mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
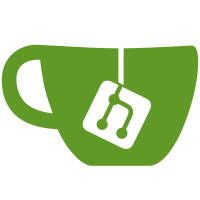
Add distribution package for managing pulls and pushes. This is based on the old code in the graph package, with major changes to work with the new image/layer model. Add v1 migration code. Update registry, api/*, and daemon packages to use the reference package's types where applicable. Update daemon package to use image/layer/tag stores instead of the graph package Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com> Signed-off-by: Tonis Tiigi <tonistiigi@gmail.com>
265 lines
11 KiB
Go
265 lines
11 KiB
Go
package parsers
|
|
|
|
import (
|
|
"reflect"
|
|
"runtime"
|
|
"strings"
|
|
"testing"
|
|
)
|
|
|
|
func TestParseDockerDaemonHost(t *testing.T) {
|
|
var (
|
|
defaultHTTPHost = "tcp://localhost:2375"
|
|
defaultHTTPSHost = "tcp://localhost:2376"
|
|
defaultUnix = "/var/run/docker.sock"
|
|
defaultHOST = "unix:///var/run/docker.sock"
|
|
)
|
|
if runtime.GOOS == "windows" {
|
|
defaultHOST = defaultHTTPHost
|
|
}
|
|
invalids := map[string]string{
|
|
"0.0.0.0": "Invalid bind address format: 0.0.0.0",
|
|
"tcp:a.b.c.d": "Invalid bind address format: tcp:a.b.c.d",
|
|
"tcp:a.b.c.d/path": "Invalid bind address format: tcp:a.b.c.d/path",
|
|
"udp://127.0.0.1": "Invalid bind address format: udp://127.0.0.1",
|
|
"udp://127.0.0.1:2375": "Invalid bind address format: udp://127.0.0.1:2375",
|
|
"tcp://unix:///run/docker.sock": "Invalid bind address format: unix",
|
|
"tcp": "Invalid bind address format: tcp",
|
|
"unix": "Invalid bind address format: unix",
|
|
"fd": "Invalid bind address format: fd",
|
|
}
|
|
valids := map[string]string{
|
|
"0.0.0.1:": "tcp://0.0.0.1:2375",
|
|
"0.0.0.1:5555": "tcp://0.0.0.1:5555",
|
|
"0.0.0.1:5555/path": "tcp://0.0.0.1:5555/path",
|
|
"[::1]:": "tcp://[::1]:2375",
|
|
"[::1]:5555/path": "tcp://[::1]:5555/path",
|
|
"[0:0:0:0:0:0:0:1]:": "tcp://[0:0:0:0:0:0:0:1]:2375",
|
|
"[0:0:0:0:0:0:0:1]:5555/path": "tcp://[0:0:0:0:0:0:0:1]:5555/path",
|
|
":6666": "tcp://localhost:6666",
|
|
":6666/path": "tcp://localhost:6666/path",
|
|
"": defaultHOST,
|
|
" ": defaultHOST,
|
|
" ": defaultHOST,
|
|
"tcp://": defaultHTTPHost,
|
|
"tcp://:7777": "tcp://localhost:7777",
|
|
"tcp://:7777/path": "tcp://localhost:7777/path",
|
|
" tcp://:7777/path ": "tcp://localhost:7777/path",
|
|
"unix:///run/docker.sock": "unix:///run/docker.sock",
|
|
"unix://": "unix:///var/run/docker.sock",
|
|
"fd://": "fd://",
|
|
"fd://something": "fd://something",
|
|
"localhost:": "tcp://localhost:2375",
|
|
"localhost:5555": "tcp://localhost:5555",
|
|
"localhost:5555/path": "tcp://localhost:5555/path",
|
|
}
|
|
for invalidAddr, expectedError := range invalids {
|
|
if addr, err := ParseDockerDaemonHost(defaultHTTPHost, defaultHTTPSHost, defaultUnix, "", invalidAddr); err == nil || err.Error() != expectedError {
|
|
t.Errorf("tcp %v address expected error %v return, got %s and addr %v", invalidAddr, expectedError, err, addr)
|
|
}
|
|
}
|
|
for validAddr, expectedAddr := range valids {
|
|
if addr, err := ParseDockerDaemonHost(defaultHTTPHost, defaultHTTPSHost, defaultUnix, "", validAddr); err != nil || addr != expectedAddr {
|
|
t.Errorf("%v -> expected %v, got (%v) addr (%v)", validAddr, expectedAddr, err, addr)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestParseTCP(t *testing.T) {
|
|
var (
|
|
defaultHTTPHost = "tcp://127.0.0.1:2376"
|
|
)
|
|
invalids := map[string]string{
|
|
"0.0.0.0": "Invalid bind address format: 0.0.0.0",
|
|
"tcp:a.b.c.d": "Invalid bind address format: tcp:a.b.c.d",
|
|
"tcp:a.b.c.d/path": "Invalid bind address format: tcp:a.b.c.d/path",
|
|
"udp://127.0.0.1": "Invalid proto, expected tcp: udp://127.0.0.1",
|
|
"udp://127.0.0.1:2375": "Invalid proto, expected tcp: udp://127.0.0.1:2375",
|
|
}
|
|
