mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
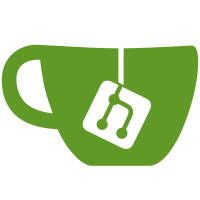
Cleanup cobra integration Update windows files for cobra and pflags Cleanup SetupRootcmd, and remove unnecessary SetFlagErrorFunc. Use cobra command traversal Signed-off-by: Daniel Nephin <dnephin@docker.com>
49 lines
1.1 KiB
Go
49 lines
1.1 KiB
Go
package container
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/docker/docker/api/client"
|
|
"github.com/docker/docker/cli"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
type unpauseOptions struct {
|
|
containers []string
|
|
}
|
|
|
|
// NewUnpauseCommand creates a new cobra.Command for `docker unpause`
|
|
func NewUnpauseCommand(dockerCli *client.DockerCli) *cobra.Command {
|
|
var opts unpauseOptions
|
|
|
|
cmd := &cobra.Command{
|
|
Use: "unpause CONTAINER [CONTAINER...]",
|
|
Short: "Unpause all processes within one or more containers",
|
|
Args: cli.RequiresMinArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
opts.containers = args
|
|
return runUnpause(dockerCli, &opts)
|
|
},
|
|
}
|
|
return cmd
|
|
}
|
|
|
|
func runUnpause(dockerCli *client.DockerCli, opts *unpauseOptions) error {
|
|
ctx := context.Background()
|
|
|
|
var errs []string
|
|
for _, container := range opts.containers {
|
|
if err := dockerCli.Client().ContainerUnpause(ctx, container); err != nil {
|
|
errs = append(errs, err.Error())
|
|
} else {
|
|
fmt.Fprintf(dockerCli.Out(), "%s\n", container)
|
|
}
|
|
}
|
|
if len(errs) > 0 {
|
|
return fmt.Errorf("%s", strings.Join(errs, "\n"))
|
|
}
|
|
return nil
|
|
}
|