mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
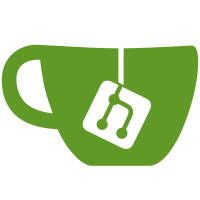
Includes: - https://github.com/docker/swarmkit/pull/1766 - https://github.com/docker/swarmkit/pull/1770 Signed-off-by: Andrea Luzzardi <aluzzardi@gmail.com>
4263 lines
110 KiB
Go
4263 lines
110 KiB
Go
// Code generated by protoc-gen-gogo.
|
|
// source: objects.proto
|
|
// DO NOT EDIT!
|
|
|
|
package api
|
|
|
|
import proto "github.com/gogo/protobuf/proto"
|
|
import fmt "fmt"
|
|
import math "math"
|
|
import docker_swarmkit_v1 "github.com/docker/swarmkit/api/timestamp"
|
|
import _ "github.com/gogo/protobuf/gogoproto"
|
|
|
|
import strings "strings"
|
|
import github_com_gogo_protobuf_proto "github.com/gogo/protobuf/proto"
|
|
import sort "sort"
|
|
import strconv "strconv"
|
|
import reflect "reflect"
|
|
import github_com_gogo_protobuf_sortkeys "github.com/gogo/protobuf/sortkeys"
|
|
|
|
import io "io"
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// Meta contains metadata about objects. Every object contains a meta field.
|
|
type Meta struct {
|
|
// Version tracks the current version of the object.
|
|
Version Version `protobuf:"bytes,1,opt,name=version" json:"version"`
|
|
// Object timestamps.
|
|
CreatedAt *docker_swarmkit_v1.Timestamp `protobuf:"bytes,2,opt,name=created_at,json=createdAt" json:"created_at,omitempty"`
|
|
UpdatedAt *docker_swarmkit_v1.Timestamp `protobuf:"bytes,3,opt,name=updated_at,json=updatedAt" json:"updated_at,omitempty"`
|
|
}
|
|
|
|
func (m *Meta) Reset() { *m = Meta{} }
|
|
func (*Meta) ProtoMessage() {}
|
|
func (*Meta) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{0} }
|
|
|
|
// Node provides the internal node state as seen by the cluster.
|
|
type Node struct {
|
|
// ID specifies the identity of the node.
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec defines the desired state of the node as specified by the user.
|
|
// The system will honor this and will *never* modify it.
|
|
Spec NodeSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// Description encapsulated the properties of the Node as reported by the
|
|
// agent.
|
|
Description *NodeDescription `protobuf:"bytes,4,opt,name=description" json:"description,omitempty"`
|
|
// Status provides the current status of the node, as seen by the manager.
|
|
Status NodeStatus `protobuf:"bytes,5,opt,name=status" json:"status"`
|
|
// ManagerStatus provides the current status of the node's manager
|
|
// component, if the node is a manager.
|
|
ManagerStatus *ManagerStatus `protobuf:"bytes,6,opt,name=manager_status,json=managerStatus" json:"manager_status,omitempty"`
|
|
// The node attachment to the ingress network.
|
|
Attachment *NetworkAttachment `protobuf:"bytes,7,opt,name=attachment" json:"attachment,omitempty"`
|
|
// Certificate is the TLS certificate issued for the node, if any.
|
|
Certificate Certificate `protobuf:"bytes,8,opt,name=certificate" json:"certificate"`
|
|
}
|
|
|
|
func (m *Node) Reset() { *m = Node{} }
|
|
func (*Node) ProtoMessage() {}
|
|
func (*Node) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{1} }
|
|
|
|
type Service struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Spec ServiceSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// PreviousSpec is the previous service spec that was in place before
|
|
// "Spec".
|
|
PreviousSpec *ServiceSpec `protobuf:"bytes,6,opt,name=previous_spec,json=previousSpec" json:"previous_spec,omitempty"`
|
|
// Runtime state of service endpoint. This may be different
|
|
// from the spec version because the user may not have entered
|
|
// the optional fields like node_port or virtual_ip and it
|
|
// could be auto allocated by the system.
|
|
Endpoint *Endpoint `protobuf:"bytes,4,opt,name=endpoint" json:"endpoint,omitempty"`
|
|
// UpdateStatus contains the status of an update, if one is in
|
|
// progress.
|
|
UpdateStatus *UpdateStatus `protobuf:"bytes,5,opt,name=update_status,json=updateStatus" json:"update_status,omitempty"`
|
|
}
|
|
|
|
func (m *Service) Reset() { *m = Service{} }
|
|
func (*Service) ProtoMessage() {}
|
|
func (*Service) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{2} }
|
|
|
|
// Endpoint specified all the network parameters required to
|
|
// correctly discover and load balance a service
|
|
type Endpoint struct {
|
|
Spec *EndpointSpec `protobuf:"bytes,1,opt,name=spec" json:"spec,omitempty"`
|
|
// Runtime state of the exposed ports which may carry
|
|
// auto-allocated swarm ports in addition to the user
|
|
// configured information.
|
|
Ports []*PortConfig `protobuf:"bytes,2,rep,name=ports" json:"ports,omitempty"`
|
|
// VirtualIPs specifies the IP addresses under which this endpoint will be
|
|
// made available.
|
|
VirtualIPs []*Endpoint_VirtualIP `protobuf:"bytes,3,rep,name=virtual_ips,json=virtualIps" json:"virtual_ips,omitempty"`
|
|
}
|
|
|
|
func (m *Endpoint) Reset() { *m = Endpoint{} }
|
|
func (*Endpoint) ProtoMessage() {}
|
|
func (*Endpoint) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{3} }
|
|
|
|
// VirtualIP specifies a set of networks this endpoint will be attached to
|
|
// and the IP addresses the target service will be made available under.
|
|
type Endpoint_VirtualIP struct {
|
|
// NetworkID for which this endpoint attachment was created.
|
|
NetworkID string `protobuf:"bytes,1,opt,name=network_id,json=networkId,proto3" json:"network_id,omitempty"`
|
|
// A virtual IP is used to address this service in IP
|
|
// layer that the client can use to send requests to
|
|
// this service. A DNS A/AAAA query on the service
|
|
// name might return this IP to the client. This is
|
|
// strictly a logical IP and there may not be any
|
|
// interfaces assigned this IP address or any route
|
|
// created for this address. More than one to
|
|
// accomodate for both IPv4 and IPv6
|
|
Addr string `protobuf:"bytes,2,opt,name=addr,proto3" json:"addr,omitempty"`
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Reset() { *m = Endpoint_VirtualIP{} }
|
|
func (*Endpoint_VirtualIP) ProtoMessage() {}
|
|
func (*Endpoint_VirtualIP) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{3, 0} }
|
|
|
|
// Task specifies the parameters for implementing a Spec. A task is effectively
|
|
// immutable and idempotent. Once it is dispatched to a node, it will not be
|
|
// dispatched to another node.
|
|
type Task struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec defines the desired state of the task as specified by the user.
|
|
// The system will honor this and will *never* modify it.
|
|
Spec TaskSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// ServiceID indicates the service under which this task is orchestrated. This
|
|
// should almost always be set.
|
|
ServiceID string `protobuf:"bytes,4,opt,name=service_id,json=serviceId,proto3" json:"service_id,omitempty"`
|
|
// Slot is the service slot number for a task.
|
|
// For example, if a replicated service has replicas = 2, there will be a
|
|
// task with slot = 1, and another with slot = 2.
|
|
Slot uint64 `protobuf:"varint,5,opt,name=slot,proto3" json:"slot,omitempty"`
|
|
// NodeID indicates the node to which the task is assigned. If this field
|
|
// is empty or not set, the task is unassigned.
|
|
NodeID string `protobuf:"bytes,6,opt,name=node_id,json=nodeId,proto3" json:"node_id,omitempty"`
|
|
// Annotations defines the names and labels for the runtime, as set by
|
|
// the cluster manager.
|
|
//
|
|
// As backup, if this field has an empty name, the runtime will
|
|
// allocate a unique name for the actual container.
|
|
//
|
|
// NOTE(stevvooe): The preserves the ability for us to making naming
|
|
// decisions for tasks in orchestrator, albeit, this is left empty for now.
|
|
Annotations Annotations `protobuf:"bytes,7,opt,name=annotations" json:"annotations"`
|
|
// ServiceAnnotations is a direct copy of the service name and labels when
|
|
// this task is created.
|
|
//
|
|
// Labels set here will *not* be propagated to the runtime target, such as a
|
|
// container. Use labels on the runtime target for that purpose.
|
|
ServiceAnnotations Annotations `protobuf:"bytes,8,opt,name=service_annotations,json=serviceAnnotations" json:"service_annotations"`
|
|
Status TaskStatus `protobuf:"bytes,9,opt,name=status" json:"status"`
|
|
// DesiredState is the target state for the task. It is set to
|
|
// TaskStateRunning when a task is first created, and changed to
|
|
// TaskStateShutdown if the manager wants to terminate the task. This field
|
|
// is only written by the manager.
|
|
DesiredState TaskState `protobuf:"varint,10,opt,name=desired_state,json=desiredState,proto3,enum=docker.swarmkit.v1.TaskState" json:"desired_state,omitempty"`
|
|
// List of network attachments by the task.