valids := map[string]string{
|
|
"": defaultHTTPHost,
|
|
"tcp://": defaultHTTPHost,
|
|
"0.0.0.1:": "tcp://0.0.0.1:2376",
|
|
"0.0.0.1:5555": "tcp://0.0.0.1:5555",
|
|
"0.0.0.1:5555/path": "tcp://0.0.0.1:5555/path",
|
|
":6666": "tcp://127.0.0.1:6666",
|
|
":6666/path": "tcp://127.0.0.1:6666/path",
|
|
"tcp://:7777": "tcp://127.0.0.1:7777",
|
|
"tcp://:7777/path": "tcp://127.0.0.1:7777/path",
|
|
"[::1]:": "tcp://[::1]:2376",
|
|
"[::1]:5555": "tcp://[::1]:5555",
|
|
"[::1]:5555/path": "tcp://[::1]:5555/path",
|
|
"[0:0:0:0:0:0:0:1]:": "tcp://[0:0:0:0:0:0:0:1]:2376",
|
|
"[0:0:0:0:0:0:0:1]:5555": "tcp://[0:0:0:0:0:0:0:1]:5555",
|
|
"[0:0:0:0:0:0:0:1]:5555/path": "tcp://[0:0:0:0:0:0:0:1]:5555/path",
|
|
"localhost:": "tcp://localhost:2376",
|
|
"localhost:5555": "tcp://localhost:5555",
|
|
"localhost:5555/path": "tcp://localhost:5555/path",
|
|
}
|
|
for invalidAddr, expectedError := range invalids {
|
|
if addr, err := ParseTCPAddr(invalidAddr, defaultHTTPHost); err == nil || err.Error() != expectedError {
|
|
t.Errorf("tcp %v address expected error %v return, got %s and addr %v", invalidAddr, expectedError, err, addr)
|
|
}
|
|
}
|
|
for validAddr, expectedAddr := range valids {
|
|
if addr, err := ParseTCPAddr(validAddr, defaultHTTPHost); err != nil || addr != expectedAddr {
|
|
t.Errorf("%v -> expected %v, got %v and addr %v", validAddr, expectedAddr, err, addr)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestParseInvalidUnixAddrInvalid(t *testing.T) {
|
|
if _, err := ParseUnixAddr("tcp://127.0.0.1", "unix:///var/run/docker.sock"); err == nil || err.Error() != "Invalid proto, expected unix: tcp://127.0.0.1" {
|
|
t.Fatalf("Expected an error, got %v", err)
|
|
}
|
|
if _, err := ParseUnixAddr("unix://tcp://127.0.0.1", "/var/run/docker.sock"); err == nil || err.Error() != "Invalid proto, expected unix: tcp://127.0.0.1" {
|
|
t.Fatalf("Expected an error, got %v", err)
|
|
}
|
|
if v, err := ParseUnixAddr("", "/var/run/docker.sock"); err != nil || v != "unix:///var/run/docker.sock" {
|
|
t.Fatalf("Expected an %v, got %v", v, "unix:///var/run/docker.sock")
|
|
}
|
|
}
|
|
|
|
func TestParseKeyValueOpt(t *testing.T) {
|
|
invalids := map[string]string{
|
|
"": "Unable to parse key/value option: ",
|
|
"key": "Unable to parse key/value option: key",
|
|
}
|
|
for invalid, expectedError := range invalids {
|
|
if _, _, err := ParseKeyValueOpt(invalid); err == nil || err.Error() != expectedError {
|
|
t.Fatalf("Expected error %v for %v, got %v", expectedError, invalid, err)
|
|
}
|
|
}
|
|
valids := map[string][]string{
|
|
"key=value": {"key", "value"},
|
|
" key = value ": {"key", "value"},
|
|
"key=value1=value2": {"key", "value1=value2"},
|
|
" key = value1 = value2 ": {"key", "value1 = value2"},
|
|
}
|
|
for valid, expectedKeyValue := range valids {
|
|
key, value, err := ParseKeyValueOpt(valid)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if key != expectedKeyValue[0] || value != expectedKeyValue[1] {
|
|
t.Fatalf("Expected {%v: %v} got {%v: %v}", expectedKeyValue[0], expectedKeyValue[1], key, value)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestParsePortRange(t *testing.T) {
|
|
if start, end, err := ParsePortRange("8000-8080"); err != nil || start != 8000 || end != 8080 {
|
|
t.Fatalf("Error: %s or Expecting {start,end} values {8000,8080} but found {%d,%d}.", err, start, end)
|
|
}
|
|
}
|
|
|
|
func TestParsePortRangeEmpty(t *testing.T) {
|
|
if _, _, err := ParsePortRange(""); err == nil || err.Error() != "Empty string specified for ports." {
|
|
t.Fatalf("Expected error 'Empty string specified for ports.', got %v", err)
|
|
}
|
|
}
|
|
|
|
func TestParsePortRangeWithNoRange(t *testing.T) {
|
|
start, end, err := ParsePortRange("8080")
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if start != 8080 || end != 8080 {
|
|
