mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
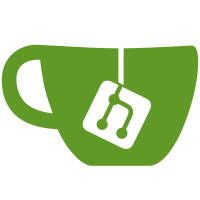
- Add IPAM cotract and remote IPAM hooks - Add ipam registration in controller - Have default IPAM follow ipamapi contract Signed-off-by: Alessandro Boch <aboch@docker.com>
81 lines
2.1 KiB
Go
81 lines
2.1 KiB
Go
// Package api defines the data structure to be used in the request/response
|
|
// messages between libnetwork and the remote ipam plugin
|
|
package api
|
|
|
|
import (
|
|
"net"
|
|
)
|
|
|
|
// Response is the basic response structure used in all responses
|
|
type Response struct {
|
|
Error string
|
|
}
|
|
|
|
// IsSuccess returns wheter the plugin response is successful
|
|
func (r *Response) IsSuccess() bool {
|
|
return r.Error == ""
|
|
}
|
|
|
|
// GetError returns the error from the response, if any.
|
|
func (r *Response) GetError() string {
|
|
return r.Error
|
|
}
|
|
|
|
// GetAddressSpacesResponse is the response to the ``get default address spaces`` request message
|
|
type GetAddressSpacesResponse struct {
|
|
Response
|
|
LocalDefaultAddressSpace string
|
|
GlobalDefaultAddressSpace string
|
|
}
|
|
|
|
// RequestPoolRequest represents the expected data in a ``request address pool`` request message
|
|
type RequestPoolRequest struct {
|
|
AddressSpace string
|
|
Pool string
|
|
SubPool string
|
|
Options map[string]string
|
|
V6 bool
|
|
}
|
|
|
|
// RequestPoolResponse represents the response message to a ``request address pool`` request
|
|
type RequestPoolResponse struct {
|
|
Response
|
|
PoolID string
|
|
Pool *net.IPNet
|
|
Data map[string]string
|
|
}
|
|
|
|
// ReleasePoolRequest represents the expected data in a ``release address pool`` request message
|
|
type ReleasePoolRequest struct {
|
|
PoolID string
|
|
}
|
|
|
|
// ReleasePoolResponse represents the response message to a ``release address pool`` request
|
|
type ReleasePoolResponse struct {
|
|
Response
|
|
}
|
|
|
|
// RequestAddressRequest represents the expected data in a ``request address`` request message
|
|
type RequestAddressRequest struct {
|
|
PoolID string
|
|
Address net.IP
|
|
Options map[string]string
|
|
}
|
|
|
|
// RequestAddressResponse represents the expected data in the response message to a ``request address`` request
|
|
type RequestAddressResponse struct {
|
|
Response
|
|
Address *net.IPNet
|
|
Data map[string]string
|
|
}
|
|
|
|
// ReleaseAddressRequest represents the expected data in a ``release address`` request message
|
|
type ReleaseAddressRequest struct {
|
|
PoolID string
|
|
Address net.IP
|
|
}
|
|
|
|
// ReleaseAddressResponse represents the response message to a ``release address`` request
|
|
type ReleaseAddressResponse struct {
|
|
Response
|
|
}
|