. See #22380. Also added sanity checks for the new --cpu-rt-runtime and --cpu-rt-period flags to ensure that that the kernel supports these features and that runtime is not greater than period. Daemon will support a --cpu-rt-runtime flag to initialize the parent cgroup on startup, this prevents the administrator from alotting runtime to docker after each restart. There are additional checks that could be added but maybe too far? Check parent cgroups to ensure values are <= parent, inspecting rtprio ulimit and issuing a warning. Signed-off-by: Erik St. Martin <alakriti@gmail.com>
68 lines
2 KiB
Go
68 lines
2 KiB
Go
package runconfig
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/docker/docker/api/types/container"
|
|
"github.com/docker/docker/pkg/sysinfo"
|
|
)
|
|
|
|
// DefaultDaemonNetworkMode returns the default network stack the daemon should
|
|
// use.
|
|
func DefaultDaemonNetworkMode() container.NetworkMode {
|
|
return container.NetworkMode("nat")
|
|
}
|
|
|
|
// IsPreDefinedNetwork indicates if a network is predefined by the daemon
|
|
func IsPreDefinedNetwork(network string) bool {
|
|
return !container.NetworkMode(network).IsUserDefined()
|
|
}
|
|
|
|
// ValidateNetMode ensures that the various combinations of requested
|
|
// network settings are valid.
|
|
func ValidateNetMode(c *container.Config, hc *container.HostConfig) error {
|
|
if hc == nil {
|
|
return nil
|
|
}
|
|
parts := strings.Split(string(hc.NetworkMode), ":")
|
|
if len(parts) > 1 {
|
|
return fmt.Errorf("invalid --net: %s", hc.NetworkMode)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// ValidateIsolation performs platform specific validation of the
|
|
// isolation in the hostconfig structure. Windows supports 'default' (or
|
|
// blank), 'process', or 'hyperv'.
|
|
func ValidateIsolation(hc *container.HostConfig) error {
|
|
// We may not be passed a host config, such as in the case of docker commit
|
|
if hc == nil {
|
|
return nil
|
|
}
|
|
if !hc.Isolation.IsValid() {
|
|
return fmt.Errorf("invalid --isolation: %q. Windows supports 'default', 'process', or 'hyperv'", hc.Isolation)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// ValidateQoS performs platform specific validation of the Qos settings
|
|
func ValidateQoS(hc *container.HostConfig) error {
|
|
return nil
|
|
}
|
|
|
|
// ValidateResources performs platform specific validation of the resource settings
|
|
func ValidateResources(hc *container.HostConfig, si *sysinfo.SysInfo) error {
|
|
// We may not be passed a host config, such as in the case of docker commit
|
|
if hc == nil {
|
|
return nil
|
|
}
|
|
|
|
if hc.Resources.CPURealtimePeriod != 0 {
|
|
return fmt.Errorf("invalid --cpu-rt-period: Windows does not support this feature")
|
|
}
|
|
if hc.Resources.CPURealtimeRuntime != 0 {
|
|
return fmt.Errorf("invalid --cpu-rt-runtime: Windows does not support this feature")
|
|
}
|
|
return nil
|
|
}
|