|
|
Networks []*NetworkAttachment `protobuf:"bytes,11,rep,name=networks" json:"networks,omitempty"`
|
|
// A copy of runtime state of service endpoint from Service
|
|
// object to be distributed to agents as part of the task.
|
|
Endpoint *Endpoint `protobuf:"bytes,12,opt,name=endpoint" json:"endpoint,omitempty"`
|
|
// LogDriver specifies the selected log driver to use for the task. Agent
|
|
// processes should always favor the value in this field.
|
|
//
|
|
// If present in the TaskSpec, this will be a copy of that value. The
|
|
// orchestrator may choose to insert a value here, which should be honored,
|
|
// such a cluster default or policy-based value.
|
|
//
|
|
// If not present, the daemon's default will be used.
|
|
LogDriver *Driver `protobuf:"bytes,13,opt,name=log_driver,json=logDriver" json:"log_driver,omitempty"`
|
|
}
|
|
|
|
func (m *Task) Reset() { *m = Task{} }
|
|
func (*Task) ProtoMessage() {}
|
|
func (*Task) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{4} }
|
|
|
|
// NetworkAttachment specifies the network parameters of attachment to
|
|
// a single network by an object such as task or node.
|
|
type NetworkAttachment struct {
|
|
// Network state as a whole becomes part of the object so that
|
|
// it always is available for use in agents so that agents
|
|
// don't have any other dependency during execution.
|
|
Network *Network `protobuf:"bytes,1,opt,name=network" json:"network,omitempty"`
|
|
// List of IPv4/IPv6 addresses that are assigned to the object
|
|
// as part of getting attached to this network.
|
|
Addresses []string `protobuf:"bytes,2,rep,name=addresses" json:"addresses,omitempty"`
|
|
// List of aliases by which a task is resolved in a network
|
|
Aliases []string `protobuf:"bytes,3,rep,name=aliases" json:"aliases,omitempty"`
|
|
}
|
|
|
|
func (m *NetworkAttachment) Reset() { *m = NetworkAttachment{} }
|
|
func (*NetworkAttachment) ProtoMessage() {}
|
|
func (*NetworkAttachment) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{5} }
|
|
|
|
type Network struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Spec NetworkSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// Driver specific operational state provided by the network driver.
|
|
DriverState *Driver `protobuf:"bytes,4,opt,name=driver_state,json=driverState" json:"driver_state,omitempty"`
|
|
// Runtime state of IPAM options. This may not reflect the
|
|
// ipam options from NetworkSpec.
|
|
IPAM *IPAMOptions `protobuf:"bytes,5,opt,name=ipam" json:"ipam,omitempty"`
|
|
}
|
|
|
|
func (m *Network) Reset() { *m = Network{} }
|
|
func (*Network) ProtoMessage() {}
|
|
func (*Network) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{6} }
|
|
|
|
// Cluster provides global cluster settings.
|
|
type Cluster struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Spec ClusterSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// RootCA contains key material for the root CA.
|
|
RootCA RootCA `protobuf:"bytes,4,opt,name=root_ca,json=rootCa" json:"root_ca"`
|
|
// Symmetric encryption key distributed by the lead manager. Used by agents
|
|
// for securing network bootstrapping and communication.
|
|
NetworkBootstrapKeys []*EncryptionKey `protobuf:"bytes,5,rep,name=network_bootstrap_keys,json=networkBootstrapKeys" json:"network_bootstrap_keys,omitempty"`
|
|
// Logical clock used to timestamp every key. It allows other managers
|
|
// and agents to unambiguously identify the older key to be deleted when
|
|
// a new key is allocated on key rotation.
|
|
EncryptionKeyLamportClock uint64 `protobuf:"varint,6,opt,name=encryption_key_lamport_clock,json=encryptionKeyLamportClock,proto3" json:"encryption_key_lamport_clock,omitempty"`
|
|
// BlacklistedCertificates tracks certificates that should no longer
|
|
// be honored. It's a mapping from CN -> BlacklistedCertificate.
|
|
// swarm. Their certificates should effectively be blacklisted.
|
|
BlacklistedCertificates map[string]*BlacklistedCertificate `protobuf:"bytes,8,rep,name=blacklisted_certificates,json=blacklistedCertificates" json:"blacklisted_certificates,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value"`
|
|
// UnlockKeys defines the keys that lock node data at rest. For example,
|
|
// this would contain the key encrypting key (KEK) that will encrypt the
|
|
// manager TLS keys at rest and the raft encryption keys at rest.
|
|
// If the key is empty, the node will be unlocked (will not require a key
|
|
// to start up from a shut down state).
|
|
UnlockKeys []*EncryptionKey `protobuf:"bytes,9,rep,name=unlock_keys,json=unlockKeys" json:"unlock_keys,omitempty"`
|
|
}
|
|
|
|
func (m *Cluster) Reset() { *m = Cluster{} }
|
|
func (*Cluster) ProtoMessage() {}
|
|
func (*Cluster) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{7} }
|
|
|
|
// Secret represents a secret that should be passed to a container or a node,
|
|
// and is immutable. It wraps the `spec` provided by the user with useful
|
|
// information that is generated from the secret data in the `spec`, such as
|
|
// the digest and size of the secret data.
|
|
type Secret struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec contains the actual secret data, as well as any context around the
|
|
// secret data that the user provides.
|
|
Spec SecretSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// Whether the secret is an internal secret (not set by a user) or not.
|
|
Internal bool `protobuf:"varint,4,opt,name=internal,proto3" json:"internal,omitempty"`
|
|
}
|
|
|
|
func (m *Secret) Reset() { *m = Secret{} }
|
|
func (*Secret) ProtoMessage() {}
|
|
func (*Secret) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{8} }
|
|
|
|
func init() {
|
|
proto.RegisterType((*Meta)(nil), "docker.swarmkit.v1.Meta")
|
|
proto.RegisterType((*Node)(nil), "docker.swarmkit.v1.Node")
|
|
proto.RegisterType((*Service)(nil), "docker.swarmkit.v1.Service")
|
|
proto.RegisterType((*Endpoint)(nil), "docker.swarmkit.v1.Endpoint")
|
|
proto.RegisterType((*Endpoint_VirtualIP)(nil), "docker.swarmkit.v1.Endpoint.VirtualIP")
|
|
proto.RegisterType((*Task)(nil), "docker.swarmkit.v1.Task")
|
|
proto.RegisterType((*NetworkAttachment)(nil), "docker.swarmkit.v1.NetworkAttachment")
|
|
proto.RegisterType((*Network)(nil), "docker.swarmkit.v1.Network")
|
|
proto.RegisterType((*Cluster)(nil), "docker.swarmkit.v1.Cluster")
|
|
proto.RegisterType((*Secret)(nil), "docker.swarmkit.v1.Secret")
|
|
}
|
|
|
|
func (m *Meta) Copy() *Meta {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Meta{
|
|
Version: *m.Version.Copy(),
|
|
CreatedAt: m.CreatedAt.Copy(),
|
|
UpdatedAt: m.UpdatedAt.Copy(),
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Node) Copy() *Node {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Node{
|
|
ID: m.ID,
|
|
Meta: *m.Meta.Copy(),
|
|
Spec: *m.Spec.Copy(),
|
|
Description: m.Description.Copy(),
|
|
Status: *m.Status.Copy(),
|
|
ManagerStatus: m.ManagerStatus.Copy(),
|
|
Attachment: m.Attachment.Copy(),
|
|
Certificate: *m.Certificate.Copy(),
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Service) Copy() *Service {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Service{
|
|
ID: m.ID,
|
|
Meta: *m.Meta.Copy(),
|
|
Spec: *m.Spec.Copy(),
|
|
PreviousSpec: m.PreviousSpec.Copy(),
|
|
Endpoint: m.Endpoint.Copy(),
|
|
UpdateStatus: m.UpdateStatus.Copy(),
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Endpoint) Copy() *Endpoint {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Endpoint{
|
|
Spec: m.Spec.Copy(),
|
|
}
|
|
|
|
if m.Ports != nil {
|
|
o.Ports = make([]*PortConfig, 0, len(m.Ports))
|
|
for _, v := range m.Ports {
|
|
o.Ports = append(o.Ports, v.Copy())
|
|
}
|
|
}
|
|
|
|
if m.VirtualIPs != nil {
|
|
o.VirtualIPs = make([]*Endpoint_VirtualIP, 0, len(m.VirtualIPs))
|
|
for _, v := range m.VirtualIPs {
|
|
o.VirtualIPs = append(o.VirtualIPs, v.Copy())
|
|
}
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Copy() *Endpoint_VirtualIP {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Endpoint_VirtualIP{
|
|
NetworkID: m.NetworkID,
|
|
Addr: m.Addr,
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Task) Copy() *Task {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Task{
|
|
ID: m.ID,
|
|
Meta: *m.Meta.Copy(),
|
|
Spec: *m.Spec.Copy(),
|
|
ServiceID: m.ServiceID,
|
|
Slot: m.Slot,
|
|
NodeID: m.NodeID,
|
|
Annotations: *m.Annotations.Copy(),
|
|
ServiceAnnotations: *m.ServiceAnnotations.Copy(),
|
|
Status: *m.Status.Copy(),
|
|
DesiredState: m.DesiredState,
|
|
Endpoint: m.Endpoint.Copy(),
|
|
LogDriver: m.LogDriver.Copy(),
|
|
}
|
|
|
|
if m.Networks != nil {
|
|
o.Networks = make([]*NetworkAttachment, 0, len(m.Networks))
|
|
for _, v := range m.Networks {
|
|
o.Networks = append(o.Networks, v.Copy())
|
|
}
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *NetworkAttachment) Copy() *NetworkAttachment {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &NetworkAttachment{
|
|
Network: m.Network.Copy(),
|
|
}
|
|
|
|
if m.Addresses != nil {
|
|
o.Addresses = make([]string, 0, len(m.Addresses))
|
|
o.Addresses = append(o.Addresses, m.Addresses...)
|
|
}
|
|
|
|
if m.Aliases != nil {
|
|
o.Aliases = make([]string, 0, len(m.Aliases))
|
|
o.Aliases = append(o.Aliases, m.Aliases...)
