mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
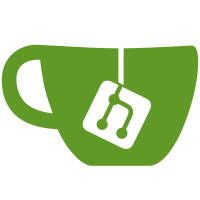
Sometimes third attacher attaching to already stopped container. Also I've changed prefix to attach and fixed cleanup on Fatal. Docker-DCO-1.1-Signed-off-by: Alexandr Morozov <lk4d4math@gmail.com> (github: LK4D4)
53 lines
1,018 B
Go
53 lines
1,018 B
Go
package main
|
|
|
|
import (
|
|
"os/exec"
|
|
"strings"
|
|
"sync"
|
|
"testing"
|
|
"time"
|
|
)
|
|
|
|
func TestMultipleAttachRestart(t *testing.T) {
|
|
cmd := exec.Command(dockerBinary, "run", "--name", "attacher", "-d", "busybox",
|
|
"/bin/sh", "-c", "sleep 2 && echo hello")
|
|
|
|
group := sync.WaitGroup{}
|
|
group.Add(4)
|
|
|
|
defer func() {
|
|
cmd = exec.Command(dockerBinary, "kill", "attacher")
|
|
if _, err := runCommand(cmd); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
deleteAllContainers()
|
|
}()
|
|
|
|
go func() {
|
|
defer group.Done()
|
|
out, _, err := runCommandWithOutput(cmd)
|
|
if err != nil {
|
|
t.Fatal(err, out)
|
|
}
|
|
}()
|
|
time.Sleep(500 * time.Millisecond)
|
|
|
|
for i := 0; i < 3; i++ {
|
|
go func() {
|
|
defer group.Done()
|
|
c := exec.Command(dockerBinary, "attach", "attacher")
|
|
|
|
out, _, err := runCommandWithOutput(c)
|
|
if err != nil {
|
|
t.Fatal(err, out)
|
|
}
|
|
if actual := strings.Trim(out, "\r\n"); actual != "hello" {
|
|
t.Fatalf("unexpected output %s expected hello", actual)
|
|
}
|
|
}()
|
|
}
|
|
|
|
group.Wait()
|
|
|
|
logDone("attach - multiple attach")
|
|
}
|