mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
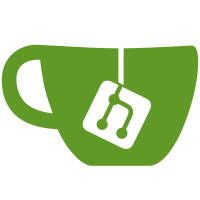
Creates a `fixedBuffer` type that is used to encapsulate functionality for reading/writing from the underlying byte slices. Uses lazily-loaded set of sync.Pools for storing buffers that are no longer needed so they can be re-used. ``` benchmark old ns/op new ns/op delta BenchmarkBytesPipeWrite-8 138469 48985 -64.62% BenchmarkBytesPipeRead-8 130922 56601 -56.77% benchmark old allocs new allocs delta BenchmarkBytesPipeWrite-8 18 8 -55.56% BenchmarkBytesPipeRead-8 0 0 +0.00% benchmark old bytes new bytes delta BenchmarkBytesPipeWrite-8 66903 1649 -97.54% BenchmarkBytesPipeRead-8 0 1 +Inf% ``` Signed-off-by: Brian Goff <cpuguy83@gmail.com>
51 lines
808 B
Go
51 lines
808 B
Go
package ioutils
|
|
|
|
import (
|
|
"errors"
|
|
"io"
|
|
)
|
|
|
|
var errBufferFull = errors.New("buffer is full")
|
|
|
|
type fixedBuffer struct {
|
|
buf []byte
|
|
pos int
|
|
lastRead int
|
|
}
|
|
|
|
func (b *fixedBuffer) Write(p []byte) (int, error) {
|
|
n := copy(b.buf[b.pos:cap(b.buf)], p)
|
|
b.pos += n
|
|
|
|
if n < len(p) {
|
|
if b.pos == cap(b.buf) {
|
|
return n, errBufferFull
|
|
}
|
|
return n, io.ErrShortWrite
|
|
}
|
|
return n, nil
|
|
}
|
|
|
|
func (b *fixedBuffer) Read(p []byte) (int, error) {
|
|
n := copy(p, b.buf[b.lastRead:b.pos])
|
|
b.lastRead += n
|
|
return n, nil
|
|
}
|
|
|
|
func (b *fixedBuffer) Len() int {
|
|
return b.pos - b.lastRead
|
|
}
|
|
|
|
func (b *fixedBuffer) Cap() int {
|
|
return cap(b.buf)
|
|
}
|
|
|
|
func (b *fixedBuffer) Reset() {
|
|
b.pos = 0
|
|
b.lastRead = 0
|
|
b.buf = b.buf[:0]
|
|
}
|
|
|
|
func (b *fixedBuffer) String() string {
|
|
return string(b.buf[b.lastRead:b.pos])
|
|
}
|