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Network) Copy() *Network {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Network{
|
|
ID: m.ID,
|
|
Meta: *m.Meta.Copy(),
|
|
Spec: *m.Spec.Copy(),
|
|
DriverState: m.DriverState.Copy(),
|
|
IPAM: m.IPAM.Copy(),
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Cluster) Copy() *Cluster {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Cluster{
|
|
ID: m.ID,
|
|
Meta: *m.Meta.Copy(),
|
|
Spec: *m.Spec.Copy(),
|
|
RootCA: *m.RootCA.Copy(),
|
|
EncryptionKeyLamportClock: m.EncryptionKeyLamportClock,
|
|
}
|
|
|
|
if m.NetworkBootstrapKeys != nil {
|
|
o.NetworkBootstrapKeys = make([]*EncryptionKey, 0, len(m.NetworkBootstrapKeys))
|
|
for _, v := range m.NetworkBootstrapKeys {
|
|
o.NetworkBootstrapKeys = append(o.NetworkBootstrapKeys, v.Copy())
|
|
}
|
|
}
|
|
|
|
if m.BlacklistedCertificates != nil {
|
|
o.BlacklistedCertificates = make(map[string]*BlacklistedCertificate)
|
|
for k, v := range m.BlacklistedCertificates {
|
|
o.BlacklistedCertificates[k] = v.Copy()
|
|
}
|
|
}
|
|
|
|
if m.UnlockKeys != nil {
|
|
o.UnlockKeys = make([]*EncryptionKey, 0, len(m.UnlockKeys))
|
|
for _, v := range m.UnlockKeys {
|
|
o.UnlockKeys = append(o.UnlockKeys, v.Copy())
|
|
}
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (m *Secret) Copy() *Secret {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
|
|
o := &Secret{
|
|
ID: m.ID,
|
|
Meta: *m.Meta.Copy(),
|
|
Spec: *m.Spec.Copy(),
|
|
Internal: m.Internal,
|
|
}
|
|
|
|
return o
|
|
}
|
|
|
|
func (this *Meta) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 7)
|
|
s = append(s, "&api.Meta{")
|
|
s = append(s, "Version: "+strings.Replace(this.Version.GoString(), `&`, ``, 1)+",\n")
|
|
if this.CreatedAt != nil {
|
|
s = append(s, "CreatedAt: "+fmt.Sprintf("%#v", this.CreatedAt)+",\n")
|
|
}
|
|
if this.UpdatedAt != nil {
|
|
s = append(s, "UpdatedAt: "+fmt.Sprintf("%#v", this.UpdatedAt)+",\n")
|
|
}
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Node) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 12)
|
|
s = append(s, "&api.Node{")
|
|
s = append(s, "ID: "+fmt.Sprintf("%#v", this.ID)+",\n")
|
|
s = append(s, "Meta: "+strings.Replace(this.Meta.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Spec: "+strings.Replace(this.Spec.GoString(), `&`, ``, 1)+",\n")
|
|
if this.Description != nil {
|
|
s = append(s, "Description: "+fmt.Sprintf("%#v", this.Description)+",\n")
|
|
}
|
|
s = append(s, "Status: "+strings.Replace(this.Status.GoString(), `&`, ``, 1)+",\n")
|
|
if this.ManagerStatus != nil {
|
|
s = append(s, "ManagerStatus: "+fmt.Sprintf("%#v", this.ManagerStatus)+",\n")
|
|
}
|
|
if this.Attachment != nil {
|
|
s = append(s, "Attachment: "+fmt.Sprintf("%#v", this.Attachment)+",\n")
|
|
}
|
|
s = append(s, "Certificate: "+strings.Replace(this.Certificate.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Service) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 10)
|
|
s = append(s, "&api.Service{")
|
|
s = append(s, "ID: "+fmt.Sprintf("%#v", this.ID)+",\n")
|
|
s = append(s, "Meta: "+strings.Replace(this.Meta.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Spec: "+strings.Replace(this.Spec.GoString(), `&`, ``, 1)+",\n")
|
|
if this.PreviousSpec != nil {
|
|
s = append(s, "PreviousSpec: "+fmt.Sprintf("%#v", this.PreviousSpec)+",\n")
|
|
}
|
|
if this.Endpoint != nil {
|
|
s = append(s, "Endpoint: "+fmt.Sprintf("%#v", this.Endpoint)+",\n")
|
|
}
|
|
if this.UpdateStatus != nil {
|
|
s = append(s, "UpdateStatus: "+fmt.Sprintf("%#v", this.UpdateStatus)+",\n")
|
|
}
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Endpoint) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 7)
|
|
s = append(s, "&api.Endpoint{")
|
|
if this.Spec != nil {
|
|
s = append(s, "Spec: "+fmt.Sprintf("%#v", this.Spec)+",\n")
|
|
}
|
|
if this.Ports != nil {
|
|
s = append(s, "Ports: "+fmt.Sprintf("%#v", this.Ports)+",\n")
|
|
}
|
|
if this.VirtualIPs != nil {
|
|
s = append(s, "VirtualIPs: "+fmt.Sprintf("%#v", this.VirtualIPs)+",\n")
|
|
}
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Endpoint_VirtualIP) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 6)
|
|
s = append(s, "&api.Endpoint_VirtualIP{")
|
|
s = append(s, "NetworkID: "+fmt.Sprintf("%#v", this.NetworkID)+",\n")
|
|
s = append(s, "Addr: "+fmt.Sprintf("%#v", this.Addr)+",\n")
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Task) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 17)
|
|
s = append(s, "&api.Task{")
|
|
s = append(s, "ID: "+fmt.Sprintf("%#v", this.ID)+",\n")
|
|
s = append(s, "Meta: "+strings.Replace(this.Meta.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Spec: "+strings.Replace(this.Spec.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "ServiceID: "+fmt.Sprintf("%#v", this.ServiceID)+",\n")
|
|
s = append(s, "Slot: "+fmt.Sprintf("%#v", this.Slot)+",\n")
|
|
s = append(s, "NodeID: "+fmt.Sprintf("%#v", this.NodeID)+",\n")
|
|
s = append(s, "Annotations: "+strings.Replace(this.Annotations.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "ServiceAnnotations: "+strings.Replace(this.ServiceAnnotations.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Status: "+strings.Replace(this.Status.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "DesiredState: "+fmt.Sprintf("%#v", this.DesiredState)+",\n")
|
|
if this.Networks != nil {
|
|
s = append(s, "Networks: "+fmt.Sprintf("%#v", this.Networks)+",\n")
|
|
}
|
|
if this.Endpoint != nil {
|
|
s = append(s, "Endpoint: "+fmt.Sprintf("%#v", this.Endpoint)+",\n")
|
|
}
|
|
if this.LogDriver != nil {
|
|
s = append(s, "LogDriver: "+fmt.Sprintf("%#v", this.LogDriver)+",\n")
|
|
}
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *NetworkAttachment) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 7)
|
|
s = append(s, "&api.NetworkAttachment{")
|
|
if this.Network != nil {
|
|
s = append(s, "Network: "+fmt.Sprintf("%#v", this.Network)+",\n")
|
|
}
|
|
s = append(s, "Addresses: "+fmt.Sprintf("%#v", this.Addresses)+",\n")
|
|
s = append(s, "Aliases: "+fmt.Sprintf("%#v", this.Aliases)+",\n")
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Network) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 9)
|
|
s = append(s, "&api.Network{")
|
|
s = append(s, "ID: "+fmt.Sprintf("%#v", this.ID)+",\n")
|
|
s = append(s, "Meta: "+strings.Replace(this.Meta.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Spec: "+strings.Replace(this.Spec.GoString(), `&`, ``, 1)+",\n")
|
|
if this.DriverState != nil {
|
|
s = append(s, "DriverState: "+fmt.Sprintf("%#v", this.DriverState)+",\n")
|
|
}
|
|
if this.IPAM != nil {
|
|
s = append(s, "IPAM: "+fmt.Sprintf("%#v", this.IPAM)+",\n")
|
|
}
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Cluster) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 12)
|
|
s = append(s, "&api.Cluster{")
|
|
s = append(s, "ID: "+fmt.Sprintf("%#v", this.ID)+",\n")
|
|
s = append(s, "Meta: "+strings.Replace(this.Meta.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Spec: "+strings.Replace(this.Spec.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "RootCA: "+strings.Replace(this.RootCA.GoString(), `&`, ``, 1)+",\n")
|
|
if this.NetworkBootstrapKeys != nil {
|
|
s = append(s, "NetworkBootstrapKeys: "+fmt.Sprintf("%#v", this.NetworkBootstrapKeys)+",\n")
|
|
}
|
|
s = append(s, "EncryptionKeyLamportClock: "+fmt.Sprintf("%#v", this.EncryptionKeyLamportClock)+",\n")
|
|
keysForBlacklistedCertificates := make([]string, 0, len(this.BlacklistedCertificates))
|
|
for k, _ := range this.BlacklistedCertificates {
|
|
keysForBlacklistedCertificates = append(keysForBlacklistedCertificates, k)
|
|
}
|
|
github_com_gogo_protobuf_sortkeys.Strings(keysForBlacklistedCertificates)
|
|
mapStringForBlacklistedCertificates := "map[string]*BlacklistedCertificate{"
|
|
for _, k := range keysForBlacklistedCertificates {
|
|
mapStringForBlacklistedCertificates += fmt.Sprintf("%#v: %#v,", k, this.BlacklistedCertificates[k])
|
|
}
|
|
mapStringForBlacklistedCertificates += "}"
|
|
if this.BlacklistedCertificates != nil {
|
|
s = append(s, "BlacklistedCertificates: "+mapStringForBlacklistedCertificates+",\n")
|
|
}
|
|
if this.UnlockKeys != nil {
|
|
s = append(s, "UnlockKeys: "+fmt.Sprintf("%#v", this.UnlockKeys)+",\n")
|
|
}
