mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
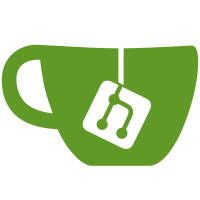
Use a tagged release of Cobra. All relevant PR's were merged, so the fork is no longer needed. Relevant changes: - spf13/cobra#552 Add a field to disable [flags] in UseLine() - spf13/cobra#567 Add `CalledAs` method to cobra.Command - spf13/cobra#580 Update error message for missing required flags - spf13/cobra#584 Add support for --version flag - spf13/cobra#614 If user has a project in symlink, just use its destination folder and work there - spf13/cobra#649 terminates the flags when -- is found in commandline - spf13/cobra#662 Add support for ignoring parse errors - spf13/cobra#686 doc: hide hidden parent flags Also various improvements were added for generating Bash completion scripts (currently not used by us) Fixes usage output for dockerd; Before this update: dockerd --help Usage: dockerd COMMAND A self-sufficient runtime for containers. After this update: dockerd --help Usage: dockerd [OPTIONS] [flags] A self-sufficient runtime for containers. Bump spf13/pflag to v1.0.1 Relevant changes: - spf13/pflag#106 allow lookup by shorthand - spf13/pflag#113 Add SortFlags option - spf13/pflag#138 Generate flag error output for errors returned from the parseFunc - spf13/pflag#141 Fixing Count flag usage string - spf13/pflag#143 add int16 flag - spf13/pflag#122 DurationSlice: implementation and tests - spf13/pflag#115 Implement BytesHex type of argument - spf13/pflag#150 Add uintSlice and boolSlice to name prettifier - spf13/pflag#155 Add multiline wrapping support - spf13/pflag#158 doc: clarify difference between string slice vs. array - spf13/pflag#160 add ability to ignore unknown flags - spf13/pflag#163 Allow Users To Show Deprecated Flags Hide [flags] in usage output Hides the [flags] in the usage output of commands (present in newer versions of Cobra), using the `.DisableFlagsInUseLine` option. Before this change: dockerd --help Usage: dockerd [OPTIONS] [flags] A self-sufficient runtime for containers. After this change: dockerd --help Usage: dockerd [OPTIONS] A self-sufficient runtime for containers. Signed-off-by: Sebastiaan van Stijn <github@gone.nl> Â# modified: vendor/github.com/spf13/pflag/string_array.go § Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
126 lines
2.7 KiB
Go
126 lines
2.7 KiB
Go
package cobra
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
"io"
|
|
"os"
|
|
"strings"
|
|
)
|
|
|
|
// GenZshCompletionFile generates zsh completion file.
|
|
func (c *Command) GenZshCompletionFile(filename string) error {
|
|
outFile, err := os.Create(filename)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer outFile.Close()
|
|
|
|
return c.GenZshCompletion(outFile)
|
|
}
|
|
|
|
// GenZshCompletion generates a zsh completion file and writes to the passed writer.
|
|
func (c *Command) GenZshCompletion(w io.Writer) error {
|
|
buf := new(bytes.Buffer)
|
|
|
|
writeHeader(buf, c)
|
|
maxDepth := maxDepth(c)
|
|
writeLevelMapping(buf, maxDepth)
|
|
writeLevelCases(buf, maxDepth, c)
|
|
|
|
_, err := buf.WriteTo(w)
|
|
return err
|
|
}
|
|
|
|
func writeHeader(w io.Writer, cmd *Command) {
|
|
fmt.Fprintf(w, "#compdef %s\n\n", cmd.Name())
|
|
}
|
|
|
|
func maxDepth(c *Command) int {
|
|
if len(c.Commands()) == 0 {
|
|
return 0
|
|
}
|
|
maxDepthSub := 0
|
|
for _, s := range c.Commands() {
|
|
subDepth := maxDepth(s)
|
|
if subDepth > maxDepthSub {
|
|
maxDepthSub = subDepth
|
|
}
|
|
}
|
|
return 1 + maxDepthSub
|
|
}
|
|
|
|
func writeLevelMapping(w io.Writer, numLevels int) {
|
|
fmt.Fprintln(w, `_arguments \`)
|
|
for i := 1; i <= numLevels; i++ {
|
|
fmt.Fprintf(w, ` '%d: :->level%d' \`, i, i)
|
|
fmt.Fprintln(w)
|
|
}
|
|
fmt.Fprintf(w, ` '%d: :%s'`, numLevels+1, "_files")
|
|
fmt.Fprintln(w)
|
|
}
|
|
|
|
func writeLevelCases(w io.Writer, maxDepth int, root *Command) {
|
|
fmt.Fprintln(w, "case $state in")
|
|
defer fmt.Fprintln(w, "esac")
|
|
|
|
for i := 1; i <= maxDepth; i++ {
|
|
fmt.Fprintf(w, " level%d)\n", i)
|
|
writeLevel(w, root, i)
|
|
fmt.Fprintln(w, " ;;")
|
|
}
|
|
fmt.Fprintln(w, " *)")
|
|
fmt.Fprintln(w, " _arguments '*: :_files'")
|
|
fmt.Fprintln(w, " ;;")
|
|
}
|
|
|
|
func writeLevel(w io.Writer, root *Command, i int) {
|
|
fmt.Fprintf(w, " case $words[%d] in\n", i)
|
|
defer fmt.Fprintln(w, " esac")
|
|
|
|
commands := filterByLevel(root, i)
|
|
byParent := groupByParent(commands)
|
|
|
|
for p, c := range byParent {
|
|
names := names(c)
|
|
fmt.Fprintf(w, " %s)\n", p)
|
|
fmt.Fprintf(w, " _arguments '%d: :(%s)'\n", i, strings.Join(names, " "))
|
|
fmt.Fprintln(w, " ;;")
|
|
}
|
|
fmt.Fprintln(w, " *)")
|
|
fmt.Fprintln(w, " _arguments '*: :_files'")
|
|
fmt.Fprintln(w, " ;;")
|
|
|
|
}
|
|
|
|
func filterByLevel(c *Command, l int) []*Command {
|
|
cs := make([]*Command, 0)
|
|
if l == 0 {
|
|
cs = append(cs, c)
|
|
return cs
|
|
}
|
|
for _, s := range c.Commands() {
|
|
cs = append(cs, filterByLevel(s, l-1)...)
|
|
}
|
|
return cs
|
|
}
|
|
|
|
func groupByParent(commands []*Command) map[string][]*Command {
|
|
m := make(map[string][]*Command)
|
|
for _, c := range commands {
|
|
parent := c.Parent()
|
|
if parent == nil {
|
|
continue
|
|
}
|
|
m[parent.Name()] = append(m[parent.Name()], c)
|
|
}
|
|
return m
|
|
}
|
|
|
|
func names(commands []*Command) []string {
|
|
ns := make([]string, len(commands))
|
|
for i, c := range commands {
|
|
ns[i] = c.Name()
|
|
}
|
|
return ns
|
|
}
|