mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
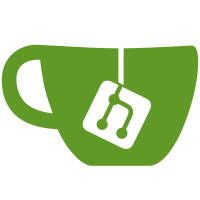
This PR adds a "request ID" to each event generated, the 'docker events' stream now looks like this: ``` 2015-09-10T15:02:50.000000000-07:00 [reqid: c01e3534ddca] de7c5d4ca927253cf4e978ee9c4545161e406e9b5a14617efb52c658b249174a: (from ubuntu) create ``` Note the `[reqID: c01e3534ddca]` part, that's new. Each HTTP request will generate its own unique ID. So, if you do a `docker build` you'll see a series of events all with the same reqID. This allow for log processing tools to determine which events are all related to the same http request. I didn't propigate the context to all possible funcs in the daemon, I decided to just do the ones that needed it in order to get the reqID into the events. I'd like to have people review this direction first, and if we're ok with it then I'll make sure we're consistent about when we pass around the context - IOW, make sure that all funcs at the same level have a context passed in even if they don't call the log funcs - this will ensure we're consistent w/o passing it around for all calls unnecessarily. ping @icecrime @calavera @crosbymichael Signed-off-by: Doug Davis <dug@us.ibm.com>
136 lines
3.3 KiB
Go
136 lines
3.3 KiB
Go
// +build freebsd linux
|
|
|
|
package server
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"net/http"
|
|
"strconv"
|
|
|
|
"github.com/docker/docker/context"
|
|
"github.com/docker/docker/daemon"
|
|
"github.com/docker/docker/pkg/sockets"
|
|
"github.com/docker/libnetwork/portallocator"
|
|
|
|
systemdActivation "github.com/coreos/go-systemd/activation"
|
|
systemdDaemon "github.com/coreos/go-systemd/daemon"
|
|
)
|
|
|
|
// newServer sets up the required serverClosers and does protocol specific checking.
|
|
func (s *Server) newServer(proto, addr string) ([]serverCloser, error) {
|
|
var (
|
|
err error
|
|
ls []net.Listener
|
|
)
|
|
switch proto {
|
|
case "fd":
|
|
ls, err = listenFD(addr)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
// We don't want to start serving on these sockets until the
|
|
// daemon is initialized and installed. Otherwise required handlers
|
|
// won't be ready.
|
|
<-s.start
|
|
case "tcp":
|
|
l, err := s.initTCPSocket(addr)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
ls = append(ls, l)
|
|
case "unix":
|
|
l, err := sockets.NewUnixSocket(addr, s.cfg.SocketGroup, s.start)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
ls = append(ls, l)
|
|
default:
|
|
return nil, fmt.Errorf("Invalid protocol format: %q", proto)
|
|
}
|
|
var res []serverCloser
|
|
for _, l := range ls {
|
|
res = append(res, &HTTPServer{
|
|
&http.Server{
|
|
Addr: addr,
|
|
Handler: s.router,
|
|
},
|
|
l,
|
|
})
|
|
}
|
|
return res, nil
|
|
}
|
|
|
|
// AcceptConnections allows clients to connect to the API server.
|
|
// Referenced Daemon is notified about this server, and waits for the
|
|
// daemon acknowledgement before the incoming connections are accepted.
|
|
func (s *Server) AcceptConnections(ctx context.Context, d *daemon.Daemon) {
|
|
// Tell the init daemon we are accepting requests
|
|
s.daemon = d
|
|
s.registerSubRouter(ctx)
|
|
go systemdDaemon.SdNotify("READY=1")
|
|
// close the lock so the listeners start accepting connections
|
|
select {
|
|
case <-s.start:
|
|
default:
|
|
close(s.start)
|
|
}
|
|
}
|
|
|
|
func allocateDaemonPort(addr string) error {
|
|
host, port, err := net.SplitHostPort(addr)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
intPort, err := strconv.Atoi(port)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
var hostIPs []net.IP
|
|
if parsedIP := net.ParseIP(host); parsedIP != nil {
|
|
hostIPs = append(hostIPs, parsedIP)
|
|
} else if hostIPs, err = net.LookupIP(host); err != nil {
|
|
return fmt.Errorf("failed to lookup %s address in host specification", host)
|
|
}
|
|
|
|
pa := portallocator.Get()
|
|
for _, hostIP := range hostIPs {
|
|
if _, err := pa.RequestPort(hostIP, "tcp", intPort); err != nil {
|
|
return fmt.Errorf("failed to allocate daemon listening port %d (err: %v)", intPort, err)
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// listenFD returns the specified socket activated files as a slice of
|
|
// net.Listeners or all of the activated files if "*" is given.
|
|
func listenFD(addr string) ([]net.Listener, error) {
|
|
// socket activation
|
|
listeners, err := systemdActivation.Listeners(false)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if listeners == nil || len(listeners) == 0 {
|
|
return nil, fmt.Errorf("No sockets found")
|
|
}
|
|
|
|
// default to all fds just like unix:// and tcp://
|
|
if addr == "" {
|
|
addr = "*"
|
|
}
|
|
|
|
fdNum, _ := strconv.Atoi(addr)
|
|
fdOffset := fdNum - 3
|
|
if (addr != "*") && (len(listeners) < int(fdOffset)+1) {
|
|
return nil, fmt.Errorf("Too few socket activated files passed in")
|
|
}
|
|
|
|
if addr == "*" {
|
|
return listeners, nil
|
|
}
|
|
|
|
return []net.Listener{listeners[fdOffset]}, nil
|
|
}
|