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func (this *Secret) GoString() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := make([]string, 0, 8)
|
|
s = append(s, "&api.Secret{")
|
|
s = append(s, "ID: "+fmt.Sprintf("%#v", this.ID)+",\n")
|
|
s = append(s, "Meta: "+strings.Replace(this.Meta.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Spec: "+strings.Replace(this.Spec.GoString(), `&`, ``, 1)+",\n")
|
|
s = append(s, "Internal: "+fmt.Sprintf("%#v", this.Internal)+",\n")
|
|
s = append(s, "}")
|
|
return strings.Join(s, "")
|
|
}
|
|
func valueToGoStringObjects(v interface{}, typ string) string {
|
|
rv := reflect.ValueOf(v)
|
|
if rv.IsNil() {
|
|
return "nil"
|
|
}
|
|
pv := reflect.Indirect(rv).Interface()
|
|
return fmt.Sprintf("func(v %v) *%v { return &v } ( %#v )", typ, typ, pv)
|
|
}
|
|
func extensionToGoStringObjects(m github_com_gogo_protobuf_proto.Message) string {
|
|
e := github_com_gogo_protobuf_proto.GetUnsafeExtensionsMap(m)
|
|
if e == nil {
|
|
return "nil"
|
|
}
|
|
s := "proto.NewUnsafeXXX_InternalExtensions(map[int32]proto.Extension{"
|
|
keys := make([]int, 0, len(e))
|
|
for k := range e {
|
|
keys = append(keys, int(k))
|
|
}
|
|
sort.Ints(keys)
|
|
ss := []string{}
|
|
for _, k := range keys {
|
|
ss = append(ss, strconv.Itoa(k)+": "+e[int32(k)].GoString())
|
|
}
|
|
s += strings.Join(ss, ",") + "})"
|
|
return s
|
|
}
|
|
func (m *Meta) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Meta) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Version.Size()))
|
|
n1, err := m.Version.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n1
|
|
if m.CreatedAt != nil {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.CreatedAt.Size()))
|
|
n2, err := m.CreatedAt.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n2
|
|
}
|
|
if m.UpdatedAt != nil {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.UpdatedAt.Size()))
|
|
n3, err := m.UpdatedAt.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n3
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Node) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Node) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ID)))
|
|
i += copy(data[i:], m.ID)
|
|
}
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Meta.Size()))
|
|
n4, err := m.Meta.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n4
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n5, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n5
|
|
if m.Description != nil {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Description.Size()))
|
|
n6, err := m.Description.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n6
|
|
}
|
|
data[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Status.Size()))
|
|
n7, err := m.Status.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n7
|
|
if m.ManagerStatus != nil {
|
|
data[i] = 0x32
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.ManagerStatus.Size()))
|
|
n8, err := m.ManagerStatus.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n8
|
|
}
|
|
if m.Attachment != nil {
|
|
data[i] = 0x3a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Attachment.Size()))
|
|
n9, err := m.Attachment.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n9
|
|
}
|
|
data[i] = 0x42
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Certificate.Size()))
|
|
n10, err := m.Certificate.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n10
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Service) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Service) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ID)))
|
|
i += copy(data[i:], m.ID)
|
|
}
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Meta.Size()))
|
|
n11, err := m.Meta.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n11
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n12, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n12
|
|
if m.Endpoint != nil {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Endpoint.Size()))
|
|
n13, err := m.Endpoint.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n13
|
|
}
|
|
if m.UpdateStatus != nil {
|
|
data[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.UpdateStatus.Size()))
|
|
n14, err := m.UpdateStatus.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n14
|
|
}
|
|
if m.PreviousSpec != nil {
|
|
data[i] = 0x32
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.PreviousSpec.Size()))
|
|
n15, err := m.PreviousSpec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n15
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Endpoint) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Endpoint) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.Spec != nil {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n16, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n16
|
|
}
|
|
if len(m.Ports) > 0 {
|
|
for _, msg := range m.Ports {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if len(m.VirtualIPs) > 0 {
|
|
for _, msg := range m.VirtualIPs {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.NetworkID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.NetworkID)))
|
|
i += copy(data[i:], m.NetworkID)
|
|
}
|
|
if len(m.Addr) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.Addr)))
|
|
i += copy(data[i:], m.Addr)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Task) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Task) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ID)))
|
|
i += copy(data[i:], m.ID)
|
|
}
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Meta.Size()))
|
|
n17, err := m.Meta.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n17
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n18, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n18
|
|
if len(m.ServiceID) > 0 {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ServiceID)))
|
|
i += copy(data[i:], m.ServiceID)
|
|
}
|
|
if m.Slot != 0 {
|
|
data[i] = 0x28
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Slot))
|
|
}
|
|
if len(m.NodeID) > 0 {
|
|
data[i] = 0x32
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.NodeID)))
|
|
i += copy(data[i:], m.NodeID)
|
|
}
|
|
data[i] = 0x3a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Annotations.Size()))
|
|
n19, err := m.Annotations.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n19
|
|
data[i] = 0x42
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.ServiceAnnotations.Size()))
|
|
n20, err := m.ServiceAnnotations.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n20
|
|
data[i] = 0x4a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Status.Size()))
|
|
n21, err := m.Status.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n21
|
|
if m.DesiredState != 0 {
|
|
data[i] = 0x50
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.DesiredState))
|
|
}
|
|
if len(m.Networks) > 0 {
|
|
for _, msg := range m.Networks {
|
|
data[i] = 0x5a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if m.Endpoint != nil {
|
|
data[i] = 0x62
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Endpoint.Size()))
|
|
n22, err := m.Endpoint.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n22
|
|
}
|
|
if m.LogDriver != nil {
|
|
data[i] = 0x6a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.LogDriver.Size()))
|
|
n23, err := m.LogDriver.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n23
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *NetworkAttachment) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *NetworkAttachment) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.Network != nil {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Network.Size()))
|
|
n24, err := m.Network.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n24
|
|
}
|
|
if len(m.Addresses) > 0 {
|
|
for _, s := range m.Addresses {
|
|
data[i] = 0x12
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
data[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
data[i] = uint8(l)
|
|
i++
|
|
i += copy(data[i:], s)
|
|
}
|
|
}
|
|
if len(m.Aliases) > 0 {
|
|
for _, s := range m.Aliases {
|
|
data[i] = 0x1a
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
data[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
data[i] = uint8(l)
|
|
i++
|
|
i += copy(data[i:], s)
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Network) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Network) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ID)))
