mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
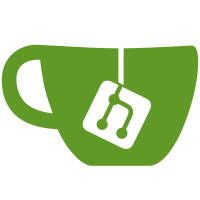
We now have one place that keeps track of (most) devices that are allowed and created within the container. That place is pkg/libcontainer/devices/devices.go This fixes several inconsistencies between which devices were created in the lxc backend and the native backend. It also fixes inconsistencies between wich devices were created and which were allowed. For example, /dev/full was being created but it was not allowed within the cgroup. It also declares the file modes and permissions of the default devices, rather than copying them from the host. This is in line with docker's philosphy of not being host dependent. Docker-DCO-1.1-Signed-off-by: Timothy Hobbs <timothyhobbs@seznam.cz> (github: https://github.com/timthelion)
26 lines
1,022 B
Go
26 lines
1,022 B
Go
package devices
|
|
|
|
/*
|
|
|
|
This code provides support for manipulating linux device numbers. It should be replaced by normal syscall functions once http://code.google.com/p/go/issues/detail?id=8106 is solved.
|
|
|
|
You can read what they are here:
|
|
|
|
- http://www.makelinux.net/ldd3/chp-3-sect-2
|
|
- http://www.linux-tutorial.info/modules.php?name=MContent&pageid=94
|
|
|
|
Note! These are NOT the same as the MAJOR(dev_t device);, MINOR(dev_t device); and MKDEV(int major, int minor); functions as defined in <linux/kdev_t.h> as the representation of device numbers used by go is different than the one used internally to the kernel! - https://github.com/torvalds/linux/blob/master/include/linux/kdev_t.h#L9
|
|
|
|
*/
|
|
|
|
func Major(devNumber int) int64 {
|
|
return int64((devNumber >> 8) & 0xfff)
|
|
}
|
|
|
|
func Minor(devNumber int) int64 {
|
|
return int64((devNumber & 0xff) | ((devNumber >> 12) & 0xfff00))
|
|
}
|
|
|
|
func Mkdev(majorNumber int64, minorNumber int64) int {
|
|
return int((majorNumber << 8) | (minorNumber & 0xff) | ((minorNumber & 0xfff00) << 12))
|
|
}
|