mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
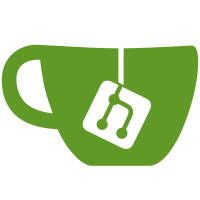
Currently pkg/archive stores nested windows files with backslashes (e.g. `dir\`, `dir\file.txt`) and this causes tar not being correctly extracted on Linux daemon. This change assures we canonicalize all paths to unix paths and add them to tar with that name independent of platform. Fixes the following test cases for Windows CI: - TestBuildAddFileWithWhitespace - TestBuildCopyFileWithWhitespace - TestBuildAddDirContentToRoot - TestBuildAddDirContentToExistingDir - TestBuildCopyDirContentToRoot - TestBuildCopyDirContentToExistDir - TestBuildDockerignore - TestBuildEnvUsage - TestBuildEnvUsage2 Signed-off-by: Ahmet Alp Balkan <ahmetalpbalkan@gmail.com>
42 lines
1,018 B
Go
42 lines
1,018 B
Go
// +build !windows
|
|
|
|
package archive
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
func TestCanonicalTarNameForPath(t *testing.T) {
|
|
cases := []struct{ in, expected string }{
|
|
{"foo", "foo"},
|
|
{"foo/bar", "foo/bar"},
|
|
{"foo/dir/", "foo/dir/"},
|
|
}
|
|
for _, v := range cases {
|
|
if out, err := canonicalTarNameForPath(v.in); err != nil {
|
|
t.Fatalf("cannot get canonical name for path: %s: %v", v.in, err)
|
|
} else if out != v.expected {
|
|
t.Fatalf("wrong canonical tar name. expected:%s got:%s", v.expected, out)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestCanonicalTarName(t *testing.T) {
|
|
cases := []struct {
|
|
in string
|
|
isDir bool
|
|
expected string
|
|
}{
|
|
{"foo", false, "foo"},
|
|
{"foo", true, "foo/"},
|
|
{"foo/bar", false, "foo/bar"},
|
|
{"foo/bar", true, "foo/bar/"},
|
|
}
|
|
for _, v := range cases {
|
|
if out, err := canonicalTarName(v.in, v.isDir); err != nil {
|
|
t.Fatalf("cannot get canonical name for path: %s: %v", v.in, err)
|
|
} else if out != v.expected {
|
|
t.Fatalf("wrong canonical tar name. expected:%s got:%s", v.expected, out)
|
|
}
|
|
}
|
|
}
|