|
|
i += copy(data[i:], m.ID)
|
|
}
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Meta.Size()))
|
|
n25, err := m.Meta.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n25
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n26, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n26
|
|
if m.DriverState != nil {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.DriverState.Size()))
|
|
n27, err := m.DriverState.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n27
|
|
}
|
|
if m.IPAM != nil {
|
|
data[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.IPAM.Size()))
|
|
n28, err := m.IPAM.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n28
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Cluster) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Cluster) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ID)))
|
|
i += copy(data[i:], m.ID)
|
|
}
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Meta.Size()))
|
|
n29, err := m.Meta.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n29
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n30, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n30
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.RootCA.Size()))
|
|
n31, err := m.RootCA.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n31
|
|
if len(m.NetworkBootstrapKeys) > 0 {
|
|
for _, msg := range m.NetworkBootstrapKeys {
|
|
data[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if m.EncryptionKeyLamportClock != 0 {
|
|
data[i] = 0x30
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.EncryptionKeyLamportClock))
|
|
}
|
|
if len(m.BlacklistedCertificates) > 0 {
|
|
for k, _ := range m.BlacklistedCertificates {
|
|
data[i] = 0x42
|
|
i++
|
|
v := m.BlacklistedCertificates[k]
|
|
msgSize := 0
|
|
if v != nil {
|
|
msgSize = v.Size()
|
|
msgSize += 1 + sovObjects(uint64(msgSize))
|
|
}
|
|
mapSize := 1 + len(k) + sovObjects(uint64(len(k))) + msgSize
|
|
i = encodeVarintObjects(data, i, uint64(mapSize))
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(k)))
|
|
i += copy(data[i:], k)
|
|
if v != nil {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(v.Size()))
|
|
n32, err := v.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n32
|
|
}
|
|
}
|
|
}
|
|
if len(m.UnlockKeys) > 0 {
|
|
for _, msg := range m.UnlockKeys {
|
|
data[i] = 0x4a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Secret) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Secret) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(len(m.ID)))
|
|
i += copy(data[i:], m.ID)
|
|
}
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Meta.Size()))
|
|
n33, err := m.Meta.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n33
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(data, i, uint64(m.Spec.Size()))
|
|
n34, err := m.Spec.MarshalTo(data[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n34
|
|
if m.Internal {
|
|
data[i] = 0x20
|
|
i++
|
|
if m.Internal {
|
|
data[i] = 1
|
|
} else {
|
|
data[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func encodeFixed64Objects(data []byte, offset int, v uint64) int {
|
|
data[offset] = uint8(v)
|
|
data[offset+1] = uint8(v >> 8)
|
|
data[offset+2] = uint8(v >> 16)
|
|
data[offset+3] = uint8(v >> 24)
|
|
data[offset+4] = uint8(v >> 32)
|
|
data[offset+5] = uint8(v >> 40)
|
|
data[offset+6] = uint8(v >> 48)
|
|
data[offset+7] = uint8(v >> 56)
|
|
return offset + 8
|
|
}
|
|
func encodeFixed32Objects(data []byte, offset int, v uint32) int {
|
|
data[offset] = uint8(v)
|
|
data[offset+1] = uint8(v >> 8)
|
|
data[offset+2] = uint8(v >> 16)
|
|
data[offset+3] = uint8(v >> 24)
|
|
return offset + 4
|
|
}
|
|
func encodeVarintObjects(data []byte, offset int, v uint64) int {
|
|
for v >= 1<<7 {
|
|
data[offset] = uint8(v&0x7f | 0x80)
|
|
v >>= 7
|
|
offset++
|
|
}
|
|
data[offset] = uint8(v)
|
|
return offset + 1
|
|
}
|
|
|
|
func (m *Meta) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = m.Version.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.CreatedAt != nil {
|
|
l = m.CreatedAt.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.UpdatedAt != nil {
|
|
l = m.UpdatedAt.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Node) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Description != nil {
|
|
l = m.Description.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Status.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.ManagerStatus != nil {
|
|
l = m.ManagerStatus.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.Attachment != nil {
|
|
l = m.Attachment.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Certificate.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
return n
|
|
}
|
|
|
|
func (m *Service) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Endpoint != nil {
|
|
l = m.Endpoint.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.UpdateStatus != nil {
|
|
l = m.UpdateStatus.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.PreviousSpec != nil {
|
|
l = m.PreviousSpec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Endpoint) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if m.Spec != nil {
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if len(m.Ports) > 0 {
|
|
for _, e := range m.Ports {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if len(m.VirtualIPs) > 0 {
|
|
for _, e := range m.VirtualIPs {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.NetworkID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = len(m.Addr)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Task) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = len(m.ServiceID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.Slot != 0 {
|
|
n += 1 + sovObjects(uint64(m.Slot))
|
|
}
|
|
l = len(m.NodeID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Annotations.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.ServiceAnnotations.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Status.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.DesiredState != 0 {
|
|
n += 1 + sovObjects(uint64(m.DesiredState))
|
|
}
|
|
if len(m.Networks) > 0 {
|
|
for _, e := range m.Networks {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if m.Endpoint != nil {
|
|
l = m.Endpoint.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.LogDriver != nil {
|
|
l = m.LogDriver.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *NetworkAttachment) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if m.Network != nil {
|
|
l = m.Network.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if len(m.Addresses) > 0 {
|
|
for _, s := range m.Addresses {
|
|
l = len(s)
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Aliases) > 0 {
|
|
for _, s := range m.Aliases {
|
|
l = len(s)
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Network) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.DriverState != nil {
|
|
l = m.DriverState.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.IPAM != nil {
|
|
l = m.IPAM.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Cluster) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.RootCA.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if len(m.NetworkBootstrapKeys) > 0 {
|
|
for _, e := range m.NetworkBootstrapKeys {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if m.EncryptionKeyLamportClock != 0 {
|
|
n += 1 + sovObjects(uint64(m.EncryptionKeyLamportClock))
|
|
}
|
|
if len(m.BlacklistedCertificates) > 0 {
|
|
for k, v := range m.BlacklistedCertificates {
|
|
_ = k
|
|
_ = v
|
|
l = 0
|
|
if v != nil {
|
|
l = v.Size()
|
|
l += 1 + sovObjects(uint64(l))
|
|
}
|
|
mapEntrySize := 1 + len(k) + sovObjects(uint64(len(k))) + l
|
|
n += mapEntrySize + 1 + sovObjects(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if len(m.UnlockKeys) > 0 {
|
|
for _, e := range m.UnlockKeys {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Secret) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Internal {
|
|
n += 2
|
|
}
|
|
return n
|
|
}
|
|
|
|
func sovObjects(x uint64) (n int) {
|
|
for {
|
|
n++
|
|
x >>= 7
|
|
if x == 0 {
|
|
break
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
func sozObjects(x uint64) (n int) {
|
|
return sovObjects(uint64((x << 1) ^ uint64((int64(x) >> 63))))
