mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
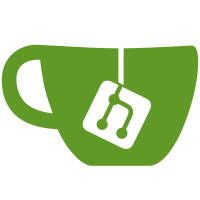
This enables image lookup when creating a container to fail when the reference exists but it is for the wrong platform. This prevents trying to run an image for the wrong platform, as can be the case with, for example binfmt_misc+qemu. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
72 lines
2.2 KiB
Go
72 lines
2.2 KiB
Go
package container
|
|
|
|
import (
|
|
"context"
|
|
"runtime"
|
|
"testing"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/container"
|
|
"github.com/docker/docker/api/types/network"
|
|
"github.com/docker/docker/client"
|
|
specs "github.com/opencontainers/image-spec/specs-go/v1"
|
|
"gotest.tools/v3/assert"
|
|
)
|
|
|
|
// TestContainerConfig holds container configuration struct that
|
|
// are used in api calls.
|
|
type TestContainerConfig struct {
|
|
Name string
|
|
Config *container.Config
|
|
HostConfig *container.HostConfig
|
|
NetworkingConfig *network.NetworkingConfig
|
|
Platform *specs.Platform
|
|
}
|
|
|
|
// create creates a container with the specified options
|
|
func create(ctx context.Context, t *testing.T, client client.APIClient, ops ...func(*TestContainerConfig)) (container.ContainerCreateCreatedBody, error) {
|
|
t.Helper()
|
|
cmd := []string{"top"}
|
|
if runtime.GOOS == "windows" {
|
|
cmd = []string{"sleep", "240"}
|
|
}
|
|
config := &TestContainerConfig{
|
|
Config: &container.Config{
|
|
Image: "busybox",
|
|
Cmd: cmd,
|
|
},
|
|
HostConfig: &container.HostConfig{},
|
|
NetworkingConfig: &network.NetworkingConfig{},
|
|
}
|
|
|
|
for _, op := range ops {
|
|
op(config)
|
|
}
|
|
|
|
return client.ContainerCreate(ctx, config.Config, config.HostConfig, config.NetworkingConfig, config.Platform, config.Name)
|
|
}
|
|
|
|
// Create creates a container with the specified options, asserting that there was no error
|
|
func Create(ctx context.Context, t *testing.T, client client.APIClient, ops ...func(*TestContainerConfig)) string {
|
|
c, err := create(ctx, t, client, ops...)
|
|
assert.NilError(t, err)
|
|
|
|
return c.ID
|
|
}
|
|
|
|
// CreateExpectingErr creates a container, expecting an error with the specified message
|
|
func CreateExpectingErr(ctx context.Context, t *testing.T, client client.APIClient, errMsg string, ops ...func(*TestContainerConfig)) {
|
|
_, err := create(ctx, t, client, ops...)
|
|
assert.ErrorContains(t, err, errMsg)
|
|
}
|
|
|
|
// Run creates and start a container with the specified options
|
|
func Run(ctx context.Context, t *testing.T, client client.APIClient, ops ...func(*TestContainerConfig)) string {
|
|
t.Helper()
|
|
id := Create(ctx, t, client, ops...)
|
|
|
|
err := client.ContainerStart(ctx, id, types.ContainerStartOptions{})
|
|
assert.NilError(t, err)
|
|
|
|
return id
|
|
}
|