mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
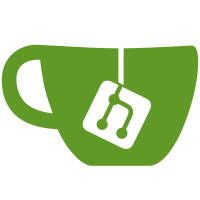
This moves validation of options to the start of the Create function to prevent hitting the filesystem and having to remove the volume from disk. Also addressing some minor nits w.r.t. errors. Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
53 lines
1.1 KiB
Go
53 lines
1.1 KiB
Go
// Package local provides the default implementation for volumes. It
|
|
// is used to mount data volume containers and directories local to
|
|
// the host server.
|
|
package local // import "github.com/docker/docker/volume/local"
|
|
|
|
import (
|
|
"os"
|
|
"syscall"
|
|
"time"
|
|
|
|
"github.com/docker/docker/errdefs"
|
|
"github.com/pkg/errors"
|
|
)
|
|
|
|
type optsConfig struct{}
|
|
|
|
func (v *localVolume) setOpts(opts map[string]string) error {
|
|
// Windows does not support any options currently
|
|
return nil
|
|
}
|
|
|
|
func validateOpts(opts map[string]string) error {
|
|
if len(opts) > 0 {
|
|
return errdefs.InvalidParameter(errors.New("options are not supported on this platform"))
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (v *localVolume) needsMount() bool {
|
|
return false
|
|
}
|
|
|
|
func (v *localVolume) mount() error {
|
|
return nil
|
|
}
|
|
func (v *localVolume) unmount() error {
|
|
return nil
|
|
}
|
|
|
|
func unmount(_ string) {}
|
|
|
|
func (v *localVolume) postMount() error {
|
|
return nil
|
|
}
|
|
|
|
func (v *localVolume) CreatedAt() (time.Time, error) {
|
|
fileInfo, err := os.Stat(v.path)
|
|
if err != nil {
|
|
return time.Time{}, err
|
|
}
|
|
ft := fileInfo.Sys().(*syscall.Win32FileAttributeData).CreationTime
|
|
return time.Unix(0, ft.Nanoseconds()), nil
|
|
}
|