|
|
}
|
|
func (this *Meta) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Meta{`,
|
|
`Version:` + strings.Replace(strings.Replace(this.Version.String(), "Version", "Version", 1), `&`, ``, 1) + `,`,
|
|
`CreatedAt:` + strings.Replace(fmt.Sprintf("%v", this.CreatedAt), "Timestamp", "docker_swarmkit_v1.Timestamp", 1) + `,`,
|
|
`UpdatedAt:` + strings.Replace(fmt.Sprintf("%v", this.UpdatedAt), "Timestamp", "docker_swarmkit_v1.Timestamp", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Node) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Node{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "NodeSpec", "NodeSpec", 1), `&`, ``, 1) + `,`,
|
|
`Description:` + strings.Replace(fmt.Sprintf("%v", this.Description), "NodeDescription", "NodeDescription", 1) + `,`,
|
|
`Status:` + strings.Replace(strings.Replace(this.Status.String(), "NodeStatus", "NodeStatus", 1), `&`, ``, 1) + `,`,
|
|
`ManagerStatus:` + strings.Replace(fmt.Sprintf("%v", this.ManagerStatus), "ManagerStatus", "ManagerStatus", 1) + `,`,
|
|
`Attachment:` + strings.Replace(fmt.Sprintf("%v", this.Attachment), "NetworkAttachment", "NetworkAttachment", 1) + `,`,
|
|
`Certificate:` + strings.Replace(strings.Replace(this.Certificate.String(), "Certificate", "Certificate", 1), `&`, ``, 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Service) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Service{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "ServiceSpec", "ServiceSpec", 1), `&`, ``, 1) + `,`,
|
|
`Endpoint:` + strings.Replace(fmt.Sprintf("%v", this.Endpoint), "Endpoint", "Endpoint", 1) + `,`,
|
|
`UpdateStatus:` + strings.Replace(fmt.Sprintf("%v", this.UpdateStatus), "UpdateStatus", "UpdateStatus", 1) + `,`,
|
|
`PreviousSpec:` + strings.Replace(fmt.Sprintf("%v", this.PreviousSpec), "ServiceSpec", "ServiceSpec", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Endpoint) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Endpoint{`,
|
|
`Spec:` + strings.Replace(fmt.Sprintf("%v", this.Spec), "EndpointSpec", "EndpointSpec", 1) + `,`,
|
|
`Ports:` + strings.Replace(fmt.Sprintf("%v", this.Ports), "PortConfig", "PortConfig", 1) + `,`,
|
|
`VirtualIPs:` + strings.Replace(fmt.Sprintf("%v", this.VirtualIPs), "Endpoint_VirtualIP", "Endpoint_VirtualIP", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Endpoint_VirtualIP) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Endpoint_VirtualIP{`,
|
|
`NetworkID:` + fmt.Sprintf("%v", this.NetworkID) + `,`,
|
|
`Addr:` + fmt.Sprintf("%v", this.Addr) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Task) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Task{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "TaskSpec", "TaskSpec", 1), `&`, ``, 1) + `,`,
|
|
`ServiceID:` + fmt.Sprintf("%v", this.ServiceID) + `,`,
|
|
`Slot:` + fmt.Sprintf("%v", this.Slot) + `,`,
|
|
`NodeID:` + fmt.Sprintf("%v", this.NodeID) + `,`,
|
|
`Annotations:` + strings.Replace(strings.Replace(this.Annotations.String(), "Annotations", "Annotations", 1), `&`, ``, 1) + `,`,
|
|
`ServiceAnnotations:` + strings.Replace(strings.Replace(this.ServiceAnnotations.String(), "Annotations", "Annotations", 1), `&`, ``, 1) + `,`,
|
|
`Status:` + strings.Replace(strings.Replace(this.Status.String(), "TaskStatus", "TaskStatus", 1), `&`, ``, 1) + `,`,
|
|
`DesiredState:` + fmt.Sprintf("%v", this.DesiredState) + `,`,
|
|
`Networks:` + strings.Replace(fmt.Sprintf("%v", this.Networks), "NetworkAttachment", "NetworkAttachment", 1) + `,`,
|
|
`Endpoint:` + strings.Replace(fmt.Sprintf("%v", this.Endpoint), "Endpoint", "Endpoint", 1) + `,`,
|
|
`LogDriver:` + strings.Replace(fmt.Sprintf("%v", this.LogDriver), "Driver", "Driver", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *NetworkAttachment) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&NetworkAttachment{`,
|
|
`Network:` + strings.Replace(fmt.Sprintf("%v", this.Network), "Network", "Network", 1) + `,`,
|
|
`Addresses:` + fmt.Sprintf("%v", this.Addresses) + `,`,
|
|
`Aliases:` + fmt.Sprintf("%v", this.Aliases) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Network) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Network{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "NetworkSpec", "NetworkSpec", 1), `&`, ``, 1) + `,`,
|
|
`DriverState:` + strings.Replace(fmt.Sprintf("%v", this.DriverState), "Driver", "Driver", 1) + `,`,
|
|
`IPAM:` + strings.Replace(fmt.Sprintf("%v", this.IPAM), "IPAMOptions", "IPAMOptions", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Cluster) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
keysForBlacklistedCertificates := make([]string, 0, len(this.BlacklistedCertificates))
|
|
for k, _ := range this.BlacklistedCertificates {
|
|
keysForBlacklistedCertificates = append(keysForBlacklistedCertificates, k)
|
|
}
|
|
github_com_gogo_protobuf_sortkeys.Strings(keysForBlacklistedCertificates)
|
|
mapStringForBlacklistedCertificates := "map[string]*BlacklistedCertificate{"
|
|
for _, k := range keysForBlacklistedCertificates {
|
|
mapStringForBlacklistedCertificates += fmt.Sprintf("%v: %v,", k, this.BlacklistedCertificates[k])
|
|
}
|
|
mapStringForBlacklistedCertificates += "}"
|
|
s := strings.Join([]string{`&Cluster{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "ClusterSpec", "ClusterSpec", 1), `&`, ``, 1) + `,`,
|
|
`RootCA:` + strings.Replace(strings.Replace(this.RootCA.String(), "RootCA", "RootCA", 1), `&`, ``, 1) + `,`,
|
|
`NetworkBootstrapKeys:` + strings.Replace(fmt.Sprintf("%v", this.NetworkBootstrapKeys), "EncryptionKey", "EncryptionKey", 1) + `,`,
|
|
`EncryptionKeyLamportClock:` + fmt.Sprintf("%v", this.EncryptionKeyLamportClock) + `,`,
|
|
`BlacklistedCertificates:` + mapStringForBlacklistedCertificates + `,`,
|
|
`UnlockKeys:` + strings.Replace(fmt.Sprintf("%v", this.UnlockKeys), "EncryptionKey", "EncryptionKey", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Secret) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Secret{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "SecretSpec", "SecretSpec", 1), `&`, ``, 1) + `,`,
|
|
`Internal:` + fmt.Sprintf("%v", this.Internal) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func valueToStringObjects(v interface{}) string {
|
|
rv := reflect.ValueOf(v)
|
|
if rv.IsNil() {
|
|
return "nil"
|
|
}
|
|
pv := reflect.Indirect(rv).Interface()
|
|
return fmt.Sprintf("*%v", pv)
|
|
}
|
|
func (m *Meta) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Meta: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Meta: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Version", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Version.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CreatedAt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.CreatedAt == nil {
|
|
m.CreatedAt = &docker_swarmkit_v1.Timestamp{}
|
|
}
|
|
if err := m.CreatedAt.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UpdatedAt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.UpdatedAt == nil {
|
|
m.UpdatedAt = &docker_swarmkit_v1.Timestamp{}
|
|
}
|
|
if err := m.UpdatedAt.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Node) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Node: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Node: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Description", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Description == nil {
|
|
m.Description = &NodeDescription{}
|
|
}
|
|
if err := m.Description.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Status", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Status.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ManagerStatus", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.ManagerStatus == nil {
|
|
m.ManagerStatus = &ManagerStatus{}
|
|
}
|
|
if err := m.ManagerStatus.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attachment", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Attachment == nil {
|
|
m.Attachment = &NetworkAttachment{}
|
|
}
|
|
if err := m.Attachment.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Certificate", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Certificate.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Service) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Service: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Service: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Endpoint", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Endpoint == nil {