t.Fatalf("Expected start and end to be the same and equal to 8080, but were %v and %v", start, end)
|
|
}
|
|
}
|
|
|
|
func TestParsePortRangeIncorrectRange(t *testing.T) {
|
|
if _, _, err := ParsePortRange("9000-8080"); err == nil || !strings.Contains(err.Error(), "Invalid range specified for the Port") {
|
|
t.Fatalf("Expecting error 'Invalid range specified for the Port' but received %s.", err)
|
|
}
|
|
}
|
|
|
|
func TestParsePortRangeIncorrectEndRange(t *testing.T) {
|
|
if _, _, err := ParsePortRange("8000-a"); err == nil || !strings.Contains(err.Error(), "invalid syntax") {
|
|
t.Fatalf("Expecting error 'Invalid range specified for the Port' but received %s.", err)
|
|
}
|
|
|
|
if _, _, err := ParsePortRange("8000-30a"); err == nil || !strings.Contains(err.Error(), "invalid syntax") {
|
|
t.Fatalf("Expecting error 'Invalid range specified for the Port' but received %s.", err)
|
|
}
|
|
}
|
|
|
|
func TestParsePortRangeIncorrectStartRange(t *testing.T) {
|
|
if _, _, err := ParsePortRange("a-8000"); err == nil || !strings.Contains(err.Error(), "invalid syntax") {
|
|
t.Fatalf("Expecting error 'Invalid range specified for the Port' but received %s.", err)
|
|
}
|
|
|
|
if _, _, err := ParsePortRange("30a-8000"); err == nil || !strings.Contains(err.Error(), "invalid syntax") {
|
|
t.Fatalf("Expecting error 'Invalid range specified for the Port' but received %s.", err)
|
|
}
|
|
}
|
|
|
|
func TestParseLink(t *testing.T) {
|
|
name, alias, err := ParseLink("name:alias")
|
|
if err != nil {
|
|
t.Fatalf("Expected not to error out on a valid name:alias format but got: %v", err)
|
|
}
|
|
if name != "name" {
|
|
t.Fatalf("Link name should have been name, got %s instead", name)
|
|
}
|
|
if alias != "alias" {
|
|
t.Fatalf("Link alias should have been alias, got %s instead", alias)
|
|
}
|
|
// short format definition
|
|
name, alias, err = ParseLink("name")
|
|
if err != nil {
|
|
t.Fatalf("Expected not to error out on a valid name only format but got: %v", err)
|
|
}
|
|
if name != "name" {
|
|
t.Fatalf("Link name should have been name, got %s instead", name)
|
|
}
|
|
if alias != "name" {
|
|
t.Fatalf("Link alias should have been name, got %s instead", alias)
|
|
}
|
|
// empty string link definition is not allowed
|
|
if _, _, err := ParseLink(""); err == nil || !strings.Contains(err.Error(), "empty string specified for links") {
|
|
t.Fatalf("Expected error 'empty string specified for links' but got: %v", err)
|
|
}
|
|
// more than two colons are not allowed
|
|
if _, _, err := ParseLink("link:alias:wrong"); err == nil || !strings.Contains(err.Error(), "bad format for links: link:alias:wrong") {
|
|
t.Fatalf("Expected error 'bad format for links: link:alias:wrong' but got: %v", err)
|
|
}
|
|
}
|
|
|
|
func TestParseUintList(t *testing.T) {
|
|
valids := map[string]map[int]bool{
|
|
"": {},
|
|
"7": {7: true},
|
|
"1-6": {1: true, 2: true, 3: true, 4: true, 5: true, 6: true},
|
|
"0-7": {0: true, 1: true, 2: true, 3: true, 4: true, 5: true, 6: true, 7: true},
|
|
"0,3-4,7,8-10": {0: true, 3: true, 4: true, 7: true, 8: true, 9: true, 10: true},
|
|
"0-0,0,1-4": {0: true, 1: true, 2: true, 3: true, 4: true},
|
|
"03,1-3": {1: true, 2: true, 3: true},
|
|
"3,2,1": {1: true, 2: true, 3: true},
|
|
"0-2,3,1": {0: true, 1: true, 2: true, 3: true},
|
|
}
|
|
for k, v := range valids {
|
|
out, err := ParseUintList(k)
|
|
if err != nil {
|
|
t.Fatalf("Expected not to fail, got %v", err)
|
|
}
|
|
if !reflect.DeepEqual(out, v) {
|
|
t.Fatalf("Expected %v, got %v", v, out)
|
|
}
|
|
}
|
|
|
|
invalids := []string{
|
|
"this",
|
|
"1--",
|
|
"1-10,,10",
|
|
"10-1",
|
|
"-1",
|
|
"-1,0",
|
|
}
|
|
for _, v := range invalids {
|
|
if out, err := ParseUintList(v); err == nil {
|
|
t.Fatalf("Expected failure with %s but got %v", v, out)
|
|
}
|
|
}
|
|
}
|