mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
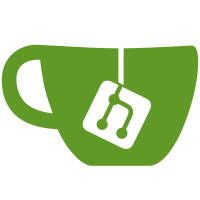
A previous change added a TTY fixup for stdin on older Windows versions to work around a Windows issue with backspace/delete behavior. This change used the OS version to determine whether to activate the behavior. However, the Windows bug is actually in the image, not the OS, so it should have used the image's OS version. This ensures that a Server TP5 container running on Windows 10 will have reasonable console behavior. Signed-off-by: John Starks <jostarks@microsoft.com>
95 lines
1.8 KiB
Go
95 lines
1.8 KiB
Go
package libcontainerd
|
|
|
|
import (
|
|
"io"
|
|
"strconv"
|
|
"strings"
|
|
|
|
"github.com/Microsoft/hcsshim"
|
|
)
|
|
|
|
// process keeps the state for both main container process and exec process.
|
|
type process struct {
|
|
processCommon
|
|
|
|
// Platform specific fields are below here.
|
|
|
|
// commandLine is to support returning summary information for docker top
|
|
commandLine string
|
|
hcsProcess hcsshim.Process
|
|
}
|
|
|
|
func openReaderFromPipe(p io.ReadCloser) io.Reader {
|
|
r, w := io.Pipe()
|
|
go func() {
|
|
if _, err := io.Copy(w, p); err != nil {
|
|
r.CloseWithError(err)
|
|
}
|
|
w.Close()
|
|
p.Close()
|
|
}()
|
|
return r
|
|
}
|
|
|
|
// fixStdinBackspaceBehavior works around a bug in Windows before build 14350
|
|
// where it interpreted DEL as VK_DELETE instead of as VK_BACK. This replaces
|
|
// DEL with BS to work around this.
|
|
func fixStdinBackspaceBehavior(w io.WriteCloser, osversion string, tty bool) io.WriteCloser {
|
|
if !tty {
|
|
return w
|
|
}
|
|
v := strings.Split(osversion, ".")
|
|
if len(v) < 3 {
|
|
return w
|
|
}
|
|
|
|
if build, err := strconv.Atoi(v[2]); err != nil || build >= 14350 {
|
|
return w
|
|
}
|
|
|
|
return &delToBsWriter{w}
|
|
}
|
|
|
|
type delToBsWriter struct {
|
|
io.WriteCloser
|
|
}
|
|
|
|
func (w *delToBsWriter) Write(b []byte) (int, error) {
|
|
const (
|
|
backspace = 0x8
|
|
del = 0x7f
|
|
)
|
|
bc := make([]byte, len(b))
|
|
for i, c := range b {
|
|
if c == del {
|
|
bc[i] = backspace
|
|
} else {
|
|
bc[i] = c
|
|
}
|
|
}
|
|
return w.WriteCloser.Write(bc)
|
|
}
|
|
|
|
type stdInCloser struct {
|
|
io.WriteCloser
|
|
hcsshim.Process
|
|
}
|
|
|
|
func createStdInCloser(pipe io.WriteCloser, process hcsshim.Process) *stdInCloser {
|
|
return &stdInCloser{
|
|
WriteCloser: pipe,
|
|
Process: process,
|
|
}
|
|
}
|
|
|
|
func (stdin *stdInCloser) Close() error {
|
|
if err := stdin.WriteCloser.Close(); err != nil {
|
|
return err
|
|
}
|
|
|
|
return stdin.Process.CloseStdin()
|
|
}
|
|
|
|
func (stdin *stdInCloser) Write(p []byte) (n int, err error) {
|
|
return stdin.WriteCloser.Write(p)
|
|
}
|