|
|
m.Endpoint = &Endpoint{}
|
|
}
|
|
if err := m.Endpoint.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UpdateStatus", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.UpdateStatus == nil {
|
|
m.UpdateStatus = &UpdateStatus{}
|
|
}
|
|
if err := m.UpdateStatus.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PreviousSpec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.PreviousSpec == nil {
|
|
m.PreviousSpec = &ServiceSpec{}
|
|
}
|
|
if err := m.PreviousSpec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Endpoint) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Endpoint: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Endpoint: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Spec == nil {
|
|
m.Spec = &EndpointSpec{}
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ports", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ports = append(m.Ports, &PortConfig{})
|
|
if err := m.Ports[len(m.Ports)-1].Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field VirtualIPs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.VirtualIPs = append(m.VirtualIPs, &Endpoint_VirtualIP{})
|
|
if err := m.VirtualIPs[len(m.VirtualIPs)-1].Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Endpoint_VirtualIP) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: VirtualIP: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: VirtualIP: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NetworkID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NetworkID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Addr", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Addr = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Task) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Task: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Task: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ServiceID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ServiceID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Slot", wireType)
|
|
}
|
|
m.Slot = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.Slot |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NodeID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NodeID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Annotations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Annotations.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ServiceAnnotations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.ServiceAnnotations.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Status", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Status.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DesiredState", wireType)
|
|
}
|
|
m.DesiredState = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.DesiredState |= (TaskState(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 11:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Networks", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Networks = append(m.Networks, &NetworkAttachment{})
|
|
if err := m.Networks[len(m.Networks)-1].Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 12:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Endpoint", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Endpoint == nil {
|
|
m.Endpoint = &Endpoint{}
|
|
}
|
|
if err := m.Endpoint.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 13:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field LogDriver", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.LogDriver == nil {
|
|
m.LogDriver = &Driver{}
|
|
}
|
|
if err := m.LogDriver.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *NetworkAttachment) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: NetworkAttachment: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: NetworkAttachment: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Network", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Network == nil {
|
|
m.Network = &Network{}
|
|
}
|
|
if err := m.Network.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Addresses", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Addresses = append(m.Addresses, string(data[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Aliases", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Aliases = append(m.Aliases, string(data[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Network) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Network: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Network: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DriverState", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.DriverState == nil {
|
|
m.DriverState = &Driver{}
|
|
}
|
|
if err := m.DriverState.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field IPAM", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.IPAM == nil {
|
|
m.IPAM = &IPAMOptions{}
|
|
}
|
|
if err := m.IPAM.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Cluster) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Cluster: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Cluster: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field RootCA", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.RootCA.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NetworkBootstrapKeys", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NetworkBootstrapKeys = append(m.NetworkBootstrapKeys, &EncryptionKey{})
|
|
if err := m.NetworkBootstrapKeys[len(m.NetworkBootstrapKeys)-1].Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field EncryptionKeyLamportClock", wireType)
|
|
}
|
|
m.EncryptionKeyLamportClock = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.EncryptionKeyLamportClock |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field BlacklistedCertificates", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
var keykey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
keykey |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey := string(data[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
if m.BlacklistedCertificates == nil {
|
|
m.BlacklistedCertificates = make(map[string]*BlacklistedCertificate)
|
|
}
|
|
if iNdEx < postIndex {
|
|
var valuekey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
valuekey |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
var mapmsglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
mapmsglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if mapmsglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postmsgIndex := iNdEx + mapmsglen
|
|
if mapmsglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if postmsgIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue := &BlacklistedCertificate{}
|
|
if err := mapvalue.Unmarshal(data[iNdEx:postmsgIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postmsgIndex
|
|
m.BlacklistedCertificates[mapkey] = mapvalue
|
|
} else {
|
|
var mapvalue *BlacklistedCertificate
|
|
m.BlacklistedCertificates[mapkey] = mapvalue
|
|
}
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UnlockKeys", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.UnlockKeys = append(m.UnlockKeys, &EncryptionKey{})
|
|
if err := m.UnlockKeys[len(m.UnlockKeys)-1].Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Secret) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Secret: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Secret: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(data[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Internal", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Internal = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func skipObjects(data []byte) (n int, err error) {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
wireType := int(wire & 0x7)
|
|
switch wireType {
|
|
case 0:
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx++
|
|
if data[iNdEx-1] < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
return iNdEx, nil
|
|
case 1:
|
|
iNdEx += 8
|
|
return iNdEx, nil
|
|
case 2:
|
|
var length int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
length |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
iNdEx += length
|
|
if length < 0 {
|
|
