mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
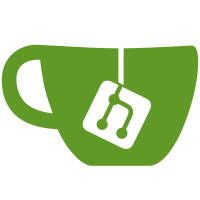
The `docker info` command compares the installed version of containerd using a Git-sha. We currently use a tag for this, but that tag is not returned by the version-API of containerd, resulting in the `docker info` output to show: containerd version: 89623f28b87a6004d4b785663257362d1658a729 (expected: v1.0.0) This patch changes the `v1.0.0` tag to the commit that corresponds with the tag, so that the `docker info` output does not show the `expected:` string. This should be considered a temporary workaround; the check for the exact version of containerd that's installed was needed when we still used the 0.2.x branch, because it did not have stable releases yet. With containerd reaching 1.0, and using SemVer, we can likely do a comparison for "Major" version, or make this a "packaging" issue, and remove the check entirely (we can still _print_ the version that's installed if we think it's usefule). Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
34 lines
1.1 KiB
Go
34 lines
1.1 KiB
Go
// +build !windows
|
|
|
|
package system
|
|
|
|
import (
|
|
"testing"
|
|
|
|
"github.com/docker/docker/integration/util/request"
|
|
"github.com/stretchr/testify/assert"
|
|
"github.com/stretchr/testify/require"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
func TestInfo_BinaryCommits(t *testing.T) {
|
|
client := request.NewAPIClient(t)
|
|
|
|
info, err := client.Info(context.Background())
|
|
require.NoError(t, err)
|
|
|
|
assert.NotNil(t, info.ContainerdCommit)
|
|
assert.NotEqual(t, "N/A", info.ContainerdCommit.ID)
|
|
assert.Equal(t, testEnv.DaemonInfo.ContainerdCommit.Expected, info.ContainerdCommit.Expected)
|
|
assert.Equal(t, info.ContainerdCommit.Expected, info.ContainerdCommit.ID)
|
|
|
|
assert.NotNil(t, info.InitCommit)
|
|
assert.NotEqual(t, "N/A", info.InitCommit.ID)
|
|
assert.Equal(t, testEnv.DaemonInfo.InitCommit.Expected, info.InitCommit.Expected)
|
|
assert.Equal(t, info.InitCommit.Expected, info.InitCommit.ID)
|
|
|
|
assert.NotNil(t, info.RuncCommit)
|
|
assert.NotEqual(t, "N/A", info.RuncCommit.ID)
|
|
assert.Equal(t, testEnv.DaemonInfo.RuncCommit.Expected, info.RuncCommit.Expected)
|
|
assert.Equal(t, info.RuncCommit.Expected, info.RuncCommit.ID)
|
|
}
|