mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
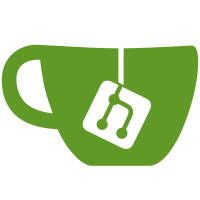
After the new libcontainer API, the reexec.Self() output of the daemon binary is used as the libcontainer factory InitPath. If it is relative, it can't be found at container start time. This patch solves the problem by making sure that we return a rooted/absolute path if a relative path is used. Docker-DCO-1.1-Signed-off-by: Phil Estes <estesp@linux.vnet.ibm.com> (github: estesp)
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
package reexec
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"os/exec"
|
|
"path/filepath"
|
|
)
|
|
|
|
var registeredInitializers = make(map[string]func())
|
|
|
|
// Register adds an initialization func under the specified name
|
|
func Register(name string, initializer func()) {
|
|
if _, exists := registeredInitializers[name]; exists {
|
|
panic(fmt.Sprintf("reexec func already registred under name %q", name))
|
|
}
|
|
|
|
registeredInitializers[name] = initializer
|
|
}
|
|
|
|
// Init is called as the first part of the exec process and returns true if an
|
|
// initialization function was called.
|
|
func Init() bool {
|
|
initializer, exists := registeredInitializers[os.Args[0]]
|
|
if exists {
|
|
initializer()
|
|
|
|
return true
|
|
}
|
|
return false
|
|
}
|
|
|
|
// Self returns the path to the current processes binary
|
|
func Self() string {
|
|
name := os.Args[0]
|
|
if filepath.Base(name) == name {
|
|
if lp, err := exec.LookPath(name); err == nil {
|
|
return lp
|
|
}
|
|
}
|
|
// handle conversion of relative paths to absolute
|
|
if absName, err := filepath.Abs(name); err == nil {
|
|
return absName
|
|
}
|
|
// if we coudn't get absolute name, return original
|
|
// (NOTE: Go only errors on Abs() if os.Getwd fails)
|
|
return name
|
|
}
|