mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
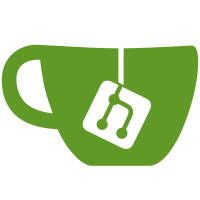
Docker-DCO-1.1-Signed-off-by: Michael Crosby <michael@crosbymichael.com> (github: crosbymichael)
46 lines
1.2 KiB
Go
46 lines
1.2 KiB
Go
package runconfig
|
|
|
|
import (
|
|
"github.com/dotcloud/docker/engine"
|
|
"github.com/dotcloud/docker/nat"
|
|
"github.com/dotcloud/docker/utils"
|
|
)
|
|
|
|
type HostConfig struct {
|
|
Binds []string
|
|
ContainerIDFile string
|
|
LxcConf []utils.KeyValuePair
|
|
Privileged bool
|
|
PortBindings nat.PortMap
|
|
Links []string
|
|
PublishAllPorts bool
|
|
Dns []string
|
|
DnsSearch []string
|
|
VolumesFrom []string
|
|
}
|
|
|
|
func ContainerHostConfigFromJob(job *engine.Job) *HostConfig {
|
|
hostConfig := &HostConfig{
|
|
ContainerIDFile: job.Getenv("ContainerIDFile"),
|
|
Privileged: job.GetenvBool("Privileged"),
|
|
PublishAllPorts: job.GetenvBool("PublishAllPorts"),
|
|
}
|
|
job.GetenvJson("LxcConf", &hostConfig.LxcConf)
|
|
job.GetenvJson("PortBindings", &hostConfig.PortBindings)
|
|
if Binds := job.GetenvList("Binds"); Binds != nil {
|
|
hostConfig.Binds = Binds
|
|
}
|
|
if Links := job.GetenvList("Links"); Links != nil {
|
|
hostConfig.Links = Links
|
|
}
|
|
if Dns := job.GetenvList("Dns"); Dns != nil {
|
|
hostConfig.Dns = Dns
|
|
}
|
|
if DnsSearch := job.GetenvList("DnsSearch"); DnsSearch != nil {
|
|
hostConfig.DnsSearch = DnsSearch
|
|
}
|
|
if VolumesFrom := job.GetenvList("VolumesFrom"); VolumesFrom != nil {
|
|
hostConfig.VolumesFrom = VolumesFrom
|
|
}
|
|
return hostConfig
|
|
}
|