mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
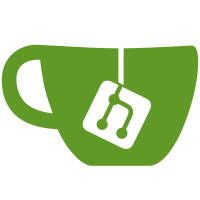
We're already using testenv here, so might as well use the exact same function that it provides. Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
42 lines
1.2 KiB
Go
42 lines
1.2 KiB
Go
package network
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/client"
|
|
"gotest.tools/v3/assert/cmp"
|
|
)
|
|
|
|
// IsNetworkAvailable provides a comparison to check if a docker network is available
|
|
func IsNetworkAvailable(c client.NetworkAPIClient, name string) cmp.Comparison {
|
|
return func() cmp.Result {
|
|
networks, err := c.NetworkList(context.Background(), types.NetworkListOptions{})
|
|
if err != nil {
|
|
return cmp.ResultFromError(err)
|
|
}
|
|
for _, network := range networks {
|
|
if network.Name == name {
|
|
return cmp.ResultSuccess
|
|
}
|
|
}
|
|
return cmp.ResultFailure(fmt.Sprintf("could not find network %s", name))
|
|
}
|
|
}
|
|
|
|
// IsNetworkNotAvailable provides a comparison to check if a docker network is not available
|
|
func IsNetworkNotAvailable(c client.NetworkAPIClient, name string) cmp.Comparison {
|
|
return func() cmp.Result {
|
|
networks, err := c.NetworkList(context.Background(), types.NetworkListOptions{})
|
|
if err != nil {
|
|
return cmp.ResultFromError(err)
|
|
}
|
|
for _, network := range networks {
|
|
if network.Name == name {
|
|
return cmp.ResultFailure(fmt.Sprintf("network %s is still present", name))
|
|
}
|
|
}
|
|
return cmp.ResultSuccess
|
|
}
|
|
}
|