return 0, ErrInvalidLengthObjects
|
|
}
|
|
return iNdEx, nil
|
|
case 3:
|
|
for {
|
|
var innerWire uint64
|
|
var start int = iNdEx
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
innerWire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
innerWireType := int(innerWire & 0x7)
|
|
if innerWireType == 4 {
|
|
break
|
|
}
|
|
next, err := skipObjects(data[start:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
iNdEx = start + next
|
|
}
|
|
return iNdEx, nil
|
|
case 4:
|
|
return iNdEx, nil
|
|
case 5:
|
|
iNdEx += 4
|
|
return iNdEx, nil
|
|
default:
|
|
return 0, fmt.Errorf("proto: illegal wireType %d", wireType)
|
|
}
|
|
}
|
|
panic("unreachable")
|
|
}
|
|
|
|
var (
|
|
ErrInvalidLengthObjects = fmt.Errorf("proto: negative length found during unmarshaling")
|
|
ErrIntOverflowObjects = fmt.Errorf("proto: integer overflow")
|
|
)
|
|
|
|
func init() { proto.RegisterFile("objects.proto", fileDescriptorObjects) }
|
|
|
|
var fileDescriptorObjects = []byte{
|
|
// 1161 bytes of a gzipped FileDescriptorProto
|
|
0x1f, 0x8b, 0x08, 0x00, 0x00, 0x09, 0x6e, 0x88, 0x02, 0xff, 0xbc, 0x57, 0x4d, 0x8f, 0x1b, 0x35,
|
|
0x18, 0xee, 0x24, 0xb3, 0xf9, 0x78, 0xb3, 0x59, 0x81, 0xa9, 0xca, 0x34, 0x2c, 0xc9, 0x92, 0x0a,
|
|
0x54, 0xa1, 0x2a, 0x15, 0xa5, 0xa0, 0x2d, 0xb4, 0x82, 0x7c, 0x09, 0xa2, 0x52, 0xa8, 0xdc, 0xb2,
|
|
0x3d, 0x46, 0xde, 0x19, 0x37, 0x0c, 0x99, 0x8c, 0x47, 0xb6, 0x93, 0x2a, 0x37, 0xc4, 0x0f, 0xe0,
|
|
0x27, 0x20, 0xce, 0xfc, 0x09, 0xae, 0x7b, 0xe0, 0xc0, 0x0d, 0x4e, 0x11, 0x9b, 0x1b, 0x37, 0x7e,
|
|
0x02, 0xb2, 0xc7, 0x93, 0xcc, 0x2a, 0x93, 0x65, 0x2b, 0x55, 0x7b, 0xb3, 0xe3, 0xe7, 0x79, 0xde,
|
|
0xd7, 0xaf, 0x1f, 0xbf, 0xe3, 0x40, 0x95, 0x1d, 0x7f, 0x4f, 0x5d, 0x29, 0x5a, 0x11, 0x67, 0x92,
|
|
0x21, 0xe4, 0x31, 0x77, 0x4c, 0x79, 0x4b, 0xbc, 0x20, 0x7c, 0x32, 0xf6, 0x65, 0x6b, 0xf6, 0x41,
|
|
0xad, 0x22, 0xe7, 0x11, 0x35, 0x80, 0x5a, 0x45, 0x44, 0xd4, 0x4d, 0x26, 0xd7, 0xa5, 0x3f, 0xa1,
|
|
0x42, 0x92, 0x49, 0x74, 0x7b, 0x35, 0x32, 0x4b, 0x57, 0x47, 0x6c, 0xc4, 0xf4, 0xf0, 0xb6, 0x1a,
|
|
0xc5, 0xbf, 0x36, 0x7f, 0xb3, 0xc0, 0x7e, 0x44, 0x25, 0x41, 0x9f, 0x42, 0x71, 0x46, 0xb9, 0xf0,
|
|
0x59, 0xe8, 0x58, 0x07, 0xd6, 0xcd, 0xca, 0x9d, 0xb7, 0x5a, 0x9b, 0x91, 0x5b, 0x47, 0x31, 0xa4,
|
|
0x63, 0x9f, 0x2c, 0x1a, 0x57, 0x70, 0xc2, 0x40, 0xf7, 0x01, 0x5c, 0x4e, 0x89, 0xa4, 0xde, 0x90,
|
|
0x48, 0x27, 0xa7, 0xf9, 0x6f, 0x67, 0xf1, 0x9f, 0x26, 0x49, 0xe1, 0xb2, 0x21, 0xb4, 0xa5, 0x62,
|
|
0x4f, 0x23, 0x2f, 0x61, 0xe7, 0x2f, 0xc4, 0x36, 0x84, 0xb6, 0x6c, 0xfe, 0x93, 0x07, 0xfb, 0x6b,
|
|
0xe6, 0x51, 0x74, 0x0d, 0x72, 0xbe, 0xa7, 0x93, 0x2f, 0x77, 0x0a, 0xcb, 0x45, 0x23, 0x37, 0xe8,
|
|
0xe1, 0x9c, 0xef, 0xa1, 0x3b, 0x60, 0x4f, 0xa8, 0x24, 0x26, 0x2d, 0x27, 0x4b, 0x58, 0x55, 0xc0,
|
|
0xec, 0x49, 0x63, 0xd1, 0xc7, 0x60, 0xab, 0xb2, 0x9a, 0x64, 0xf6, 0xb3, 0x38, 0x2a, 0xe6, 0x93,
|
|
0x88, 0xba, 0x09, 0x4f, 0xe1, 0x51, 0x1f, 0x2a, 0x1e, 0x15, 0x2e, 0xf7, 0x23, 0xa9, 0x2a, 0x69,
|
|
0x6b, 0xfa, 0x8d, 0x6d, 0xf4, 0xde, 0x1a, 0x8a, 0xd3, 0x3c, 0x74, 0x1f, 0x0a, 0x42, 0x12, 0x39,
|
|
0x15, 0xce, 0x8e, 0x56, 0xa8, 0x6f, 0x4d, 0x40, 0xa3, 0x4c, 0x0a, 0x86, 0x83, 0xbe, 0x84, 0xbd,
|
|
0x09, 0x09, 0xc9, 0x88, 0xf2, 0xa1, 0x51, 0x29, 0x68, 0x95, 0x77, 0x32, 0xb7, 0x1e, 0x23, 0x63,
|
|
0x21, 0x5c, 0x9d, 0xa4, 0xa7, 0xa8, 0x0f, 0x40, 0xa4, 0x24, 0xee, 0x77, 0x13, 0x1a, 0x4a, 0xa7,
|
|
0xa8, 0x55, 0xde, 0xcd, 0xcc, 0x85, 0xca, 0x17, 0x8c, 0x8f, 0xdb, 0x2b, 0x30, 0x4e, 0x11, 0xd1,
|
|
0x17, 0x50, 0x71, 0x29, 0x97, 0xfe, 0x73, 0xdf, 0x25, 0x92, 0x3a, 0x25, 0xad, 0xd3, 0xc8, 0xd2,
|
|
0xe9, 0xae, 0x61, 0x66, 0x53, 0x69, 0x66, 0xf3, 0xcf, 0x1c, 0x14, 0x9f, 0x50, 0x3e, 0xf3, 0xdd,
|
|
0x57, 0x7b, 0xdc, 0xf7, 0xce, 0x1c, 0x77, 0x66, 0x66, 0x26, 0xec, 0xc6, 0x89, 0x1f, 0x42, 0x89,
|
|
0x86, 0x5e, 0xc4, 0xfc, 0x50, 0x9a, 0xe3, 0xce, 0x74, 0x4b, 0xdf, 0x60, 0xf0, 0x0a, 0x8d, 0xfa,
|
|
0x50, 0x8d, 0x5d, 0x3c, 0x3c, 0x73, 0xd6, 0x07, 0x59, 0xf4, 0x6f, 0x35, 0xd0, 0x1c, 0xd2, 0xee,
|
|
0x34, 0x35, 0x43, 0x3d, 0xa8, 0x46, 0x9c, 0xce, 0x7c, 0x36, 0x15, 0x43, 0xbd, 0x89, 0xc2, 0x85,
|
|
0x36, 0x81, 0x77, 0x13, 0x96, 0x9a, 0x35, 0x7f, 0xce, 0x41, 0x29, 0xc9, 0x11, 0xdd, 0x35, 0xe5,
|
|
0xb0, 0xb6, 0x27, 0x94, 0x60, 0xb5, 0x54, 0x5c, 0x89, 0xbb, 0xb0, 0x13, 0x31, 0x2e, 0x85, 0x93,
|
|
0x3b, 0xc8, 0x6f, 0xf3, 0xec, 0x63, 0xc6, 0x65, 0x97, 0x85, 0xcf, 0xfd, 0x11, 0x8e, 0xc1, 0xe8,
|
|
0x19, 0x54, 0x66, 0x3e, 0x97, 0x53, 0x12, 0x0c, 0xfd, 0x48, 0x38, 0x79, 0xcd, 0x7d, 0xef, 0xbc,
|
|
0x90, 0xad, 0xa3, 0x18, 0x3f, 0x78, 0xdc, 0xd9, 0x5b, 0x2e, 0x1a, 0xb0, 0x9a, 0x0a, 0x0c, 0x46,
|
|
0x6a, 0x10, 0x89, 0xda, 0x23, 0x28, 0xaf, 0x56, 0xd0, 0x2d, 0x80, 0x30, 0xb6, 0xe8, 0x70, 0x65,
|
|
0x9a, 0xea, 0x72, 0xd1, 0x28, 0x1b, 0xe3, 0x0e, 0x7a, 0xb8, 0x6c, 0x00, 0x03, 0x0f, 0x21, 0xb0,
|
|
0x89, 0xe7, 0x71, 0x6d, 0xa1, 0x32, 0xd6, 0xe3, 0xe6, 0xef, 0x3b, 0x60, 0x3f, 0x25, 0x62, 0x7c,
|
|
0xd9, 0x6d, 0x46, 0xc5, 0xdc, 0x30, 0xdd, 0x2d, 0x00, 0x11, 0x1f, 0xa5, 0xda, 0x8e, 0xbd, 0xde,
|
|
0x8e, 0x39, 0x60, 0xb5, 0x1d, 0x03, 0x88, 0xb7, 0x23, 0x02, 0x26, 0xb5, 0xbf, 0x6c, 0xac, 0xc7,
|
|
0xe8, 0x06, 0x14, 0x43, 0xe6, 0x69, 0x7a, 0x41, 0xd3, 0x61, 0xb9, 0x68, 0x14, 0x54, 0x4b, 0x19,
|
|
0xf4, 0x70, 0x41, 0x2d, 0x0d, 0x3c, 0x75, 0x6f, 0x49, 0x18, 0x32, 0x49, 0x54, 0x53, 0x12, 0xe6,
|
|
0xfe, 0x67, 0x1a, 0xab, 0xbd, 0x86, 0x25, 0xf7, 0x36, 0xc5, 0x44, 0x47, 0xf0, 0x46, 0x92, 0x6f,
|
|
0x5a, 0xb0, 0xf4, 0x32, 0x82, 0xc8, 0x28, 0xa4, 0x56, 0x52, 0x7d, 0xb2, 0xbc, 0xbd, 0x4f, 0xea,
|
|
0x0a, 0x66, 0xf5, 0xc9, 0x0e, 0x54, 0x3d, 0x2a, 0x7c, 0x4e, 0x3d, 0x7d, 0x03, 0xa9, 0x03, 0x07,
|
|
0xd6, 0xcd, 0xbd, 0x2d, 0x9f, 0x1e, 0x23, 0x42, 0xf1, 0xae, 0xe1, 0xe8, 0x19, 0x6a, 0x43, 0xc9,
|
|
0xf8, 0x46, 0x38, 0x15, 0xed, 0xdd, 0x0b, 0xf6, 0xc7, 0x15, 0xed, 0x4c, 0x07, 0xd9, 0x7d, 0xa9,
|
|
0x0e, 0x72, 0x0f, 0x20, 0x60, 0xa3, 0xa1, 0xc7, 0xfd, 0x19, 0xe5, 0x4e, 0x55, 0x73, 0x6b, 0x59,
|
|
0xdc, 0x9e, 0x46, 0xe0, 0x72, 0xc0, 0x46, 0xf1, 0xb0, 0xf9, 0xa3, 0x05, 0xaf, 0x6f, 0x24, 0x85,
|
|
0x3e, 0x82, 0xa2, 0x49, 0xeb, 0xbc, 0x47, 0x80, 0xe1, 0xe1, 0x04, 0x8b, 0xf6, 0xa1, 0xac, 0xee,
|
|
0x08, 0x15, 0x82, 0xc6, 0xb7, 0xbf, 0x8c, 0xd7, 0x3f, 0x20, 0x07, 0x8a, 0x24, 0xf0, 0x89, 0x5a,
|
|
0xcb, 0xeb, 0xb5, 0x64, 0xda, 0xfc, 0x29, 0x07, 0x45, 0x23, 0x76, 0xd9, 0xed, 0xdc, 0x84, 0xdd,
|
|
0xb8, 0x59, 0x0f, 0x60, 0x37, 0x2e, 0xa7, 0xb1, 0x84, 0xfd, 0xbf, 0x45, 0xad, 0xc4, 0xf8, 0xd8,
|
|
0x0e, 0x0f, 0xc0, 0xf6, 0x23, 0x32, 0x31, 0xad, 0x3c, 0x33, 0xf2, 0xe0, 0x71, 0xfb, 0xd1, 0x37,
|
|
0x51, 0xec, 0xec, 0xd2, 0x72, 0xd1, 0xb0, 0xd5, 0x0f, 0x58, 0xd3, 0x9a, 0xbf, 0xec, 0x40, 0xb1,
|
|
0x1b, 0x4c, 0x85, 0xa4, 0xfc, 0xb2, 0x0b, 0x62, 0xc2, 0x6e, 0x14, 0xa4, 0x0b, 0x45, 0xce, 0x98,
|
|
0x1c, 0xba, 0xe4, 0xbc, 0x5a, 0x60, 0xc6, 0x64, 0xb7, 0xdd, 0xd9, 0x53, 0x44, 0xd5, 0x48, 0xe2,
|
|
0x39, 0x2e, 0x28, 0x6a, 0x97, 0xa0, 0x67, 0x70, 0x2d, 0x69, 0xbf, 0xc7, 0x8c, 0x49, 0x21, 0x39,
|
|
0x89, 0x86, 0x63, 0x3a, 0x57, 0xdf, 0xbc, 0xfc, 0xb6, 0x97, 0x49, 0x3f, 0x74, 0xf9, 0x5c, 0x17,
|
|
0xea, 0x21, 0x9d, 0xe3, 0xab, 0x46, 0xa0, 0x93, 0xf0, 0x1f, 0xd2, 0xb9, 0x40, 0x9f, 0xc1, 0x3e,
|
|
0x5d, 0xc1, 0x94, 0xe2, 0x30, 0x20, 0x13, 0xf5, 0x61, 0x19, 0xba, 0x01, 0x73, 0xc7, 0xba, 0xb7,
|
|
0xd9, 0xf8, 0x3a, 0x4d, 0x4b, 0x7d, 0x15, 0x23, 0xba, 0x0a, 0x80, 0x04, 0x38, 0xc7, 0x01, 0x71,
|
|
0xc7, 0x81, 0x2f, 0xd4, 0xfb, 0x33, 0xf5, 0xd8, 0x50, 0xed, 0x49, 0xe5, 0x76, 0x78, 0x4e, 0xb5,
|
|
0x5a, 0x9d, 0x35, 0x37, 0xf5, 0x74, 0x11, 0xfd, 0x50, 0xf2, 0x39, 0x7e, 0xf3, 0x38, 0x7b, 0x15,
|
|
0x75, 0xa0, 0x32, 0x0d, 0x55, 0xf8, 0xb8, 0x06, 0xe5, 0x8b, 0xd6, 0x00, 0x62, 0x96, 0xda, 0x79,
|
|
0x6d, 0x06, 0xfb, 0xe7, 0x05, 0x47, 0xaf, 0x41, 0x7e, 0x4c, 0xe7, 0xb1, 0x7f, 0xb0, 0x1a, 0xa2,
|
|
0xcf, 0x61, 0x67, 0x46, 0x82, 0x29, 0x35, 0xce, 0x79, 0x3f, 0x2b, 0x5e, 0xb6, 0x24, 0x8e, 0x89,
|
|
0x9f, 0xe4, 0x0e, 0xad, 0xe6, 0xaf, 0x16, 0x14, 0x9e, 0x50, 0x97, 0x53, 0xf9, 0x4a, 0x1d, 0x7a,
|
|
0x78, 0xc6, 0xa1, 0xf5, 0xec, 0xc7, 0x8b, 0x8a, 0xba, 0x61, 0xd0, 0x1a, 0x94, 0xfc, 0x50, 0x52,
|
|
0x1e, 0x92, 0x40, 0x3b, 0xb4, 0x84, 0x57, 0xf3, 0xce, 0xfe, 0xc9, 0x69, 0xfd, 0xca, 0x5f, 0xa7,
|
|
0xf5, 0x2b, 0xff, 0x9e, 0xd6, 0xad, 0x1f, 0x96, 0x75, 0xeb, 0x64, 0x59, 0xb7, 0xfe, 0x58, 0xd6,
|
|
0xad, 0xbf, 0x97, 0x75, 0xeb, 0xb8, 0xa0, 0xff, 0x02, 0x7d, 0xf8, 0x5f, 0x00, 0x00, 0x00, 0xff,
|
|
0xff, 0x38, 0xf8, 0x23, 0xac, 0x72, 0x0d, 0x00, 0x00,
|
|
}
|